Python 调用接口的方法包括使用标准库模块、第三方库,以及手动构建 HTTP 请求等,常用的方法有 requests 库、http.client 模块、以及 urllib 库等。其中,requests 库因其简洁易用而被广泛推荐。 requests 库提供了简单的接口调用方式,可以通过几行代码发送 HTTP 请求并处理响应。使用 requests 库时,首先需要安装该库,然后通过指定 URL、请求方法(如 GET、POST)、请求头和请求体等参数来发起请求。以下是如何使用 requests 库来调接口的详细解释。
一、PYTHON 调用接口的常用方法
Python 提供了多种方法来调用 HTTP 接口,以下是一些常见的方式:
- 使用 requests 库
requests 是 Python 中最流行的 HTTP 库之一,它提供了简单而优雅的 API 来发送 HTTP 请求。使用 requests 库,你可以轻松地发送 GET、POST、PUT、DELETE 等请求。以下是一个简单的示例:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code)
print(response.json())
在这个示例中,我们使用 requests.get()
方法发送了一个 GET 请求,并打印了响应的状态码和 JSON 数据。requests 库还支持其他 HTTP 方法,如 requests.post()
、requests.put()
和 requests.delete()
,它们的用法与 requests.get()
类似。
- 使用 urllib 库
urllib 是 Python 的标准库,提供了一组用于处理 URL 的模块。虽然 urllib 的 API 相对复杂,但它也是调用接口的一个选择。以下是一个使用 urllib 发送 GET 请求的示例:
import urllib.request
import json
with urllib.request.urlopen('https://api.example.com/data') as response:
data = json.loads(response.read().decode())
print(data)
在这个示例中,我们使用 urllib.request.urlopen()
方法打开一个 URL,并读取响应数据。然后,我们使用 json.loads()
将响应数据解析为 Python 对象。
- 使用 http.client 模块
http.client 是 Python 的另一个标准库模块,提供了底层的 HTTP 协议处理功能。虽然使用起来稍微复杂一些,但它可以在需要更多控制的情况下使用。以下是一个简单的示例:
import http.client
import json
conn = http.client.HTTPSConnection('api.example.com')
conn.request('GET', '/data')
response = conn.getresponse()
data = json.loads(response.read().decode())
print(data)
conn.close()
在这个示例中,我们创建了一个 HTTPS 连接,并发送了一个 GET 请求。然后,我们读取响应数据并解析为 Python 对象。
二、使用 requests 库的高级功能
requests 库不仅提供了简单的接口调用功能,还支持许多高级功能,如会话管理、请求参数、请求头、认证、超时和错误处理等。
- 会话管理
requests 库提供了 Session
对象,用于跨请求保持会话信息。使用 Session
对象可以在多个请求之间共享 cookies 和其他会话数据。例如:
import requests
session = requests.Session()
session.get('https://example.com/login')
response = session.get('https://example.com/data')
print(response.json())
在这个示例中,我们首先创建了一个 Session
对象,并使用它发送了两个请求。第二个请求将共享第一个请求的会话信息。
- 请求参数
在发送请求时,可以通过 params
参数指定查询参数。例如:
import requests
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get('https://api.example.com/data', params=params)
print(response.url)
在这个示例中,我们通过 params
参数指定了查询参数,requests 库会自动将这些参数附加到 URL 中。
- 请求头
在发送请求时,可以通过 headers
参数指定请求头。例如:
import requests
headers = {'User-Agent': 'my-app/0.0.1'}
response = requests.get('https://api.example.com/data', headers=headers)
print(response.status_code)
在这个示例中,我们通过 headers
参数指定了自定义的请求头。
- 认证
requests 库支持多种认证方式,包括基本认证和 OAuth。以下是一个使用基本认证的示例:
import requests
from requests.auth import HTTPBasicAuth
response = requests.get('https://api.example.com/data', auth=HTTPBasicAuth('username', 'password'))
