前端接Python接口的关键在于:使用HTTP请求与后端交互、解析返回的数据、处理错误和异常。在这些步骤中,使用AJAX或Fetch API发送请求是最常见的方法。
一、使用HTTP请求与后端交互
前端与后端的交互通常通过HTTP请求实现。Python后端通常会使用框架如Flask或Django来构建API接口,这些接口可以接收HTTP请求并返回响应。前端开发者可以使用AJAX或Fetch API来向这些接口发送请求。AJAX是一种基于JavaScript的技术,广泛应用于网页开发中。它允许网页在不重新加载的情况下更新数据。Fetch API是现代浏览器中提供的原生接口,用于发出网络请求并处理响应。
-
AJAX请求
AJAX使用XMLHttpRequest对象来与服务器进行交互。首先,需要创建一个XMLHttpRequest对象,然后调用其open方法来初始化请求。最后,通过send方法将请求发送到服务器。
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/data', true);
xhr.onload = function() {
if (xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send();
-
Fetch API
Fetch API提供了一个更加现代化和易于使用的接口来进行HTTP请求。它基于Promise,因此可以更加优雅地处理异步请求。
fetch('https://example.com/api/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
二、解析返回的数据
通常,Python接口会返回JSON格式的数据,因为JSON是一种轻量级的数据交换格式,易于解析和生成。在前端接收到响应后,需要对JSON数据进行解析,以便在页面上显示或进一步处理。
-
解析JSON数据
在Fetch API中,可以直接使用response.json()方法将响应转换为JSON对象。在AJAX中,则需要使用JSON.parse()方法对响应文本进行解析。
// Fetch API
fetch('https://example.com/api/data')
.then(response => response.json())
.then(data => {
console.log(data);
});
// AJAX
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/data', true);
xhr.onload = function() {
if (xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
console.log(data);
}
};
xhr.send();
三、处理错误和异常
在进行HTTP请求时,可能会遇到各种错误,如网络问题、服务器错误、无效的响应数据等。因此,前端开发者需要实现错误处理机制,以提高应用的健壮性。
-
Fetch API中的错误处理
Fetch API提供了.catch()方法来捕获请求中的任何错误。例如,网络错误或解析JSON时的错误。
fetch('https://example.com/api/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
-
AJAX中的错误处理
在AJAX中,可以通过检查XMLHttpRequest对象的状态码来检测错误,并在onerror事件中处理网络错误。
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/data', true);
xhr.onload = function() {
if (xhr.status >= 200 && xhr.status < 300) {
var data = JSON.parse(xhr.responseText);
console.log(data);
} else {
console.error('Request failed with status:', xhr.status);
}
};
xhr.onerror = function() {
console.error('Network error occurred');
};
xhr.send();
四、处理跨域问题
在许多情况下,前端和后端会运行在不同的域名或端口下,这会导致跨域请求的问题。浏览器的同源策略会阻止来自不同源的请求。为了允许跨域请求,后端需要配置CORS(跨域资源共享)。
-
在Python后端中配置CORS
如果使用Flask作为后端,可以安装并使用Flask-CORS扩展来配置CORS。
from flask import Flask
from flask_cors import CORS
app = Flask(__name__)
CORS(app)
@app.route('/api/data')
def get_data():
return {'key': 'value'}
如果使用Django,可以通过django-cors-headers来配置CORS。
# settings.py
INSTALLED_APPS = [
...
'corsheaders',
...
]
MIDDLEWARE = [
...
'corsheaders.middleware.CorsMiddleware',
...
