在Python中,求列表的乘积可以通过多种方式实现。常用的方法包括使用循环、reduce函数、numpy库。下面将详细介绍这几种方法,并对其中一种方法进行深入讲解。
一、使用循环
使用循环是最基础的方法之一,通过遍历列表中的每一个元素,将其乘积累积到一个变量中。
def list_product(lst):
product = 1
for num in lst:
product *= num
return product
示例
numbers = [1, 2, 3, 4, 5]
print(list_product(numbers)) # 输出:120
详细描述: 在这段代码中,我们定义了一个函数list_product
,它接受一个列表lst
作为参数。我们首先初始化一个变量product
为1,因为乘法的单位元是1。然后,我们使用一个for
循环遍历列表中的每一个元素num
,并将其与product
相乘,最后返回product
的值。
二、使用reduce函数
Python的functools
模块提供了reduce
函数,它可以用于对列表元素进行累计操作。
from functools import reduce
def list_product(lst):
return reduce(lambda x, y: x * y, lst)
示例
numbers = [1, 2, 3, 4, 5]
print(list_product(numbers)) # 输出:120
在这里,reduce
函数接受一个二元函数和一个列表作为参数。lambda x, y: x * y
是一个匿名函数,用于将列表中的两个元素相乘。reduce
会对列表中的元素进行累计操作,从而得到最终的乘积。
三、使用numpy库
numpy
是Python中一个强大的科学计算库,它提供了高效的数组操作。
import numpy as np
def list_product(lst):
return np.prod(lst)
示例
numbers = [1, 2, 3, 4, 5]
print(list_product(numbers)) # 输出:120
numpy
中的prod
函数可以直接对数组或列表中的元素进行乘积操作,且由于numpy
的底层实现是用C语言编写的,因此在处理大规模数据时效率更高。
四、递归实现
递归是一种编程技巧,通过函数自身调用来实现复杂问题的解决。虽然在求乘积问题中递归并不是最优解,但它提供了一种不同的思维方式。
def list_product(lst):
if len(lst) == 0:
return 1
else:
return lst[0] * list_product(lst[1:])
示例
numbers = [1, 2, 3, 4, 5]
print(list_product(numbers)) # 输出:120
在这段代码中,我们定义了一个递归函数list_product
。如果列表为空,函数返回1;否则,函数返回列表第一个元素与剩余元素乘积的结果。
五、性能比较
对于小规模的数据集,使用循环、reduce
、numpy
等方法的性能差异并不明显。然而,在处理大规模数据时,numpy
通常表现得更为优越,因为它在底层进行了优化。
进行性能比较可以通过以下代码实现:
import time
def performance_test():
numbers = list(range(1, 10001)) # 一个包含10000个数字的列表
start_time = time.time()
list_product(numbers)
print("Loop method time:", time.time() - start_time)
start_time = time.time()
reduce(lambda x, y: x * y, numbers)
print("Reduce method time:", time.time() - start_time)
start_time = time.time()
np.prod(numbers)
print("Numpy method time:", time.time() - start_time)
performance_test()
通过性能测试可以发现,numpy
方法在处理大规模数据时通常更快。这是因为numpy
利用了底层的C语言实现,并进行了向量化操作,使得运算速度大幅提高。
六、错误处理
在编写函数时,我们也需要考虑输入的合法性。例如,列表中可能包含非数字元素或为空,这些情况都需要进行处理。
def list_product_safe(lst):
if not all(isinstance(x, (int, float)) for x in lst):
raise ValueError("List must contain only numbers.")
if len(lst) == 0:
return 1
return np.prod(lst)
示例
try:
numbers = [1, 2, 'a', 4, 5]
print(list_product_safe(numbers))
except ValueError as e:
print(e) # 输出:List must contain only numbers.
在这段代码中,我们使用isinstance
函数判断列表中的每一个元素是否为数字类型。如果列表中有非数字元素,函数将抛出一个ValueError
异常。此外,我们还处理了列表为空的情况,返回1作为默认值。
七、总结
在Python中,求列表乘积的方法多种多样。最简单的方法是使用循环,但对于大规模数据集,numpy
提供了更高效的解决方案。此外,通过使用reduce
函数,我们可以更清晰地表达累计操作。编写函数时,还应考虑输入的合法性和可能的异常情况,以提高代码的鲁棒性。在选择具体方法时,应根据数据规模和性能需求做出合理的选择。
相关问答FAQs:
如何在Python中计算列表的乘积?
在Python中,可以使用reduce
函数结合operator.mul
来计算列表的乘积。首先,需要导入reduce
和operator
模块。示例代码如下:
from functools import reduce
import operator
my_list = [1, 2, 3, 4]
product = reduce(operator.mul, my_list, 1)
print(product) # 输出24
这种方法适用于任何数字列表,确保计算乘积的同时也能够处理空列表的情况。
是否可以使用循环来计算列表的乘积?
当然可以!通过简单的for循环,可以遍历列表并逐步计算乘积。以下是一个示例:
my_list = [1, 2, 3, 4]
product = 1
for num in my_list:
product *= num
print(product) # 输出24
这种方法易于理解,非常适合初学者。
使用NumPy库计算列表的乘积有什么优势?
NumPy库提供了高效的数组操作,可以快速计算乘积。使用numpy.prod()
函数,不仅简洁而且性能优越,尤其是在处理大规模数据时。示例如下:
import numpy as np
my_list = [1, 2, 3, 4]
product = np.prod(my_list)
print(product) # 输出24
使用NumPy时,确保已经安装了该库,可以通过pip install numpy
来进行安装。
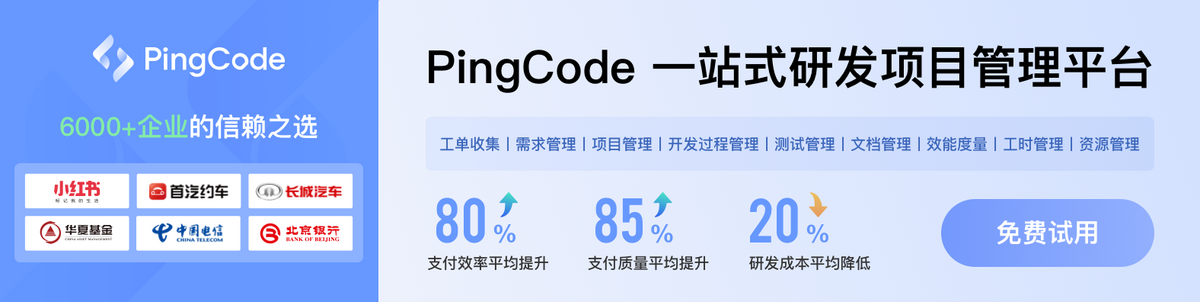