在Python中,可以通过多种方法去掉字符串中的特定字符或空格,常用的方法包括:使用strip()
方法去掉字符串两端的空格、使用replace()
方法替换特定字符为空字符串、使用re.sub()
函数进行正则表达式替换。 其中,strip()
方法对于去掉字符串两端的空格和特定字符非常有效,而replace()
方法则适用于去掉字符串中所有出现的特定字符。re.sub()
函数则提供了更为灵活的方式,通过正则表达式匹配模式去掉复杂的字符组合。以下将详细展开这些方法的使用。
一、使用strip()
方法
strip()
方法用于去除字符串首尾的空格或指定字符。strip()
可以通过参数指定需要去掉的字符,如果不指定,默认去掉空格。
1. 去掉两端空格
text = " Hello, World! "
cleaned_text = text.strip()
print(cleaned_text) # 输出: "Hello, World!"
2. 去掉特定字符
如果需要去掉字符串两端的特定字符,可以在strip()
中指定该字符。
text = "---Hello, World!---"
cleaned_text = text.strip('-')
print(cleaned_text) # 输出: "Hello, World!"
二、使用replace()
方法
replace()
方法用于替换字符串中的指定字符或子字符串。它可以将所有匹配的字符或子字符串替换为新的字符或子字符串。
1. 去掉所有特定字符
text = "Hello, World!"
cleaned_text = text.replace("o", "")
print(cleaned_text) # 输出: "Hell, Wrld!"
2. 去掉子字符串
text = "Hello, World!"
cleaned_text = text.replace("World", "")
print(cleaned_text) # 输出: "Hello, !"
三、使用re.sub()
函数
re.sub()
函数是Python正则表达式模块re
中的一个函数,用于替换字符串中匹配正则表达式的部分。它提供了一种强大的方式来去除复杂的字符模式。
1. 去掉所有数字
import re
text = "H3ll0, W0rld!"
cleaned_text = re.sub(r'\d', '', text)
print(cleaned_text) # 输出: "Hll, Wrld!"
2. 去掉所有非字母字符
import re
text = "Hello, World! 123"
cleaned_text = re.sub(r'[^a-zA-Z]', '', text)
print(cleaned_text) # 输出: "HelloWorld"
四、结合多种方法
在实际应用中,可能需要结合多种方法来处理字符串。例如,可以先使用strip()
去掉两端的空格,再使用replace()
去掉中间的特定字符。
text = " --Hello, World!-- "
去掉两端的空格和横杠
cleaned_text = text.strip().strip('-')
去掉所有的逗号
cleaned_text = cleaned_text.replace(',', '')
print(cleaned_text) # 输出: "Hello World!"
五、处理大规模文本
在处理大规模文本时,以上方法仍然有效,但需要注意效率问题。例如,可以通过将文本分割为较小的块,逐块处理以节省内存。
1. 分块处理
def clean_large_text(text):
# 分块大小
chunk_size = 1024
cleaned_text = []
for i in range(0, len(text), chunk_size):
chunk = text[i:i + chunk_size]
# 清理每个块
cleaned_chunk = chunk.strip().replace(',', '')
cleaned_text.append(cleaned_chunk)
return ''.join(cleaned_text)
large_text = " --Hello, World!-- " * 1000
cleaned_large_text = clean_large_text(large_text)
print(cleaned_large_text[:50]) # 输出部分结果
六、性能优化
为了提高处理速度,可以考虑使用以下技术:
- 多线程/多进程处理:对于极大规模的文本处理,可以使用Python的多线程或多进程模块(如
concurrent.futures
或multiprocessing
)来并行处理。 - 使用高效的数据结构:在需要频繁操作字符串的场景中,可以考虑使用
StringIO
模块或其他高效的数据结构来减少内存消耗和提高操作效率。 - 编译正则表达式:在使用
re.sub()
时,先编译正则表达式可以提高匹配效率。
import re
from concurrent.futures import ThreadPoolExecutor
def clean_chunk(chunk):
# 使用编译后的正则表达式
pattern = re.compile(r'[^a-zA-Z]')
return pattern.sub('', chunk)
def clean_large_text_concurrently(text):
chunk_size = 1024
cleaned_chunks = []
with ThreadPoolExecutor() as executor:
for i in range(0, len(text), chunk_size):
chunk = text[i:i + chunk_size]
# 并行清理每个块
future = executor.submit(clean_chunk, chunk)
cleaned_chunks.append(future.result())
return ''.join(cleaned_chunks)
示例大文本
large_text = "Hello, World! 123 " * 1000
cleaned_large_text_concurrent = clean_large_text_concurrently(large_text)
print(cleaned_large_text_concurrent[:50]) # 输出部分结果
通过以上各种方法,能够有效地去掉Python字符串中的空格、特定字符或复杂模式。根据具体需求选择合适的方法可以提高处理效率和代码的可读性。
相关问答FAQs:
如何在Python中去掉字符串的空格?
在Python中,可以使用strip()
方法去掉字符串前后的空格,例如:my_string = " Hello World "
,使用my_string.strip()
将返回"Hello World"
。如果只想去掉前面的空格,可以使用lstrip()
,后面的空格则使用rstrip()
。
Python中如何移除特定字符?
要移除字符串中的特定字符,可以使用replace()
方法。比如,my_string = "Hello World!"
,如果想去掉感叹号,可以使用my_string.replace("!", "")
,这将返回"Hello World"
。此外,translate()
方法也可以实现更复杂的字符替换。
如何在Python中删除字符串的某些子串?
使用replace()
方法同样可以删除特定的子串。例如,如果字符串是"I love Python programming"
,想要删除"Python "
,可以使用my_string.replace("Python ", "")
,最终结果为"I love programming"
。对于多个子串的删除,可以结合使用replace()
进行多次调用。
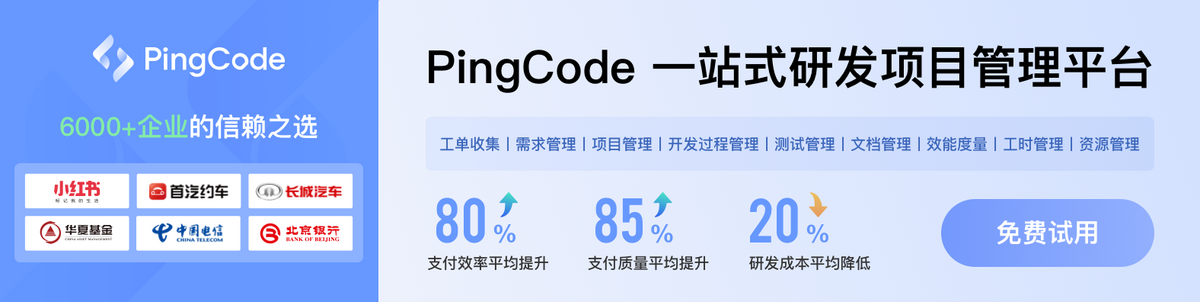