print(response.status_code)
在这个示例中,我们使用 HTTPBasicAuth
类指定了基本认证的用户名和密码。
- 超时和错误处理
requests 库允许通过 timeout
参数指定请求的超时时间,并支持异常处理。例如:
import requests
try:
response = requests.get('https://api.example.com/data', timeout=5)
response.raise_for_status()
except requests.exceptions.Timeout:
print('Request timed out')
except requests.exceptions.HTTPError as err:
print(f'HTTP error occurred: {err}')
except requests.exceptions.RequestException as err:
print(f'Request exception occurred: {err}')
else:
print(response.json())
在这个示例中,我们通过 timeout
参数指定了请求的超时时间,并使用 raise_for_status()
方法检查 HTTP 错误。我们还通过异常处理捕获并处理了请求中的错误。
三、使用 urllib 库的高级功能
urllib 库提供了一些高级功能,如自定义请求头、处理重定向、代理支持和认证等。
- 自定义请求头
在发送请求时,可以使用 urllib.request.Request
对象自定义请求头。例如:
import urllib.request
url = 'https://api.example.com/data'
request = urllib.request.Request(url, headers={'User-Agent': 'my-app/0.0.1'})
with urllib.request.urlopen(request) as response:
data = response.read().decode()
print(data)
在这个示例中,我们创建了一个 Request
对象,并通过 headers
参数指定了自定义的请求头。
- 处理重定向
urllib 库会自动处理 HTTP 重定向,但可以通过 HTTPRedirectHandler
类自定义重定向行为。例如:
import urllib.request
class MyRedirectHandler(urllib.request.HTTPRedirectHandler):
def redirect_request(self, req, fp, code, msg, headers, newurl):
print(f'Redirected to: {newurl}')
return super().redirect_request(req, fp, code, msg, headers, newurl)
opener = urllib.request.build_opener(MyRedirectHandler)
with opener.open('http://example.com') as response:
data = response.read().decode()
print(data)
在这个示例中,我们创建了一个自定义的 HTTPRedirectHandler
类,并在重定向时打印新 URL。
- 代理支持
urllib 库支持通过环境变量或 ProxyHandler
类配置代理。例如:
import urllib.request
proxy_handler = urllib.request.ProxyHandler({'http': 'http://proxy.example.com:8080'})
opener = urllib.request.build_opener(proxy_handler)
with opener.open('http://example.com') as response:
data = response.read().decode()
print(data)
在这个示例中,我们通过 ProxyHandler
类配置了 HTTP 代理。
- 认证
urllib 库支持基本认证和摘要认证。以下是一个使用基本认证的示例:
import urllib.request
password_mgr = urllib.request.HTTPPasswordMgrWithDefaultRealm()
password_mgr.add_password(None, 'https://api.example.com', 'username', 'password')
auth_handler = urllib.request.HTTPBasicAuthHandler(password_mgr)
opener = urllib.request.build_opener(auth_handler)
with opener.open('https://api.example.com/data') as response:
data = response.read().decode()
print(data)
在这个示例中,我们使用 HTTPPasswordMgrWithDefaultRealm
和 HTTPBasicAuthHandler
类指定了基本认证的用户名和密码。
四、使用 http.client 模块的高级功能
http.client 模块提供了一些高级功能,如自定义请求头、处理重定向和错误处理等。
- 自定义请求头
在发送请求时,可以使用 HTTPConnection
或 HTTPSConnection
