要在Python中随机选择图片,你可以使用多个库和方法,例如os库来列出目录中的文件、random库来选择随机文件、以及PIL(Pillow)库来处理和显示图像。首先,你需要列出目标目录中的所有图片文件,然后使用random.choice()函数来随机选择其中一张图片进行操作。
为了详细展开这一过程,我们将详细介绍如何使用Python实现这一功能,包括如何设置环境、如何处理图像文件以及如何在程序中应用随机选择的图片。
一、环境准备
在处理图像之前,确保你的Python环境中安装了所需的库。你需要安装Pillow库来处理图片。你可以使用以下命令安装:
pip install pillow
Pillow是Python Imaging Library的一个分支,它支持打开、操作和保存许多不同格式的图像文件。
二、列出目录中的图片文件
在选择随机图片之前,你需要列出某个目录中的所有图片文件。我们可以使用os库来实现这一点。
import os
def list_images(directory):
return [f for f in os.listdir(directory) if f.endswith(('.png', '.jpg', '.jpeg', '.gif'))]
在这里,list_images
函数接收一个目录路径作为参数,并返回一个包含该目录中所有图片文件名的列表。我们使用str.endswith()
方法来筛选常见的图片格式。
三、随机选择一张图片
有了图片文件列表后,我们可以使用random库来随机选择一张图片。
import random
def select_random_image(directory):
images = list_images(directory)
return random.choice(images) if images else None
在select_random_image
函数中,我们首先调用list_images
函数获取图片文件列表,然后使用random.choice()
从中随机选择一张图片。如果目录中没有图片文件,我们返回None
。
四、打开和显示随机选择的图片
选定图片后,我们可以使用Pillow库来打开和显示这张图片。
from PIL import Image
def open_and_display_image(directory, image_name):
if image_name:
img_path = os.path.join(directory, image_name)
with Image.open(img_path) as img:
img.show()
在open_and_display_image
函数中,我们首先构建图片的完整路径,然后使用Image.open()
打开图片,并调用img.show()
来显示图片。这将打开一个窗口,显示选中的图片。
五、完整的Python脚本
以下是一个完整的Python脚本,演示如何在特定目录中随机选择一张图片并显示:
import os
import random
from PIL import Image
def list_images(directory):
return [f for f in os.listdir(directory) if f.endswith(('.png', '.jpg', '.jpeg', '.gif'))]
def select_random_image(directory):
images = list_images(directory)
return random.choice(images) if images else None
def open_and_display_image(directory, image_name):
if image_name:
img_path = os.path.join(directory, image_name)
with Image.open(img_path) as img:
img.show()
def main():
directory = '/path/to/your/image/directory'
image_name = select_random_image(directory)
if image_name:
print(f"Selected Image: {image_name}")
open_and_display_image(directory, image_name)
else:
print("No images found in the directory.")
if __name__ == "__main__":
main()
六、注意事项
-
路径问题:确保
directory
变量中设置的路径是你的图片目录的绝对路径或相对路径。 -
文件格式:根据需要,调整
list_images
函数中过滤图片格式的文件扩展名。 -
图像库:如果你需要对图像进行进一步处理(如调整大小、旋转等),Pillow库提供了丰富的API来实现这些功能。
通过上述步骤,你可以使用Python随机选择并显示目录中的一张图片。这一过程结合了文件操作、随机选择和图像处理,是许多图像处理任务的基础。
相关问答FAQs:
如何在Python中随机选择图片?
在Python中,可以使用os
模块结合random
模块来随机选择文件夹中的图片。首先,利用os.listdir()
方法获取文件夹内所有文件的列表,然后利用random.choice()
从中随机选择一张图片。确保在选择时只筛选出图片格式的文件,例如.jpg、.png等。
有哪些库可以帮助我在Python中处理图片?
Python有多个强大的库可以处理图片,例如PIL
(Python Imaging Library)和OpenCV
。PIL可以用于打开、操作和保存多种格式的图片,而OpenCV则更适合进行复杂的图像处理和计算机视觉任务。这些库可以与随机选择图片的功能结合,进行更丰富的操作。
如何在Python中显示随机选择的图片?
要显示随机选择的图片,可以使用matplotlib
库。通过matplotlib.pyplot
中的imshow()
方法可以展示图片。在选择图片后,利用PIL
库打开它,并将其转换为适合显示的格式,接着调用show()
方法即可弹出窗口显示图片。
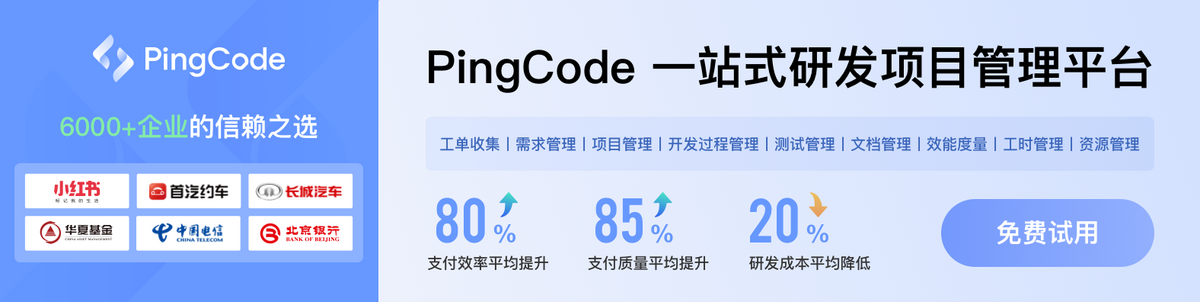