在Python中引入接口的主要方法是使用抽象基类(Abstract Base Classes, ABC)、通过模块化设计、使用协议和实现接口的设计模式。 其中,抽象基类是Python中定义接口的一种方式,允许定义方法的集合而不提供具体实现。通过模块化设计可以实现接口的分离,使代码更具可维护性。使用协议可以实现灵活的接口定义,而设计模式则提供了多种实现接口的方式。下面将详细介绍这些方法。
一、使用抽象基类(ABC)
抽象基类在Python中用于定义接口的标准方式。通过引入abc
模块,可以创建不能直接实例化的类,要求子类实现特定的方法。
-
定义抽象基类
在Python中,可以通过
abc
模块定义抽象基类。抽象基类定义了接口的基本结构和方法,但不提供具体实现。要定义一个抽象基类,需要继承自ABC
类,并使用@abstractmethod
装饰器标记抽象方法。from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def speak(self):
pass
在上面的例子中,
Animal
是一个抽象基类,其中包含一个抽象方法speak
。任何继承自Animal
的子类都必须实现speak
方法。 -
实现抽象基类
要实现抽象基类,子类需要继承该基类并实现所有的抽象方法。否则,子类也将是一个抽象类,不能被实例化。
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
在这个例子中,
Dog
和Cat
类继承了Animal
类,并实现了speak
方法。 -
使用抽象基类的好处
使用抽象基类可以确保子类提供特定的行为,同时允许为接口提供默认实现。此外,抽象基类提供了一种检查类型的机制,可以用来确保对象符合特定接口。
def animal_sound(animal: Animal):
print(animal.speak())
dog = Dog()
cat = Cat()
animal_sound(dog) # 输出: Woof!
animal_sound(cat) # 输出: Meow!
在这个例子中,
animal_sound
函数接受任何实现了Animal
接口的对象,并调用其speak
方法。
二、通过模块化设计
模块化设计是另一种实现接口的方法。通过将接口定义在单独的模块中,可以实现代码的分离和复用。
-
定义接口模块
可以在一个单独的模块中定义接口,然后在需要的地方进行导入。这样可以实现接口的分离和代码复用。
# interface.py
class AnimalInterface:
def speak(self):
raise NotImplementedError("Subclasses should implement this!")
-
实现接口
在其他模块中,可以通过继承接口类来实现接口。
# dog.py
from interface import AnimalInterface
class Dog(AnimalInterface):
def speak(self):
return "Woof!"
# cat.py
from interface import AnimalInterface
class Cat(AnimalInterface):
def speak(self):
return "Meow!"
-
模块化设计的优势
模块化设计可以实现代码的分离和复用。通过将接口定义在单独的模块中,可以在多个地方使用同一个接口定义。
# main.py
from dog import Dog
from cat import Cat
def animal_sound(animal):
print(animal.speak())
dog = Dog()
cat = Cat()
animal_sound(dog) # 输出: Woof!
animal_sound(cat) # 输出: Meow!
在这个例子中,
Dog
和Cat
类都实现了AnimalInterface
接口,并在main.py
中使用。
三、使用协议(Protocols)
Python 3.8引入了协议(Protocols),通过协议可以定义结构化的接口,而不需要显式的继承关系。协议是一种灵活的接口定义方式,允许在定义接口时不需要显式地继承某个类。
-
定义协议
协议是一种接口定义方式,可以通过
typing
模块中的Protocol
类定义。协议定义了一组方法和属性,任何实现这些方法和属性的类都被认为符合该协议。from typing import Protocol
class AnimalProtocol(Protocol):
def speak(self) -> str:
...
-
实现协议
要实现协议,只需要定义符合协议方法签名的类,而不需要显式地继承协议类。
class Dog:
def speak(self) -> str:
return "Woof!"
class Cat:
def speak(self) -> str:
return "Meow!"
-
使用协议
协议可以用于类型检查和接口验证,确保对象符合特定协议。
def animal_sound(animal: AnimalProtocol):
print(animal.speak())
dog = Dog()
cat = Cat()
animal_sound(dog) # 输出: Woof!
animal_sound(cat) # 输出: Meow!
