在Python中,多线程是一种能够让程序同时执行多个任务的技术。Python通过使用threading
模块、concurrent.futures
模块、以及multiprocessing
模块来实现多线程。其中,threading
模块是最常用的,因为它为线程管理提供了简单的接口。虽然Python的全局解释器锁(GIL)限制了多线程在CPU密集型任务中的效率,但在I/O密集型任务中,多线程仍然可以显著提升程序性能。通过合理使用多线程,可以在不增加硬件成本的情况下提高程序的执行效率。
下面我们将详细探讨Python中多线程的实现及其应用。
一、THREADING
模块的使用
threading
模块是Python标准库中用于线程管理的模块。它提供了线程对象和多个工具来管理线程。
- 创建线程
在Python中,可以通过直接实例化threading.Thread
来创建一个线程。线程对象的target
参数指定线程要执行的函数,args
参数用于传递给函数的参数。
import threading
def print_numbers():
for i in range(5):
print(i)
创建线程
thread = threading.Thread(target=print_numbers)
启动线程
thread.start()
等待线程完成
thread.join()
- 线程同步
由于多个线程可能会同时访问共享资源,因此需要同步机制来避免竞态条件。threading
模块提供了Lock
对象来实现互斥锁。
import threading
lock = threading.Lock()
def thread_safe_increment(counter):
with lock:
for _ in range(1000):
counter[0] += 1
counter = [0]
threads = [threading.Thread(target=thread_safe_increment, args=(counter,)) for _ in range(10)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print("Counter:", counter[0])
- 线程间通信
threading
模块提供了多个工具来实现线程间通信,如Queue
对象。Queue
对象是线程安全的,可以在多个线程之间安全地传递数据。
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print("Produced:", i)
def consumer(q):
while not q.empty():
item = q.get()
print("Consumed:", item)
q.task_done()
q = queue.Queue()
t1 = threading.Thread(target=producer, args=(q,))
t2 = threading.Thread(target=consumer, args=(q,))
t1.start()
t2.start()
t1.join()
t2.join()
二、CONCURRENT.FUTURES
模块
concurrent.futures
模块提供了一个高级接口来管理线程。它提供了ThreadPoolExecutor
类,用于在一个池中管理多个线程。
- 使用
ThreadPoolExecutor
ThreadPoolExecutor
可以用于管理线程池,通过提交任务来执行。
import concurrent.futures
def square(n):
return n * n
numbers = [1, 2, 3, 4, 5]
with concurrent.futures.ThreadPoolExecutor() as executor:
results = executor.map(square, numbers)
print(list(results))
- 管理任务
ThreadPoolExecutor
提供了submit
方法,可以用于提交单个任务,并返回一个Future
对象。Future
对象可以用于获取任务的结果。
import concurrent.futures
def square(n):
return n * n
with concurrent.futures.ThreadPoolExecutor() as executor:
future = executor.submit(square, 2)
print("Result:", future.result())
三、MULTIPROCESSING
模块
尽管threading
和concurrent.futures
