在Python中画多个菱形可以通过使用matplotlib、turtle库或其他图形处理库实现,主要步骤包括:确定菱形的顶点坐标、利用绘图库绘制菱形、调整菱形的布局。接下来,我们将详细介绍如何使用这些库来绘制多个菱形。
一、使用MATPLOTLIB绘制多个菱形
Matplotlib是Python中一个强大的绘图库,能够方便地绘制各种图形,包括菱形。我们可以通过定义菱形的顶点坐标,然后使用Polygon
对象来绘制。
-
定义菱形顶点
要绘制菱形,首先需要确定其顶点坐标。在二维平面中,一个标准的菱形可以用四个顶点坐标来定义。例如,假设菱形中心在坐标(0,0),那么顶点可以设定为(0, a)、(a, 0)、(0, -a)、(-a, 0),其中a是菱形的对角线一半长度。
-
使用Polygon绘制单个菱形
Matplotlib中的
Polygon
对象可以用于绘制多边形。使用Polygon
对象,我们可以通过传入顶点坐标列表来绘制菱形。import matplotlib.pyplot as plt
from matplotlib.patches import Polygon
def draw_rhombus(ax, center, a):
x, y = center
vertices = [(x, y + a), (x + a, y), (x, y - a), (x - a, y)]
rhombus = Polygon(vertices, closed=True, edgecolor='r', facecolor='none')
ax.add_patch(rhombus)
fig, ax = plt.subplots()
draw_rhombus(ax, (0, 0), 1)
ax.set_xlim(-2, 2)
ax.set_ylim(-2, 2)
plt.grid(True)
plt.show()
-
绘制多个菱形
可以通过循环或其他方式生成多个菱形的顶点坐标,然后在同一图中绘制。
fig, ax = plt.subplots()
centers = [(0, 0), (2, 2), (-2, -2), (2, -2), (-2, 2)]
for center in centers:
draw_rhombus(ax, center, 1)
ax.set_xlim(-4, 4)
ax.set_ylim(-4, 4)
plt.grid(True)
plt.show()
二、使用TURTLE绘制多个菱形
Turtle是Python内置的一个简单易用的绘图库,适合用于绘制简单图形。它以一个海龟为中心,通过移动海龟的位置来绘制图形。
-
初始化Turtle环境
首先,需要导入turtle库并初始化绘图窗口。
import turtle
screen = turtle.Screen()
screen.setup(width=600, height=600)
-
定义绘制单个菱形的函数
可以通过转动角度和前进一定距离来绘制菱形。
def draw_rhombus(t, size):
for _ in range(2):
t.forward(size)
t.left(60)
t.forward(size)
t.left(120)
t = turtle.Turtle()
t.penup()
-
绘制多个菱形
可以通过移动海龟到不同的位置来绘制多个菱形。
positions = [(0, 0), (100, 100), (-100, -100), (100, -100), (-100, 100)]
for position in positions:
t.goto(position)
t.pendown()
draw_rhombus(t, 50)
t.penup()
turtle.done()
三、使用其他库
除了Matplotlib和Turtle外,Python还有其他库可以用于绘制图形,如Pygame、Pillow等。每个库都有其独特的优点和用法,选择合适的库取决于具体需求。
-
Pygame
Pygame是一个用于开发游戏的库,支持丰富的图形绘制功能。可以通过在屏幕上绘制多边形来实现多个菱形的绘制。
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((600, 600))
screen.fill((255, 255, 255))
def draw_rhombus(surface, center, size, color):
x, y = center
vertices = [(x, y + size), (x + size, y), (x, y - size), (x - size, y)]
pygame.draw.polygon(surface, color, vertices, 1)
centers = [(300, 300), (400, 400), (200, 200), (400, 200), (200, 400)]
for center in centers:
draw_rhombus(screen, center, 50, (255, 0, 0))
pygame.display.flip()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
-
Pillow
Pillow是Python的图像处理库,可以用于生成和编辑图像。虽然不如Matplotlib和Pygame直接用于绘图,但可以生成图像文件。
from PIL import Image, ImageDraw
img = Image.new('RGB', (600, 600), (255, 255, 255))
draw = ImageDraw.Draw(img)
def draw_rhombus(draw, center, size):
x, y = center
vertices = [(x, y + size), (x + size, y), (x, y - size), (x - size, y)]
draw.polygon(vertices, outline=(255, 0, 0))
centers = [(300, 300), (400, 400), (200, 200), (400, 200), (200, 400)]
for center in centers:
draw_rhombus(draw, center, 50)
img.show()
img.save("rhombuses.png")
通过使用上述方法,可以在Python中实现多个菱形的绘制。选择合适的库和方法可以根据实际需求和偏好进行决定。无论是Matplotlib、Turtle、Pygame还是Pillow,都各有优势,可以帮助完成不同场景下的图形绘制任务。
相关问答FAQs:
如何在Python中绘制多个菱形?
要在Python中绘制多个菱形,可以使用Matplotlib库。你可以通过创建多个多边形对象来实现菱形的绘制。首先需要安装Matplotlib库,使用pip install matplotlib
进行安装。接着,可以使用plt.plot()
函数来绘制菱形,调整坐标以实现多个菱形的效果。
使用什么工具或库可以绘制菱形?
在Python中,最常用的绘图库是Matplotlib和Turtle。Matplotlib适合进行复杂的图形绘制和数据可视化,而Turtle则更适合初学者进行简单的图形绘制。如果你想要更直观和简单的绘图体验,可以选择Turtle库来绘制菱形。
如何自定义菱形的颜色和大小?
在Matplotlib中,可以通过设置多边形的facecolor
和edgecolor
参数来自定义菱形的颜色。菱形的大小可以通过调整坐标点的比例来实现。例如,可以使用plt.fill()
函数绘制填充颜色的菱形,并通过参数调整其大小。
在绘制多个菱形时,如何控制它们之间的间距?
为了控制多个菱形之间的间距,可以在绘制时调整每个菱形的坐标。例如,可以通过在x轴或y轴上增加固定的步长来实现菱形的间距。此外,使用循环结构可以在绘制多个菱形时更加灵活地控制它们的位置和间距。
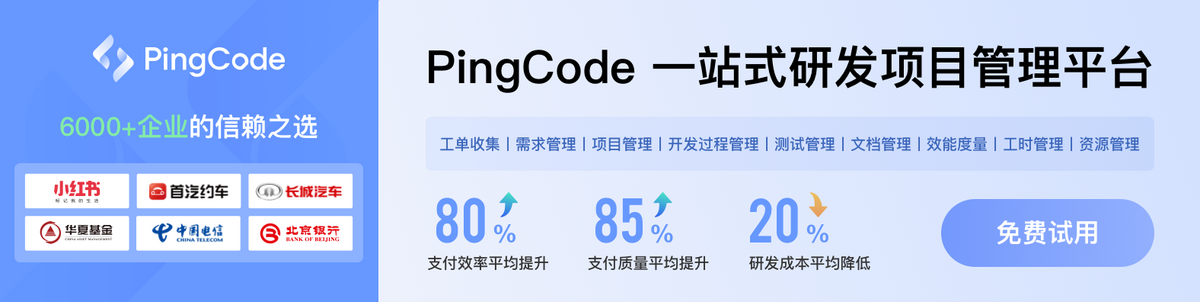