在Python中,可以通过多种方式依据Shell(sh)输入来执行命令并处理结果。主要方法包括使用subprocess
模块、os
模块、以及sh
库等。其中,subprocess
模块是最为推荐和强大的工具,因为它提供了更好的接口来启动和与子进程进行通信。下面将详细介绍这些方法。
一、使用subprocess
模块
subprocess
模块允许你生成新的进程、连接它们的输入/输出/错误管道,以及获取它们的返回值。它提供了比os.system
更强大和灵活的方式来执行shell命令。
1. 使用subprocess.run()
subprocess.run()
是Python 3.5中引入的一个函数,用于运行命令并等待命令完成。它返回一个CompletedProcess
实例。
import subprocess
def run_command(command):
result = subprocess.run(command, shell=True, text=True, capture_output=True)
if result.returncode == 0:
print("Command succeeded:", result.stdout)
else:
print("Command failed with error:", result.stderr)
run_command("ls -l")
在这个例子中,shell=True
允许命令通过shell执行,text=True
将输出作为字符串处理,capture_output=True
则捕获标准输出和标准错误。
2. 使用subprocess.Popen()
subprocess.Popen()
提供了更多的灵活性,允许你直接与子进程交互。
import subprocess
def execute_command(command):
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
if process.returncode == 0:
print("Output:", stdout)
else:
print("Error:", stderr)
execute_command("ls -l")
在这个例子中,Popen
对象允许我们在命令执行时进行交互,比如向进程发送输入或实时读取输出。
二、使用os
模块
os
模块是Python中用于与操作系统交互的模块之一。尽管os.system()
已经不再推荐使用,但了解其用法依然有意义。
import os
def system_command(command):
exit_code = os.system(command)
if exit_code == 0:
print("Command executed successfully")
else:
print("Command failed")
system_command("ls -l")
os.system()
的缺点是无法捕获命令的输出,且安全性较低。
三、使用sh
库
sh
库是Python的一个第三方库,提供了更为Pythonic的方式来执行Shell命令。它的使用方法非常简洁。
import sh
def sh_command(command):
try:
output = sh.ls("-l")
print("Command output:", output)
except sh.ErrorReturnCode as e:
print("Error:", e)
sh_command("ls -l")
sh
库的好处是命令执行的语法与Shell非常接近,且能够轻松处理命令的输入和输出。
四、Python Shell脚本与外部输入的集成
除了使用Python代码直接执行shell命令,Python脚本本身也可以通过外部输入集成到更大的Shell脚本中。可以通过参数传递、文件输入输出等方式实现。
1. 使用argparse
获取Shell输入
argparse
模块允许Python脚本接收来自Shell的命令行参数。
import argparse
def main():
parser = argparse.ArgumentParser(description="Process some integers.")
parser.add_argument("command", type=str, help="Command to execute")
args = parser.parse_args()
run_command(args.command)
if __name__ == "__main__":
main()
通过这种方式,可以在Shell中运行Python脚本并传递命令参数。
2. 通过文件进行输入输出
Python脚本可以与Shell通过文件进行交互,尤其适用于处理大量数据的场景。
def read_file(filename):
with open(filename, 'r') as file:
data = file.read()
print(data)
def write_file(filename, content):
with open(filename, 'w') as file:
file.write(content)
使用Shell重定向进行文件输入输出
python script.py < input.txt > output.txt
五、通过Python与Shell的联合使用实现复杂任务
在实际应用中,Python与Shell常常结合使用,以发挥各自的优势。例如,使用Shell进行系统级任务(如文件操作、调用命令行工具),而使用Python进行数据处理和逻辑控制。
1. 自动化任务
Python脚本可以用于自动化Shell命令的执行,例如定期备份、日志分析等。
import subprocess
import time
def backup_files():
while True:
subprocess.run("tar -czf backup.tar.gz /path/to/directory", shell=True)
time.sleep(86400) # 每24小时执行一次
backup_files()
2. 数据处理
在Shell中调用Python脚本进行复杂的数据处理任务。
#!/bin/bash
Shell脚本调用Python处理数据
output=$(python3 process_data.py < data.txt)
echo "Processed Data: $output"
3. 系统监控
结合使用Shell命令和Python脚本进行系统监控和警报。
import subprocess
def check_disk_usage():
result = subprocess.run("df -h", shell=True, text=True, capture_output=True)
if "100%" in result.stdout:
print("Disk is full!")
check_disk_usage()
总结:
在Python中依据Shell输入执行命令的方法有很多,主要包括subprocess
模块、os
模块、sh
库等。在这些方法中,subprocess
模块是最为推荐的,因为它提供了更强大的功能和更好的安全性。此外,Python脚本可以通过命令行参数、文件等方式与Shell进行交互,适用于自动化任务、数据处理、系统监控等复杂场景。通过合理选择和组合这些工具,可以极大提高开发效率和脚本的可维护性。
相关问答FAQs:
如何在Python中接收Shell脚本的输入?
在Python中,可以使用subprocess
模块来执行Shell命令并接收其输出。通过subprocess.run()
或subprocess.Popen()
可以实现与Shell的交互,具体取决于需要的复杂性。如果只需简单输出,可以使用check_output()
获取结果。
Python中如何处理Shell命令的错误?
处理Shell命令时,使用subprocess
模块可以捕获错误。通过设置check=True
参数,Python会在Shell命令返回非零状态时抛出异常。捕获subprocess.CalledProcessError
可以让你更好地处理这些错误,执行自定义的错误处理逻辑。
如何在Python中将Shell命令的输出存储到变量中?
可以通过subprocess.run()
或subprocess.check_output()
将Shell命令的输出直接存储到Python变量中。使用stdout=subprocess.PIPE
参数可以获取命令输出,而后通过result.stdout
访问该输出,确保对输出进行适当的解码以便于使用。
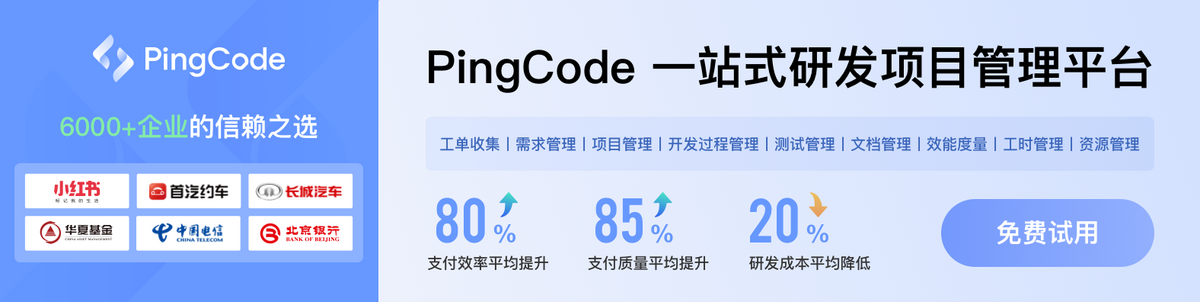