Python可以通过使用多个库来爬取TXT文件,例如requests、BeautifulSoup、scrapy等。首先,使用requests库发送HTTP请求获取网页内容,然后用BeautifulSoup解析HTML,提取TXT链接,最后下载并保存TXT文件。requests库的易用性、BeautifulSoup的强大解析能力以及scrapy的高效爬虫功能,使Python成为处理网络数据的强大工具。
一、使用REQUESTS库发送HTTP请求
requests库是一个用于发送HTTP请求的简单易用的库。它可以帮助我们获取网页的HTML内容。以下是如何使用requests库来获取网页内容的步骤:
-
安装requests库:
首先,需要确保已经安装了requests库。可以使用pip命令来安装:
pip install requests
-
发送HTTP请求:
使用requests库发送HTTP请求来获取网页内容。可以通过
requests.get(url)
方法来获取网页内容。import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
print("Successfully retrieved the webpage!")
html_content = response.text
else:
print("Failed to retrieve the webpage. Status code:", response.status_code)
-
处理HTTP响应:
当请求成功时,可以使用
response.text
属性获取网页的HTML内容。请求失败时,可以通过response.status_code
来检查错误代码。
二、解析HTML提取TXT链接
获取网页内容后,下一步是解析HTML并提取TXT文件的链接。这时可以使用BeautifulSoup库来解析HTML。
-
安装BeautifulSoup库:
使用pip安装BeautifulSoup库:
pip install beautifulsoup4
-
解析HTML:
使用BeautifulSoup库解析HTML内容,并提取所有的链接。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
找到所有的<a>标签
links = soup.find_all('a')
提取TXT文件的链接
txt_links = [link.get('href') for link in links if link.get('href').endswith('.txt')]
print("Found TXT links:", txt_links)
-
处理相对链接:
如果链接是相对路径,需要将其转换为绝对路径,可以使用
urljoin
方法。from urllib.parse import urljoin
txt_links = [urljoin(url, link) for link in txt_links]
三、下载并保存TXT文件
提取到TXT文件的链接后,可以下载并保存这些文件。
-
下载TXT文件:
使用requests库下载TXT文件,并将其内容保存到本地。
for txt_link in txt_links:
txt_response = requests.get(txt_link)
if txt_response.status_code == 200:
file_name = txt_link.split('/')[-1]
with open(file_name, 'w', encoding='utf-8') as file:
file.write(txt_response.text)
print(f"Downloaded {file_name}")
else:
print(f"Failed to download {txt_link}. Status code:", txt_response.status_code)
-
处理编码问题:
在保存文件时,可能会遇到编码问题。确保使用正确的编码格式(如
utf-8
)来保存文件。
四、使用SCRAPY进行高效爬取
对于需要爬取大量数据的情况,可以考虑使用Scrapy框架。Scrapy是一个快速、高效、可扩展的爬虫框架。
-
安装Scrapy:
使用pip安装Scrapy:
pip install scrapy
-
创建Scrapy项目:
使用Scrapy命令行工具创建一个新的Scrapy项目。
scrapy startproject myproject
cd myproject
scrapy genspider myspider example.com
-
编写爬虫代码:
编辑生成的爬虫代码,编写逻辑来爬取TXT文件。
import scrapy
class MySpider(scrapy.Spider):
name = "myspider"
start_urls = ['http://example.com']
def parse(self, response):
for href in response.css('a::attr(href)').getall():
if href.endswith('.txt'):
yield scrapy.Request(url=response.urljoin(href), callback=self.save_txt)
def save_txt(self, response):
file_name = response.url.split("/")[-1]
with open(file_name, 'wb') as file:
file.write(response.body)
self.log(f"Saved file {file_name}")
-
运行爬虫:
使用Scrapy命令运行爬虫。
scrapy crawl myspider
五、处理常见问题
在爬取TXT文件时,可能会遇到一些常见的问题,例如重定向、反爬虫机制、文件大小限制等。以下是一些解决方案:
-
处理重定向:
requests库默认会跟随重定向,但可以通过设置
allow_redirects=False
来禁用。response = requests.get(url, allow_redirects=False)
-
绕过反爬虫机制:
有些网站会通过检测User-Agent来阻止爬虫。可以通过设置请求头来模拟浏览器请求。
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url, headers=headers)
-
处理文件大小限制:
在下载大文件时,可以考虑分块下载,以减少内存占用。
with requests.get(txt_link, stream=True) as r:
with open(file_name, 'wb') as f:
for chunk in r.iter_content(chunk_size=8192):
f.write(chunk)
六、结论
通过使用Python的requests、BeautifulSoup和Scrapy库,可以高效地爬取TXT文件。requests库用于发送HTTP请求,获取网页内容;BeautifulSoup用于解析HTML,提取链接;Scrapy用于处理复杂的爬虫任务。通过结合使用这些工具,可以轻松实现对网络数据的抓取和处理。
相关问答FAQs:
如何使用Python爬取文本文件中的内容?
可以使用Python的内置函数和库来读取和爬取文本文件的内容。例如,使用open()
函数可以打开文件并读取其内容,结合requests
库可以从网络上下载文本文件。以下是一个简单的示例:
import requests
url = 'http://example.com/file.txt'
response = requests.get(url)
with open('file.txt', 'w') as file:
file.write(response.text)
确保在运行代码之前安装了requests库,可以通过pip install requests
命令安装。
在爬取txt文件时,如何处理编码问题?
在爬取txt文件时,编码问题可能会导致读取错误或乱码。使用requests
库时,可以通过response.encoding
属性设置正确的编码格式,比如utf-8
或gbk
。示例代码如下:
response.encoding = 'utf-8' # 或 'gbk'
这将确保在读取文件内容时,使用适当的编码格式。
如何提高Python爬虫的效率,快速爬取多个txt文件?
可以使用多线程或异步IO来提高爬虫的效率。使用concurrent.futures
库中的ThreadPoolExecutor
可以方便地实现多线程爬取。以下是一个示例:
from concurrent.futures import ThreadPoolExecutor
import requests
def download_file(url):
response = requests.get(url)
with open(url.split('/')[-1], 'w') as file:
file.write(response.text)
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(download_file, urls)
这样可以同时处理多个文件的下载,提高爬取效率。
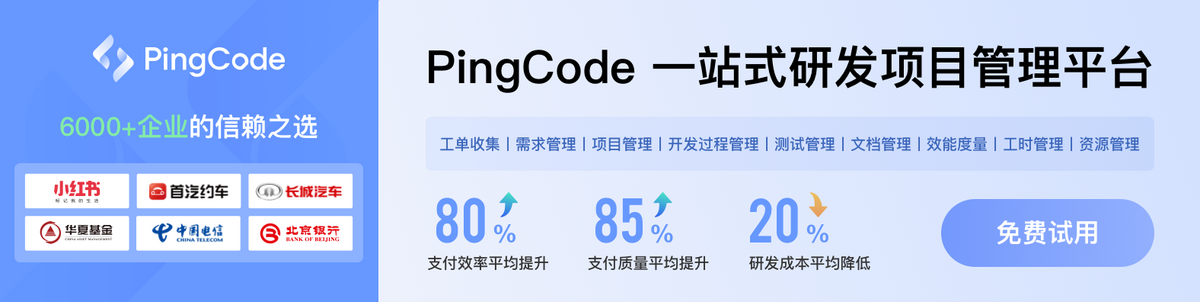