在Python中定义一个“car”(汽车)类可以通过使用类定义和面向对象编程(OOP)原则来实现。首先,创建一个类来表示汽车对象、在类中定义汽车的属性(如颜色、品牌、型号)和行为(如启动、加速)。通过面向对象的方式,代码更加模块化和易于维护。可以通过使用构造函数__init__
方法来初始化汽车对象的属性。例如,为汽车定义颜色、品牌、型号等属性,并为其实现启动、加速等方法,使汽车类更加完善和易于使用。
一、CAR类的基本定义
定义一个“car”类是面向对象编程中的基本任务之一。Python作为一种面向对象的语言,提供了丰富的工具来创建和管理对象。在Python中,类是一种用户自定义的数据类型,包含数据和方法的定义。
- 类的基本结构
在Python中,定义一个类使用class
关键字,类名一般使用大写字母开头。以下是一个基本的“car”类的定义结构:
class Car:
def __init__(self, brand, model, color):
self.brand = brand
self.model = model
self.color = color
在这个类中,__init__
方法是一个构造函数,用来初始化对象的属性。self
是一个指向实例对象本身的引用,在类的方法中第一个参数必须是self
。
- 属性的定义
汽车类的属性可以包括品牌(brand)、型号(model)和颜色(color)等,这些属性在__init__
方法中进行初始化。属性是类中的变量,用于存储对象的状态。
class Car:
def __init__(self, brand, model, color):
self.brand = brand # 汽车品牌
self.model = model # 汽车型号
self.color = color # 汽车颜色
二、定义汽车的行为
汽车不仅有静态的属性,还有动态的行为。行为通过类的方法来定义,方法是类的函数,用于描述对象的行为。
- 启动方法
启动是汽车的基本行为之一,可以通过一个方法来模拟这个动作。
class Car:
def __init__(self, brand, model, color):
self.brand = brand
self.model = model
self.color = color
def start(self):
print(f"{self.brand} {self.model} is starting.")
在这个方法中,self
参数使得我们可以访问实例属性,并通过打印语句模拟汽车启动。
- 加速方法
加速是另一个常见的汽车行为,可以通过增加速度属性和加速方法来实现。
class Car:
def __init__(self, brand, model, color, speed=0):
self.brand = brand
self.model = model
self.color = color
self.speed = speed
def accelerate(self, increment):
self.speed += increment
print(f"{self.brand} {self.model} accelerates to {self.speed} km/h.")
accelerate
方法接受一个参数increment
,用于增加当前速度,并更新speed
属性。
三、实例化和使用CAR对象
定义完类后,可以通过实例化来创建对象,并使用对象的方法来模拟汽车的行为。
- 创建汽车对象
通过类名调用,传入必要的参数创建对象。
my_car = Car("Toyota", "Corolla", "Red")
- 使用汽车对象的方法
一旦创建了汽车对象,就可以调用其方法来执行行为。
my_car.start()
my_car.accelerate(20)
以上代码将模拟汽车启动并加速的过程。
四、扩展CAR类的功能
在实际应用中,汽车类可能需要更复杂的行为和属性,可以通过增加更多的方法和属性来扩展类的功能。
- 增加刹车功能
刹车是汽车的重要功能之一,可以通过减少速度的方法来实现。
class Car:
def __init__(self, brand, model, color, speed=0):
self.brand = brand
self.model = model
self.color = color
self.speed = speed
def brake(self, decrement):
self.speed = max(0, self.speed - decrement)
print(f"{self.brand} {self.model} slows down to {self.speed} km/h.")
- 增加显示状态功能
显示当前汽车状态的方法可以帮助我们了解汽车的当前状态。
class Car:
def __init__(self, brand, model, color, speed=0):
self.brand = brand
self.model = model
self.color = color
self.speed = speed
def display_status(self):
print(f"Car: {self.brand} {self.model}, Color: {self.color}, Speed: {self.speed} km/h.")