]
CORS_ORIGIN_ALLOW_ALL = True
五、在应用中使用Python接口的数据
接收到Python接口返回的数据后,前端需要对这些数据进行处理并在页面上显示。例如,可以将数据绑定到HTML元素中,或使用JavaScript库如React或Vue进行动态渲染。
-
在HTML中显示数据
可以使用JavaScript将数据插入到HTML中。例如,如果接口返回一个用户列表,可以将其显示在表格中。
<table id="user-table">
<tr>
<th>Name</th>
<th>Email</th>
</tr>
</table>
<script>
fetch('https://example.com/api/users')
.then(response => response.json())
.then(users => {
const table = document.getElementById('user-table');
users.forEach(user => {
const row = table.insertRow();
const cellName = row.insertCell();
const cellEmail = row.insertCell();
cellName.textContent = user.name;
cellEmail.textContent = user.email;
});
});
</script>
-
在JavaScript框架中使用数据
在现代前端开发中,常用的JavaScript框架如React、Vue或Angular提供了强大的工具来处理数据和更新UI。
-
React
在React中,可以将请求数据存储在组件的状态中,并通过渲染函数动态更新UI。
import React, { useState, useEffect } from 'react';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://example.com/api/users')
.then(response => response.json())
.then(data => setUsers(data));
}, []);
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name} - {user.email}</li>
))}
</ul>
);
}
export default UserList;
-
Vue
在Vue中,可以利用组件的data属性存储数据,并使用模板语法在HTML中展示数据。
<template>
<ul>
<li v-for="user in users" :key="user.id">
{{ user.name }} - {{ user.email }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
users: []
};
},
mounted() {
fetch('https://example.com/api/users')
.then(response => response.json())
.then(data => {
this.users = data;
});
}
};
</script>
-
总之,前端接Python接口是现代Web开发中常见的任务。通过有效地使用HTTP请求、解析数据、处理错误、配置CORS以及在页面中展示数据,开发者可以创建强大而灵活的Web应用程序。每个步骤都需要仔细设计和实现,以确保应用的高效性和可靠性。
相关问答FAQs:
如何在前端使用Python接口的数据?
在前端使用Python接口的数据通常需要通过HTTP请求来获取。前端可以使用JavaScript的Fetch API或Axios库来发送GET或POST请求到Python后端的API端点。后端可以使用Flask或Django等框架提供API接口,前端接收到的数据可以通过JSON格式进行解析并展示在网页上。
哪些Python框架适合创建API供前端调用?
有多个Python框架适合创建API,最常用的包括Flask和Django。Flask是一个轻量级的框架,适合快速开发小型应用;而Django则提供了更全面的功能,适合构建复杂的Web应用。FastAPI也是一个流行的选择,它支持异步编程和自动生成API文档,非常适合高性能应用。
如何处理前端与Python接口之间的跨域问题?
在前端与Python接口之间进行数据交互时,可能会遇到跨域请求的问题。为了解决这个问题,可以在Python后端中使用CORS(跨源资源共享)。如果是Flask框架,可以使用flask-cors
库来设置允许特定来源的请求,而在Django中,可以通过django-cors-headers
来实现。这样就可以确保前端能够顺利访问后端API。
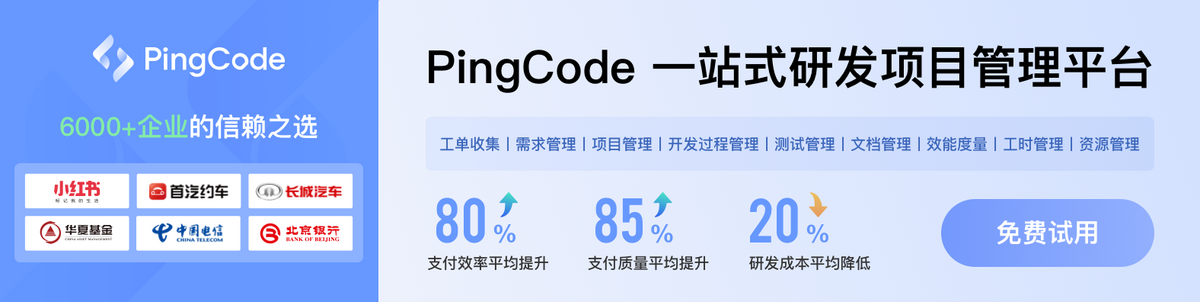