在Python中,转为Unicode字符串可以通过以下方法实现:使用str.encode()
方法、使用unicode_literals
导入、使用chr()
和ord()
函数。其中,使用str.encode()
方法是最常见的方式,这种方法可以将Python字符串编码为字节,然后再使用decode()
方法将其转换为Unicode字符串。详细介绍如下:
一、使用str.encode()
方法
在Python中,字符串默认是Unicode字符串,但有时需要将其转为特定编码的Unicode字符串。这时可以使用str.encode()
方法将字符串转换为字节串,然后再使用decode()
方法将其转为Unicode字符串。
示例:
# Python 3.x
original_string = "Hello, 世界"
将字符串编码为UTF-8字节串
byte_string = original_string.encode('utf-8')
将字节串解码为Unicode字符串
unicode_string = byte_string.decode('utf-8')
print(unicode_string) # 输出: Hello, 世界
在上面的示例中,original_string.encode('utf-8')
将字符串编码为UTF-8字节串,而byte_string.decode('utf-8')
则将其解码为Unicode字符串。这种方法确保了字符串在不同编码之间的转换。
二、使用unicode_literals
导入
在Python 2中,默认的字符串不是Unicode字符串,因此需要通过from __future__ import unicode_literals
来自动将所有字符串字面量视为Unicode字符串。
示例:
# Python 2.x
from __future__ import unicode_literals
original_string = "Hello, 世界"
print(type(original_string)) # 输出: <type 'unicode'>
通过导入unicode_literals
,Python 2中的所有字符串字面量都被视为Unicode字符串,这使得处理Unicode字符串变得更为简单。
三、使用chr()
和ord()
函数
在处理Unicode字符时,chr()
和ord()
函数也非常有用。chr()
函数返回一个字符对应的Unicode字符串,而ord()
函数返回字符的Unicode码点。
示例:
# Python 3.x
unicode_char = chr(20320) # 返回字符 '你'
unicode_code_point = ord('你') # 返回 20320
print(unicode_char) # 输出: 你
print(unicode_code_point) # 输出: 20320
通过这些函数,可以方便地在字符和其Unicode码点之间进行转换,这在处理特定Unicode字符时非常有用。
四、总结
在Python中,转为Unicode字符串的方法有多种选择,具体使用哪种方法取决于使用的Python版本和具体需求。在Python 3中,字符串默认是Unicode字符串,而在Python 2中需要特别处理。无论是通过str.encode()
方法、unicode_literals
导入,还是使用chr()
和ord()
函数,都可以有效地实现Unicode字符串的转换和处理。理解这些方法并灵活运用,可以帮助你在Python编程中更好地处理Unicode字符串。
相关问答FAQs:
如何在Python中将字符串转换为Unicode?
在Python中,所有字符串都是Unicode字符串。如果你想将一个普通字符串转换为Unicode格式,可以使用str.encode()
方法。这将返回一个字节串。例如,my_string = "hello"
,使用unicode_string = my_string.encode('utf-8')
可以将其转换为UTF-8编码的字节串。需要注意的是,在Python 3中,字符串默认就是Unicode。
Unicode编码有什么常见的用途?
Unicode编码在处理不同语言和符号时非常重要。它可以确保文本在不同平台和应用程序之间的一致性,特别是在涉及多种语言的情况下。开发者通常使用Unicode来保证字符的正确显示,避免出现乱码问题。在Web开发中,Unicode也使得网站能够支持全球用户。
如何查看Python中字符串的Unicode编码?
可以使用ord()
函数来获取单个字符的Unicode码点。例如,对于字符串中的字符,可以通过ord('A')
获得其Unicode码点。对于整个字符串,可以遍历每个字符并打印其Unicode值,例如:[ord(char) for char in my_string]
。这种方式可以帮助开发者了解字符在Unicode标准中的表现。
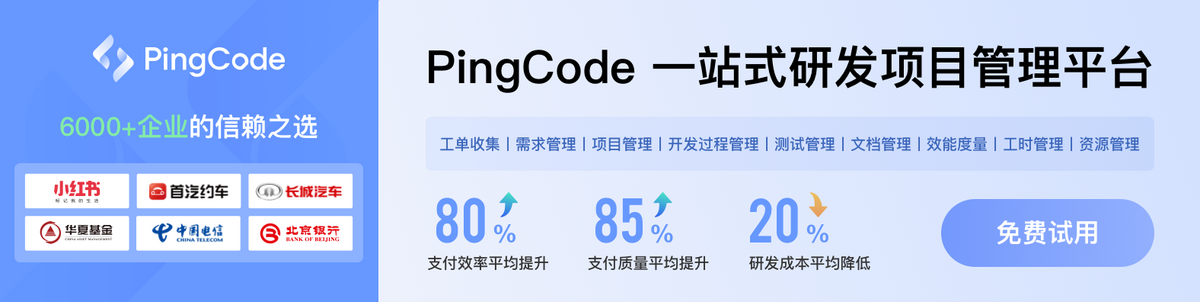