模块在I/O密集型任务中表现良好,但在CPU密集型任务中,由于GIL的限制,它们的性能可能不理想。multiprocessing
模块通过使用多个进程而非线程来绕过GIL,从而提高性能。
- 使用
multiprocessing
multiprocessing
模块提供了一个与threading
模块类似的接口,但它是基于进程而非线程的。
from multiprocessing import Process
def print_numbers():
for i in range(5):
print(i)
process = Process(target=print_numbers)
process.start()
process.join()
- 进程间通信
multiprocessing
模块提供了Queue
和Pipe
对象,用于在进程之间进行通信。
from multiprocessing import Process, Queue
def producer(q):
for i in range(5):
q.put(i)
print("Produced:", i)
def consumer(q):
while not q.empty():
item = q.get()
print("Consumed:", item)
q = Queue()
p1 = Process(target=producer, args=(q,))
p2 = Process(target=consumer, args=(q,))
p1.start()
p2.start()
p1.join()
p2.join()
- 共享内存
multiprocessing
模块还提供了Value
和Array
对象,用于在进程之间共享数据。
from multiprocessing import Process, Value
def increment(counter):
for _ in range(1000):
with counter.get_lock():
counter.value += 1
counter = Value('i', 0)
processes = [Process(target=increment, args=(counter,)) for _ in range(10)]
for process in processes:
process.start()
for process in processes:
process.join()
print("Counter:", counter.value)
四、多线程的应用场景
- I/O密集型任务
多线程非常适合I/O密集型任务,如网络请求、文件读写等。在这些任务中,线程通常会在等待I/O操作完成时阻塞。多线程可以在一个线程阻塞时,切换到另一个线程继续执行,从而提高效率。
- 并行化任务
多线程可以用于并行化多个独立任务。例如,在一个Web服务器中,可以为每个请求创建一个线程,以便同时处理多个请求。
- 任务调度
多线程可以用于实现任务调度系统,例如定时任务。通过为每个任务创建一个线程,可以更容易地管理和调度任务。
- 增强用户体验
在GUI应用程序中,多线程可以用于保持界面响应。例如,在下载文件时,可以在后台线程中执行下载操作,从而不阻塞用户界面。
总结来说,Python中的多线程提供了一种有效的方式来管理和执行并发任务。尽管GIL限制了多线程在某些情境下的效率,但通过合理使用threading
、concurrent.futures
和multiprocessing
模块,开发者仍然可以显著提高程序的性能和响应速度。
相关问答FAQs:
Python多线程的基本概念是什么?
Python中的多线程是一种并发编程的方式,允许同时运行多个线程以提高程序的执行效率。每个线程可以执行独立的任务,尤其适合I/O密集型操作,如网络请求、文件读写等。Python通过threading
模块提供了简单易用的多线程支持,使得开发者可以更轻松地实现并行处理。
使用多线程时需要注意哪些问题?
在运用多线程时,需谨慎处理共享资源,以避免线程间的数据竞争和不一致性问题。可以使用Lock
、RLock
等同步机制来确保同一时刻只有一个线程可以访问共享资源。此外,Python的全局解释器锁(GIL)也限制了多线程的性能提升,尤其是在CPU密集型任务中,可能需要考虑使用多进程替代。
如何在Python中创建和管理线程?
在Python中创建线程通常使用threading.Thread
类。可以通过继承该类并重写run
方法,或直接传入目标函数来实现线程的功能。管理线程可以通过start()
方法启动,使用join()
方法确保主线程等待子线程完成。通过设置线程的守护状态,可以控制线程在主程序结束时是否继续运行,从而实现更灵活的线程管理。
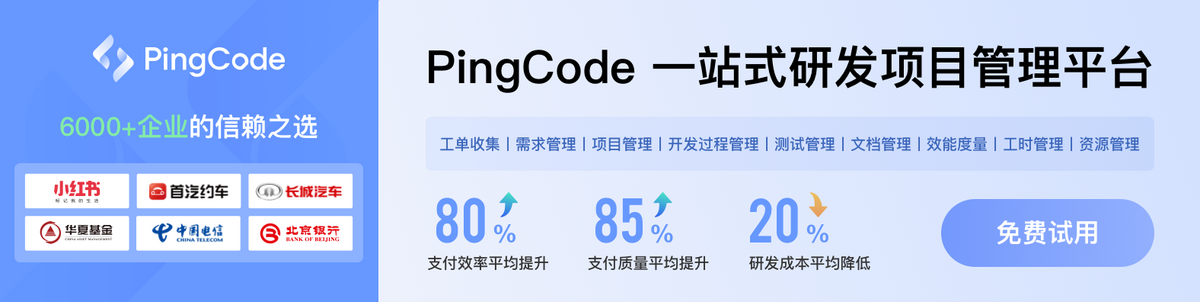