在这个例子中,
animal_sound
函数接受任何符合AnimalProtocol
协议的对象,并调用其speak
方法。
四、设计模式中的接口实现
在软件设计中,设计模式提供了一种解决特定问题的通用方案。Python可以通过多种设计模式实现接口。
-
适配器模式
适配器模式用于将一个接口转换成另一个接口。它提供了一种方法,使接口不兼容的类能够协同工作。
class Dog:
def bark(self) -> str:
return "Woof!"
class DogAdapter:
def __init__(self, dog: Dog):
self._dog = dog
def speak(self) -> str:
return self._dog.bark()
在这个例子中,
DogAdapter
类将Dog
类的bark
方法适配为speak
方法。 -
装饰器模式
装饰器模式用于动态地给对象增加行为,而不会影响其他对象。它是一种灵活的接口实现方式。
class AnimalDecorator:
def __init__(self, animal):
self._animal = animal
def speak(self):
return self._animal.speak() + " (decorated)"
在这个例子中,
AnimalDecorator
类为实现接口的对象增加了新的行为。 -
策略模式
策略模式用于定义一系列算法,并将每个算法封装起来,使它们可以互换使用。策略模式可以用于实现多态接口。
class SpeakStrategy:
def speak(self):
pass
class DogSpeak(SpeakStrategy):
def speak(self):
return "Woof!"
class CatSpeak(SpeakStrategy):
def speak(self):
return "Meow!"
class AnimalContext:
def __init__(self, strategy: SpeakStrategy):
self._strategy = strategy
def perform_speak(self):
return self._strategy.speak()
在这个例子中,
AnimalContext
类可以使用不同的SpeakStrategy
实现多态接口。
五、总结
在Python中引入接口的方法多种多样,包括使用抽象基类、模块化设计、协议以及设计模式中的接口实现。这些方法各有优缺点,选择合适的方法取决于具体的应用场景和需求。通过合理使用这些方法,可以提高代码的可维护性和可扩展性,从而实现更加灵活的接口设计。
相关问答FAQs:
如何在Python中使用接口?
在Python中,接口通常是通过抽象基类(Abstract Base Classes, ABC)来实现的。您可以使用abc
模块来创建接口。定义一个接口时,您可以继承ABC
类,并使用@abstractmethod
装饰器来声明需要实现的方法。这样做能够确保任何实现该接口的类都必须提供这些方法的具体实现。
Python接口和类之间有什么区别?
接口主要用于定义一个类应该遵循的协议,而不提供具体的实现。类则可以包含具体的实现逻辑。通过接口,您可以确保不同的类遵循相同的结构,从而实现多态性。使用接口可以增强代码的可维护性和可扩展性。
在Python中如何检查一个类是否实现了特定接口?
可以使用isinstance()
函数来检查一个对象是否是某个类或接口的实例。如果您使用了抽象基类,可以直接检查该对象是否是某个抽象基类的子类或实现类。这样可以确保在运行时,您的对象确实实现了所需的接口。
可以给出一个简单的示例,说明如何在Python中定义和实现接口吗?
当然可以!以下是一个简单的示例:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
return "Woof!"
class Cat(Animal):
def sound(self):
return "Meow!"
# 测试实现
dog = Dog()
cat = Cat()
print(dog.sound()) # 输出: Woof!
print(cat.sound()) # 输出: Meow!
在这个示例中,Animal
是一个接口,Dog
和Cat
是实现了该接口的类。每个实现类都提供了sound
方法的具体实现。
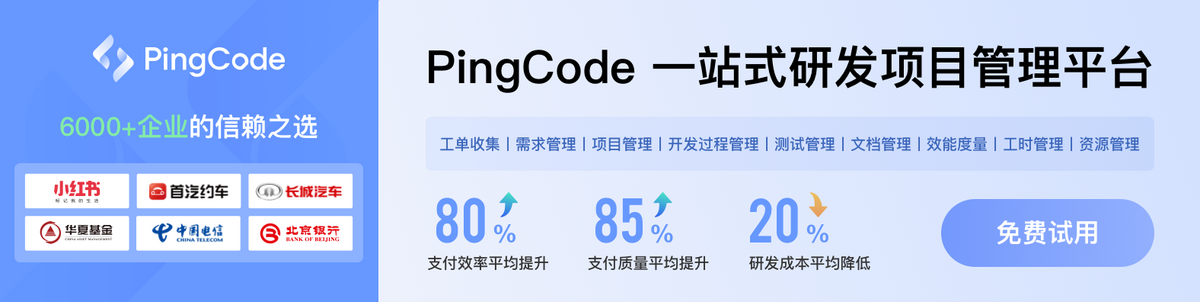