对象自定义请求头。例如:
import http.client
conn = http.client.HTTPSConnection('api.example.com')
headers = {'User-Agent': 'my-app/0.0.1'}
conn.request('GET', '/data', headers=headers)
response = conn.getresponse()
print(response.status)
print(response.read().decode())
conn.close()
在这个示例中,我们通过 headers
参数指定了自定义的请求头。
- 处理重定向
http.client 模块不会自动处理 HTTP 重定向,需要手动处理。例如:
import http.client
import urllib.parse
conn = http.client.HTTPConnection('example.com')
conn.request('GET', '/')
response = conn.getresponse()
if response.status in (301, 302):
location = response.getheader('Location')
new_url = urllib.parse.urlparse(location)
conn = http.client.HTTPConnection(new_url.netloc)
conn.request('GET', new_url.path)
response = conn.getresponse()
print(response.status)
print(response.read().decode())
conn.close()
在这个示例中,我们手动处理了 HTTP 重定向。
- 错误处理
http.client 模块提供了异常处理机制,可以捕获并处理请求中的错误。例如:
import http.client
try:
conn = http.client.HTTPSConnection('api.example.com')
conn.request('GET', '/data')
response = conn.getresponse()
print(response.status)
print(response.read().decode())
except http.client.HTTPException as err:
print(f'HTTP error occurred: {err}')
finally:
conn.close()
在这个示例中,我们通过异常处理捕获并处理了请求中的错误。
五、总结
Python 提供了多种方法来调用 HTTP 接口,包括使用 requests 库、urllib 库和 http.client 模块。requests 库因其简洁易用而被广泛推荐,而 urllib 和 http.client 模块则提供了更多的控制选项。在调用接口时,可以使用这些库提供的高级功能,如会话管理、请求参数、请求头、认证、超时和错误处理等,以满足不同的需求。根据具体应用场景选择合适的库和功能,可以更高效地进行接口调用。
相关问答FAQs:
如何使用Python发送HTTP请求以调用接口?
在Python中,可以使用requests库轻松发送HTTP请求来调用接口。首先,确保安装了requests库,可以通过命令pip install requests
来安装。使用该库时,您只需构建请求并调用接口的URL。例如,使用GET请求可以这样写:
import requests
response = requests.get('https://api.example.com/data')
print(response.json())
对于POST请求,可以通过以下方式发送数据:
import requests
data = {'key': 'value'}
response = requests.post('https://api.example.com/data', json=data)
print(response.json())
在调接口时如何处理异常和错误?
在调用接口的过程中,可能会遇到网络问题或者API返回错误。为了提高代码的健壮性,可以使用try-except结构来捕获异常。例如:
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # 检查请求是否成功
data = response.json()
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except requests.exceptions.RequestException as e:
print(f"Error occurred: {e}")
通过这种方式,可以有效地处理HTTP错误并做出相应的处理。
如何在Python中设置请求头以调用接口?
某些API在调用时需要特定的请求头,例如认证信息或内容类型。可以通过在请求中添加headers参数来设置这些头。例如:
import requests
url = 'https://api.example.com/data'
headers = {
'Authorization': 'Bearer your_token',
'Content-Type': 'application/json'
}
response = requests.get(url, headers=headers)
print(response.json())
这样,您可以根据API的要求灵活配置请求头,确保接口调用顺利进行。
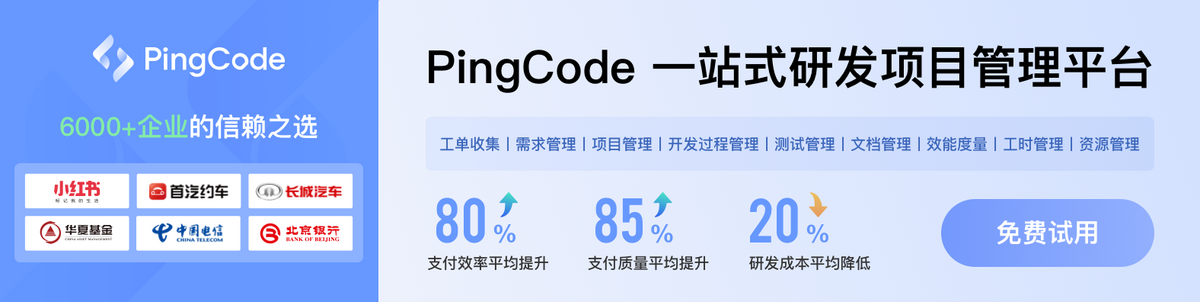