在Python中读取txt文件中的数字可以通过多种方法来实现,常用的方法包括使用内置的open函数、利用pandas库、使用numpy库等。以下将详细介绍其中一种方法,即使用Python内置的open函数读取txt文件中的数字。
使用Python内置的open函数读取txt文件中的数字是一种简单而有效的方法。首先,需要以读取模式打开文件,然后逐行读取文件内容,并将每一行中的数字提取出来。可以使用正则表达式库re来匹配数字,或者简单地使用字符串操作函数。以下是一个基本示例:
# 使用open函数读取txt文件中的数字
def read_numbers_from_file(file_path):
numbers = []
with open(file_path, 'r') as file:
for line in file:
# 使用字符串操作提取数字
line_numbers = [int(i) for i in line.split() if i.isdigit()]
numbers.extend(line_numbers)
return numbers
调用函数并打印结果
file_path = 'numbers.txt'
numbers = read_numbers_from_file(file_path)
print(numbers)
在这个示例中,我们定义了一个函数read_numbers_from_file
,用于读取文件并提取其中的数字。通过open
函数打开文件,并逐行读取内容。使用字符串的split
方法分割每一行的内容,并通过isdigit
方法检查每个单词是否为数字。如果是数字,就将其转换为整数并添加到列表中。
一、使用正则表达式读取数字
正则表达式提供了更为灵活的方式来匹配txt文件中的数字。Python的re
库可以方便地使用正则表达式进行字符串匹配和提取。
import re
def read_numbers_with_regex(file_path):
numbers = []
with open(file_path, 'r') as file:
for line in file:
# 使用正则表达式提取数字
line_numbers = re.findall(r'\d+', line)
numbers.extend(map(int, line_numbers))
return numbers
调用函数并打印结果
file_path = 'numbers.txt'
numbers = read_numbers_with_regex(file_path)
print(numbers)
在这个示例中,re.findall
函数用于查找每一行中的所有数字,并返回一个字符串列表。然后,使用map
函数将字符串列表转换为整数列表。
二、使用pandas读取数字
Pandas库是处理数据的强大工具,它能方便地读取和操作各种格式的数据,包括txt文件。对于每一行都遵循某种结构的txt文件,pandas的read_csv
函数可以发挥作用。
import pandas as pd
def read_numbers_with_pandas(file_path):
# 假设每行数据以空格分隔
df = pd.read_csv(file_path, sep=" ", header=None)
# 将所有数字存入一个列表
numbers = df.values.flatten().tolist()
return [int(num) for num in numbers if isinstance(num, (int, float))]
调用函数并打印结果
file_path = 'numbers.txt'
numbers = read_numbers_with_pandas(file_path)
print(numbers)
在这个示例中,我们使用pandas.read_csv
函数读取txt文件,并假设每行数据以空格分隔。df.values.flatten().tolist()
方法将DataFrame中的所有值展平为一个列表。
三、使用numpy读取数字
Numpy库专注于数值计算,它提供了一些强大的函数来读取和处理数据。对于结构化的数据文件,numpy.loadtxt
函数可以很好地完成任务。
import numpy as np
def read_numbers_with_numpy(file_path):
# 使用numpy读取数据
numbers = np.loadtxt(file_path)
return numbers.tolist()
调用函数并打印结果
file_path = 'numbers.txt'
numbers = read_numbers_with_numpy(file_path)
print(numbers)
在这个示例中,numpy.loadtxt
函数直接将txt文件中的数据读取为numpy数组,然后通过tolist
方法将其转换为Python列表。
四、处理不同格式的数字
在实际应用中,txt文件中的数字可能存在不同的格式,例如带有小数点、逗号分隔的数字等。需要根据具体情况调整读取方法。
- 读取小数:如果txt文件中包含小数,可以在提取数字时将字符串转换为浮点数。
def read_float_numbers(file_path):
numbers = []
with open(file_path, 'r') as file:
for line in file:
# 提取小数
line_numbers = re.findall(r'\d+\.\d+', line)
numbers.extend(map(float, line_numbers))
return numbers
- 处理带有逗号的数字:如果数字中包含逗号分隔符,需在读取时去掉逗号。
def read_comma_separated_numbers(file_path):
numbers = []
with open(file_path, 'r') as file:
for line in file:
# 去掉逗号并提取数字
line_numbers = [int(i.replace(',', '')) for i in re.findall(r'\d+', line)]
numbers.extend(line_numbers)
return numbers
五、读取大文件的优化
对于非常大的txt文件,逐行读取可能会占用大量内存。可以考虑使用生成器逐行处理文件内容,减少内存占用。
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield from (int(i) for i in line.split() if i.isdigit())
生成器使用示例
file_path = 'numbers.txt'
for number in read_large_file(file_path):
print(number)
使用生成器可以实现惰性读取,即每次只加载当前行到内存中,适合处理大文件。
六、总结
在Python中读取txt文件中的数字可以通过多种方法实现,具体选择哪种方法取决于文件的结构和数据格式。使用open函数和正则表达式是最基础的方法,适用于简单的数字提取。对于更复杂的结构化数据,pandas和numpy提供了强大的工具来读取和处理数据。在处理大文件时,使用生成器可以有效减少内存占用。根据实际需求和数据格式选择合适的方法,才能高效地从txt文件中读取数字。
相关问答FAQs:
如何在Python中打开和读取txt文件?
要在Python中打开和读取txt文件,可以使用内置的open
函数。使用with
语句可以确保文件在读取后被正确关闭。以下是一个基本的示例代码:
with open('文件名.txt', 'r') as file:
content = file.read()
print(content)
这样可以轻松地读取文件的全部内容。
如何将txt文件中的数字提取为列表?
在读取txt文件后,可以使用split()
方法将内容分割成单独的元素,并通过列表解析将其转换为数字。例如:
with open('文件名.txt', 'r') as file:
numbers = [int(num) for num in file.read().split()]
print(numbers)
此代码将txt文件中的所有数字提取到一个列表中。
如何处理txt文件中的非数字字符?
在读取文件并提取数字时,可能会遇到非数字字符。可以使用isdigit()
方法进行过滤。下面是一个示例:
with open('文件名.txt', 'r') as file:
numbers = [int(num) for num in file.read().split() if num.isdigit()]
print(numbers)
这样,只有文件中有效的数字会被提取到列表中。
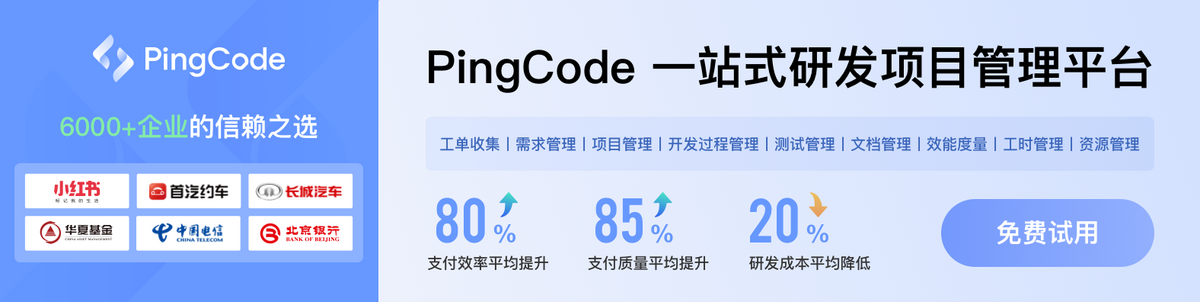