在Python中选择菜单可以通过定义函数、使用字典映射菜单选项、利用循环来实现用户交互。使用函数有助于代码的组织和可重用性;字典映射可以使菜单选项与功能直接关联,方便管理和扩展;循环可以保持菜单的持续显示和操作的重复执行。函数、字典映射、循环是实现菜单选择的三大关键元素。下面我将详细描述如何在Python中有效地实现菜单选择。
一、定义函数实现菜单功能
函数是Python中组织代码的基础单元。在实现菜单选择时,我们可以为每个菜单选项定义一个函数,这样不仅可以使代码更具模块化,还能提高可读性和维护性。通过将每个功能封装在一个函数中,我们可以轻松地在主程序中调用这些功能。
1.1 定义功能函数
首先,为每个菜单选项定义一个独立的函数。例如,如果我们有一个简单的计算器菜单,可能包括加法、减法、乘法和除法功能,我们可以为每个功能定义一个函数。
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
if b != 0:
return a / b
else:
return "Cannot divide by zero"
1.2 定义菜单函数
定义一个函数来显示菜单并处理用户输入。此函数将调用上面定义的功能函数。
def show_menu():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice(1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(f"The result is: {add(num1, num2)}")
elif choice == '2':
print(f"The result is: {subtract(num1, num2)}")
elif choice == '3':
print(f"The result is: {multiply(num1, num2)}")
elif choice == '4':
print(f"The result is: {divide(num1, num2)}")
else:
print("Invalid input")
二、使用字典映射菜单选项
字典是一种非常强大的数据结构,适合用来将菜单选项和功能直接关联。我们可以使用字典来简化菜单选择的实现,并使代码更加灵活和易于扩展。
2.1 创建字典映射
将菜单选项与其对应的功能函数映射起来:
operations = {
'1': add,
'2': subtract,
'3': multiply,
'4': divide
}
2.2 使用字典进行选择
在菜单函数中,使用字典来调用对应的功能函数:
def show_menu_with_dict():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice(1/2/3/4): ")
if choice in operations:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
result = operations[choice](num1, num2)
print(f"The result is: {result}")
else:
print("Invalid input")
三、利用循环实现持续操作
在实际应用中,菜单通常需要持续显示,直到用户选择退出。通过使用循环,我们可以实现这一需求,使得用户可以进行多次操作。
3.1 使用循环保持菜单显示
可以使用while
循环让菜单一直显示,直到用户选择退出:
def main():
while True:
show_menu_with_dict()
cont = input("Do you want to continue? (yes/no): ")
if cont.lower() != 'yes':
break
if __name__ == "__main__":
main()
3.2 添加退出选项
在菜单中添加一个选项,用于退出程序:
def show_menu_with_exit():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Exit")
choice = input("Enter choice(1/2/3/4/5): ")
if choice == '5':
print("Exiting the program...")
return False
if choice in operations:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
result = operations[choice](num1, num2)
print(f"The result is: {result}")
else:
print("Invalid input")
return True
def main_with_exit():
while True:
if not show_menu_with_exit():
break
if __name__ == "__main__":
main_with_exit()
四、增强用户体验和功能扩展
通过对菜单系统进行进一步的优化,我们可以显著提升用户体验,并为将来的扩展做好准备。
4.1 输入验证和错误处理
在用户输入数值时,进行验证以确保输入正确,并提供相应的错误处理机制。
def get_number(prompt):
while True:
try:
return float(input(prompt))
except ValueError:
print("Invalid input. Please enter a number.")
def show_menu_with_validation():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Exit")
choice = input("Enter choice(1/2/3/4/5): ")
if choice == '5':
print("Exiting the program...")
return False
if choice in operations:
num1 = get_number("Enter first number: ")
num2 = get_number("Enter second number: ")
result = operations[choice](num1, num2)
print(f"The result is: {result}")
else:
print("Invalid input")
return True
def main_with_validation():
while True:
if not show_menu_with_validation():
break
if __name__ == "__main__":
main_with_validation()
4.2 增加新的功能
当需要增加新的功能时,只需定义新的函数并更新字典映射即可。例如,添加一个求平方根的功能:
import math
def sqrt(a):
if a >= 0:
return math.sqrt(a)
else:
return "Cannot take square root of negative number"
operations['6'] = sqrt
def show_menu_with_sqrt():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Exit")
print("6. Square root")
choice = input("Enter choice(1/2/3/4/5/6): ")
if choice == '5':
print("Exiting the program...")
return False
if choice in operations:
if choice == '6':
num = get_number("Enter number: ")
result = operations[choice](num)
else:
num1 = get_number("Enter first number: ")
num2 = get_number("Enter second number: ")
result = operations[choice](num1, num2)
print(f"The result is: {result}")
else:
print("Invalid input")
return True
def main_with_sqrt():
while True:
if not show_menu_with_sqrt():
break
if __name__ == "__main__":
main_with_sqrt()
通过以上步骤,我们可以在Python中实现一个灵活、易于扩展的菜单选择系统。这种方法不仅提高了代码的可读性和维护性,还为将来的功能扩展提供了便利。
相关问答FAQs:
如何使用Python创建一个动态菜单?
在Python中,可以使用循环和条件语句来创建一个动态菜单。通过定义一个函数,可以让用户根据提示输入选择的菜单项,然后根据用户的选择执行相应的操作。使用while
循环可以持续显示菜单,直到用户选择退出。这种方法不仅简洁易懂,还能增强用户交互体验。
有哪些Python库可以帮助我创建更复杂的菜单?
有许多Python库可以帮助创建更复杂的菜单,例如curses
和tkinter
。curses
库适合在终端中创建文本用户界面,而tkinter
则适合开发图形用户界面(GUI)。使用这些库可以实现更多的交互功能,比如按钮、下拉菜单和对话框,从而提供更丰富的用户体验。
如何处理用户在菜单中无效选择的情况?
在设计菜单时,处理用户无效选择非常重要。可以通过条件判断来验证用户输入,若输入不在预设的选项中,应提示用户输入有效的选项。这样可以提高程序的健壮性,确保用户体验不受影响。通过循环结构,可以让用户不断尝试直到输入有效的选项为止。
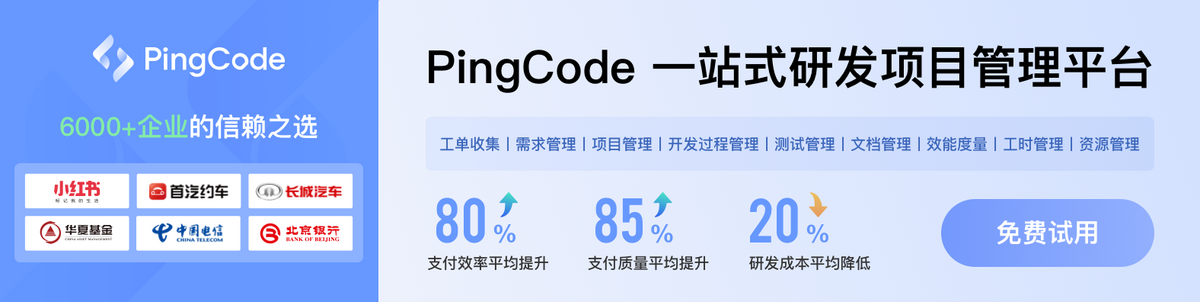