五、使用继承和多态增强CAR类
面向对象编程的重要特性是继承和多态,通过这些特性可以更好地组织和扩展代码。
- 继承
可以通过继承创建更具体的汽车类型,例如电动车。
class ElectricCar(Car):
def __init__(self, brand, model, color, battery_capacity, speed=0):
super().__init__(brand, model, color, speed)
self.battery_capacity = battery_capacity
def charge(self):
print(f"{self.brand} {self.model} is charging with {self.battery_capacity} kWh capacity.")
- 多态
多态允许我们调用子类的方法,而无需知道对象的确切类型。
def car_action(car):
car.start()
car.accelerate(30)
my_car = Car("Toyota", "Corolla", "Red")
electric_car = ElectricCar("Tesla", "Model S", "White", 100)
car_action(my_car)
car_action(electric_car)
通过这种方式,可以灵活地处理不同类型的汽车对象,而不需要更改函数的实现。
总结
通过定义一个“car”类,并为其添加属性和方法,我们可以有效地模拟汽车的行为。在此过程中,利用Python的面向对象特性,使代码更加模块化和可扩展。继承和多态的使用进一步增强了代码的灵活性,使得我们可以轻松创建不同类型的汽车对象并进行操作。通过这种方式,Python的面向对象编程为我们提供了强大的工具来解决复杂的编程问题。
相关问答FAQs:
如何在Python中定义一个表示汽车的类?
在Python中,可以通过定义一个类来表示汽车。类可以包含汽车的属性(如品牌、颜色、型号等)和方法(如加速、刹车等)。以下是一个简单的例子:
class Car:
def __init__(self, brand, model, color):
self.brand = brand
self.model = model
self.color = color
def accelerate(self):
print(f"{self.brand} {self.model} is accelerating.")
def brake(self):
print(f"{self.brand} {self.model} is braking.")
通过这个类,您可以创建汽车的实例,并调用相应的方法。
在Python中如何为汽车类添加功能?
可以通过在类中添加更多的方法来扩展汽车类的功能。例如,可以添加方法来显示汽车信息、计算油耗等。以下是一个扩展的示例:
class Car:
def __init__(self, brand, model, color, fuel_efficiency):
self.brand = brand
self.model = model
self.color = color
self.fuel_efficiency = fuel_efficiency # 每加仑多少英里
def display_info(self):
print(f"Car: {self.brand} {self.model}, Color: {self.color}, Fuel Efficiency: {self.fuel_efficiency} MPG")
def calculate_range(self, fuel):
return fuel * self.fuel_efficiency # 计算行驶范围
这样,用户可以获取汽车的详细信息并计算其行驶范围。
在Python中如何创建汽车对象并使用其方法?
创建汽车对象非常简单,只需调用类的构造函数并传入所需的参数。以下是如何创建汽车对象并调用其方法的示例:
my_car = Car("Toyota", "Camry", "Red", 28)
my_car.display_info() # 显示汽车信息
print("Range with 10 gallons:", my_car.calculate_range(10), "miles") # 计算行驶范围
my_car.accelerate() # 调用加速方法
通过这种方式,您可以轻松创建和使用汽车对象。
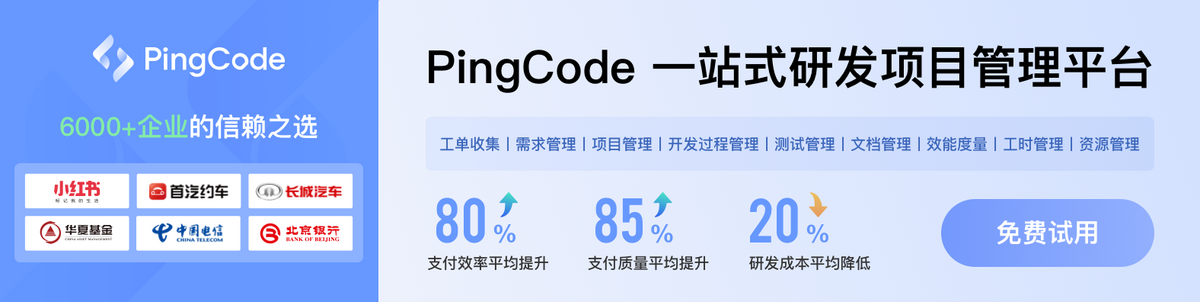