在Python中,想要让函数换行输出,可以使用换行符(\n)、多行字符串(""" """)、字符串连接和print函数结合使用。其中,最常用的方法是使用换行符(\n)。换行符可以轻松地在字符串中添加换行效果,使输出结果在不同的行显示。
详细描述:
换行符(\n)是一种特殊字符,代表换行。当你在字符串中使用它时,输出将自动在该位置换行。例如,在print函数中使用换行符,可以实现多行输出:
def example_function():
output = "Hello\nWorld\nPython"
print(output)
example_function()
在这个例子中,字符串中的\n
使得“Hello”、“World”和“Python”分别位于不同的行上输出。
一、使用换行符(\n)
使用换行符是最简单和直接的方法之一。将\n
插入到字符串中的适当位置,即可在输出时实现换行。
-
基本用法
在Python中,换行符
\n
可以直接用于字符串中,达到换行输出的效果。例如:def print_message():
message = "Hello, World!\nWelcome to Python programming."
print(message)
print_message()
在这个例子中,
Hello, World!
和Welcome to Python programming.
会被打印在不同的行上。 -
在循环中使用
当你需要在循环中输出多行时,也可以结合
\n
进行换行:def print_numbers():
numbers = ""
for i in range(5):
numbers += str(i) + "\n"
print(numbers)
print_numbers()
这种方法将在每次迭代后添加一个换行符,从而使数字在不同的行上输出。
二、使用多行字符串(""" """)
多行字符串是另一种实现换行输出的方法。使用三个引号("""
或'''
)可以创建一个多行字符串,Python会自动处理其中的换行。
-
基本用法
多行字符串可以直接用于定义包含多行文本的字符串:
def print_poem():
poem = """Roses are red,
Violets are blue,
Python is sweet,
And so are you."""
print(poem)
print_poem()
在这个例子中,诗歌的每一行都会在输出中自动换行。
-
结合函数使用
多行字符串可以轻松与函数结合使用,特别是在需要返回或打印多行文本时:
def get_multiline_text():
return """This is a multiline text.
It spans across several lines.
Each line is separate."""
print(get_multiline_text())
这种方式非常适合用于格式化和输出多行文本。
三、使用字符串连接
字符串连接可以用于动态生成多行字符串,通过组合多行字符串和换行符,实现灵活的输出格式。
-
基本用法
通过字符串的加法运算,可以将多个字符串拼接在一起:
def generate_multiline_message():
line1 = "This is line 1."
line2 = "This is line 2."
line3 = "This is line 3."
message = line1 + "\n" + line2 + "\n" + line3
print(message)
generate_multiline_message()
这种方法允许在运行时根据条件动态组合字符串。
-
在函数中应用
在函数中,可以根据输入参数或其他条件动态生成包含换行的输出:
def custom_message(name):
greeting = "Hello, " + name + "!\n"
body = "Welcome to the community.\n"
footer = "Enjoy your stay!"
message = greeting + body + footer
print(message)
custom_message("Alice")
这种灵活性使得字符串连接成为生成复杂输出的有效方法。
四、使用print函数的多个参数
在Python的print函数中,多个参数会自动以空格分隔输出,而不需要显式的换行符。
-
基本用法
使用print函数时,可以传递多个参数,每个参数都会被输出在同一行,并以空格分隔:
def print_with_spaces():
print("Hello", "world", "!")
print_with_spaces()
输出为
Hello world !
,但若需要换行,可以在参数中显式添加\n
:def print_with_newlines():
print("Hello\n", "world\n", "!")
print_with_newlines()
这样会产生每个单词在新行的输出。
-
结合end参数
print函数的
end
参数可以用于控制输出的结尾字符,默认是换行符\n
。通过修改end
参数,可以改变输出格式:def print_without_newline():
print("Hello", end=" ")
print("world", end="!")
print_without_newline()
在这个例子中,输出为
Hello world!
