在Python中导入re模块的方法是使用import语句,通过该语句可以将re模块引入到你的Python脚本中,从而使用其提供的正则表达式功能。使用方法如下:import re、在脚本中使用re模块提供的函数来进行正则表达式操作。
在Python中,正则表达式是一种强大的工具,用于匹配字符串中的模式。re模块是Python中用于处理正则表达式的标准库模块,因此在进行任何正则表达式操作之前,必须先导入re模块。导入re模块的方法非常简单,只需在Python脚本的开头使用import语句即可,例如:import re
。一旦导入了re模块,就可以使用其提供的多种功能来处理字符串匹配、查找和替换操作。接下来,我们将详细介绍如何使用re模块的一些基本功能,并提供一些示例代码来演示其应用。
一、RE模块的基本功能
在导入re模块后,我们可以使用其提供的多种功能来进行正则表达式操作。以下是re模块中一些常用的函数及其用途:
re.match()
re.match()
函数用于从字符串的起始位置开始匹配。如果匹配成功,则返回一个Match对象,否则返回None。这意味着re.match()
只检查字符串的开头部分。
import re
pattern = r"hello"
string = "hello world"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
re.search()
与re.match()
不同,re.search()
会扫描整个字符串并返回第一个匹配的对象。即使模式不是在字符串的开始位置,re.search()
也会找到第一个符合条件的匹配。
import re
pattern = r"world"
string = "hello world"
search = re.search(pattern, string)
if search:
print("Match found:", search.group())
else:
print("No match")
re.findall()
re.findall()
会返回一个列表,包含所有与模式匹配的部分。即使没有匹配,返回的也是一个空列表。
import re
pattern = r"\d+"
string = "There are 2 apples and 5 oranges."
matches = re.findall(pattern, string)
print("Matches found:", matches)
re.sub()
re.sub()
用于替换字符串中的匹配项。它接收三个参数:模式、替换的字符串和要处理的字符串。
import re
pattern = r"apples"
replacement = "bananas"
string = "There are 2 apples and 5 oranges."
new_string = re.sub(pattern, replacement, string)
print("New string:", new_string)
二、正则表达式的语法
在使用re模块时,理解正则表达式的语法是至关重要的。以下是一些常用的正则表达式符号:
- 点号(.)
点号匹配除了换行符以外的任何字符。
import re
pattern = r"h.llo"
string = "hello"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
- 星号(*)
星号匹配前面的字符0次或多次。
import re
pattern = r"he.*o"
string = "heo"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
- 加号(+)
加号匹配前面的字符1次或多次。
import re
pattern = r"he.+o"
string = "heo"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
- 问号(?)
问号匹配前面的字符0次或1次。
import re
pattern = r"he.?o"
string = "heo"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
- 花括号({n,m})
花括号用于匹配前面的字符n到m次。
import re
pattern = r"he{1,2}o"
string = "heo"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
三、正则表达式中的分组与反向引用
在正则表达式中,可以使用圆括号()来创建分组。分组允许你在匹配中捕获子模式,并使用反向引用来重新使用这些子模式。
- 分组
分组通过圆括号()实现,可以捕获匹配的子字符串。
import re
pattern = r"(hello) (world)"
string = "hello world"
match = re.match(pattern, string)
if match:
print("Group 1:", match.group(1))
print("Group 2:", match.group(2))
else:
print("No match")
- 反向引用
反向引用允许在同一个正则表达式中引用之前定义的分组。反向引用的语法是\1
, \2
等,表示第一个、第二个分组。
import re
pattern = r"(hello) \1"
string = "hello hello"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
四、正则表达式的常用技巧
在处理正则表达式时,有一些常用的技巧可以帮助提高效率和准确性。
- 使用原始字符串
在Python中,正则表达式通常使用原始字符串(以r开头),这样可以避免转义字符带来的困扰。
- 非贪婪匹配
默认情况下,正则表达式是贪婪的,即尽可能多地匹配字符。可以使用?
来指定非贪婪匹配。
import re
pattern = r"<.*?>"
string = "<html><head></head></html>"
matches = re.findall(pattern, string)
print("Matches found:", matches)
- 使用标志
re模块提供了一些标志,可以修改匹配的行为,例如re.IGNORECASE
用于忽略大小写。
import re
pattern = r"hello"
string = "HELLO"
match = re.match(pattern, string, re.IGNORECASE)
if match:
print("Match found:", match.group())
else:
print("No match")
五、正则表达式的应用场景
正则表达式在实际应用中有广泛的用途,包括但不限于:
- 输入验证
可以使用正则表达式验证用户输入的格式是否正确,例如电子邮件地址、电话号码等。
- 数据提取
从文本中提取特定格式的数据,例如日期、时间、URL等。
- 文本替换
使用正则表达式进行复杂的文本替换操作,例如替换敏感信息、格式化文本等。
通过导入re模块并掌握正则表达式的基本语法和技巧,可以极大地提高字符串处理的效率和灵活性。无论是在数据清洗、文本分析还是网络爬虫中,正则表达式都是一项不可或缺的工具。
相关问答FAQs:
如何在Python中导入re模块?
要在Python中使用正则表达式功能,您需要导入re模块。可以通过在代码的开头添加import re
来完成导入。这一行代码将使您能够使用re模块提供的所有功能,比如搜索、匹配和替换字符串中的模式。
re模块中有哪些常用的函数?
re模块提供了多种函数来处理字符串。常用的有re.search()
用于查找字符串中是否包含某个模式,re.match()
用于从字符串的起始位置匹配模式,re.findall()
用于返回所有匹配的字符串列表,以及re.sub()
用于替换字符串中的指定模式。这些函数为文本处理和数据清洗提供了强大的工具。
在导入re模块后,我如何测试正则表达式?
导入re模块后,可以使用re模块的函数来测试正则表达式。您可以定义一个正则表达式并用它匹配一个字符串。例如,使用re.search(r'\d+', 'abc123')
可以查找字符串中是否有数字。如果存在,返回的结果将包含找到的匹配项的信息。通过这种方式,您可以轻松地验证您的正则表达式是否按预期工作。
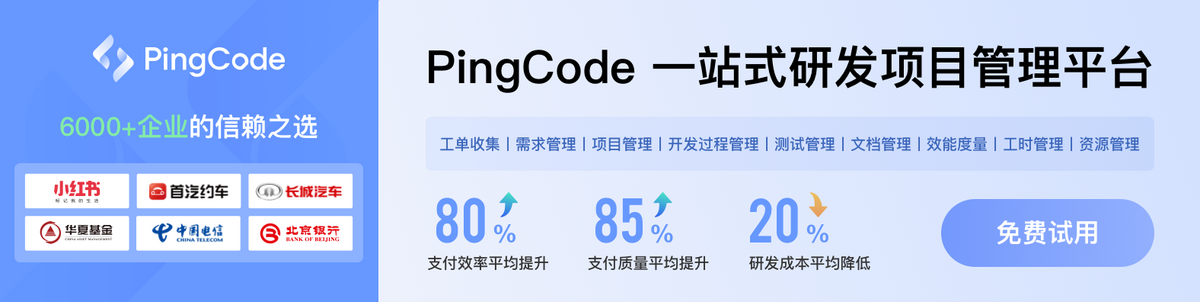