,而不是换行。
五、使用格式化字符串
格式化字符串允许在字符串中插入变量和表达式,并自动处理换行问题。
-
基本用法
使用f-string或str.format方法,可以轻松地创建多行字符串:
def formatted_output(name, age):
message = f"Name: {name}\nAge: {age}"
print(message)
formatted_output("Bob", 30)
在这个例子中,
{name}
和{age}
被替换为实际的变量值,并且每个变量占据一行。 -
复杂格式化
对于更复杂的格式化需求,可以使用多行格式化字符串:
def detailed_info(name, age, country):
info = (
f"Name: {name}\n"
f"Age: {age}\n"
f"Country: {country}"
)
print(info)
detailed_info("Alice", 28, "USA")
这种方法不仅简洁,还能保持良好的可读性。
六、使用Textwrap模块
Python的textwrap模块提供了对长字符串进行包装和格式化的功能,适合用于处理需要手动换行的长文本。
-
基本用法
textwrap模块的wrap函数可以用于将长字符串自动分割为多行:
import textwrap
def wrapped_text():
text = "Python is a high-level programming language, designed to be easy to read and simple to implement."
wrapped = textwrap.fill(text, width=50)
print(wrapped)
wrapped_text()
在这个例子中,长字符串被自动分成宽度为50的多行输出。
-
高级应用
对于更复杂的文本格式化,可以使用textwrap中的其他函数,如dedent和indent:
import textwrap
def formatted_paragraph():
paragraph = """
Python is an interpreted, high-level,
general-purpose programming language.
Its design philosophy emphasizes code readability.
"""
dedented_text = textwrap.dedent(paragraph)
print(dedented_text)
formatted_paragraph()
使用dedent可以清除多行字符串中的多余缩进,使其格式更美观。
总结来说,在Python中换行输出有多种方法可供选择:使用换行符、多行字符串、字符串连接、print函数的多个参数、格式化字符串以及textwrap模块。根据具体的应用场景和需求,可以选择最适合的方法来实现换行输出。每种方法都有其独特的优点和适用场景,了解它们的使用方式可以帮助你在编写Python代码时更加高效和灵活。
相关问答FAQs:
如何在Python函数中实现多行输出?
在Python中,可以使用三个引号('''或""")来定义多行字符串,这样可以轻松实现函数的多行输出。此外,也可以通过多次调用print()
函数来输出不同的内容。以下是一个示例:
def multi_line_output():
print("这是第一行")
print("这是第二行")
print("这是第三行")
Python中如何使用换行符进行输出?
换行符\n
可以在字符串中插入一个换行,使得输出更加清晰。只需在字符串中加入\n
,就可以实现换行。例如:
def output_with_newline():
print("第一行\n第二行\n第三行")
在Python中,如何格式化输出以便换行?
使用format()
方法或f-string可以在输出中插入换行符,允许在字符串中动态插入变量并保持格式美观。示例代码如下:
def formatted_output(name):
print(f"你好,{name}!\n欢迎来到Python编程世界。\n希望你能喜欢这里。")
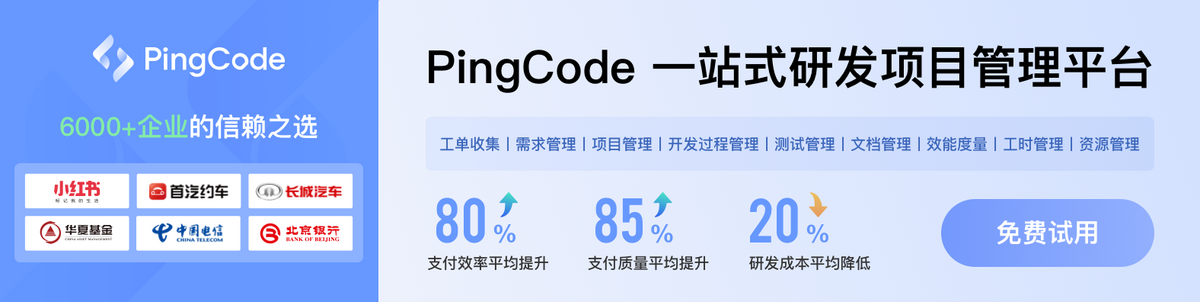