Python请求接口地址的方法有:使用requests库、使用http.client模块、使用urllib库。其中,requests库是最常用且简单的方法。它提供了丰富的功能来处理HTTP请求,使得与网络交互变得更加便捷。下面将详细介绍如何使用requests库来请求接口地址,并探讨其他方法的优缺点。
一、REQUESTS库的使用
requests库是Python中用于发送HTTP请求的强大工具。它的设计目的是让HTTP请求变得非常简单和直观。以下是如何使用requests库请求接口地址的详细步骤。
- 安装和导入requests库
首先,确保您的Python环境中已经安装了requests库。如果没有安装,可以使用以下命令进行安装:
pip install requests
安装完成后,在您的Python脚本中导入requests库:
import requests
- 发送GET请求
GET请求用于从服务器获取数据。要发送GET请求,只需调用requests.get()方法,并传递目标URL。例如:
response = requests.get('https://api.example.com/data')
在这个例子中,https://api.example.com/data
是目标接口地址。response
对象包含了服务器的响应数据。
- 处理响应数据
response
对象提供了多种方法来访问服务器的响应数据。常用的方法包括:
response.text
:以字符串形式获取响应体。response.json()
:将响应体解析为JSON格式(前提是响应是JSON格式)。response.status_code
:获取HTTP状态码。response.headers
:获取响应头信息。
例如:
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Request failed with status code {response.status_code}")
- 发送POST请求
POST请求用于向服务器发送数据。要发送POST请求,使用requests.post()方法,并传递数据。例如:
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/post', data=payload)
- 请求头和参数
在发送请求时,您可能需要设置请求头或添加查询参数。可以通过headers
和params
参数实现:
headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}
params = {'param1': 'value1', 'param2': 'value2'}
response = requests.get('https://api.example.com/data', headers=headers, params=params)
二、HTTP.CLIENT模块的使用
http.client模块是Python的标准库之一,提供了与HTTP服务器进行交互的底层接口。虽然不如requests库直观,但在某些情况下,它可以提供更细粒度的控制。
- 创建连接
要使用http.client模块,首先需要创建一个HTTP连接:
import http.client
connection = http.client.HTTPSConnection('api.example.com')
- 发送请求
使用connection对象的request()方法发送请求:
connection.request('GET', '/data')
response = connection.getresponse()
- 处理响应
使用response对象的方法获取响应数据:
if response.status == 200:
response_data = response.read()
print(response_data.decode('utf-8'))
else:
print(f"Request failed with status code {response.status}")
- 关闭连接
使用完连接后,确保关闭连接:
connection.close()
三、URLLIB库的使用
urllib是Python的标准库,提供了一组用于处理URL的模块。与requests库相比,urllib的API稍显复杂,但在处理简单请求时足够实用。
- 导入urllib库
import urllib.request
- 发送GET请求
使用urlopen()方法发送GET请求:
response = urllib.request.urlopen('https://api.example.com/data')
response_data = response.read()
- 处理响应
print(response_data.decode('utf-8'))
- 发送POST请求
要发送POST请求,需要创建请求对象,并指定数据:
data = urllib.parse.urlencode({'key1': 'value1', 'key2': 'value2'}).encode('utf-8')
request = urllib.request.Request('https://api.example.com/post', data=data)
response = urllib.request.urlopen(request)
- 添加请求头
可以使用add_header()方法为请求对象添加请求头:
request.add_header('Authorization', 'Bearer YOUR_ACCESS_TOKEN')
四、选择合适的方法
在选择请求接口的方法时,应该考虑以下因素:
- 复杂度和易用性:requests库最为简单易用,非常适合快速开发和调试。
- 灵活性和控制:http.client模块提供了更底层的控制,适合需要自定义复杂请求的场景。
- 兼容性和依赖:urllib是Python的内置库,不需要额外安装,适合对外部依赖有严格限制的环境。
综上所述,在大多数情况下,建议使用requests库进行接口请求。它提供了简单且强大的API,能够满足绝大多数的HTTP请求需求。同时,了解http.client和urllib的使用也可以帮助您在某些特定场景下更好地处理HTTP请求。
相关问答FAQs:
如何使用Python发送GET请求?
使用Python发送GET请求可以通过内置的requests
库来实现。首先,确保已经安装了requests
库。可以使用命令pip install requests
进行安装。示例代码如下:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code) # 打印响应状态码
print(response.json()) # 打印响应内容
如何在Python中发送POST请求?
发送POST请求同样可以使用requests
库。POST请求通常用于提交数据到服务器。以下是一个简单的示例,展示如何发送JSON数据:
import requests
url = 'https://api.example.com/submit'
data = {'key': 'value'}
response = requests.post(url, json=data)
print(response.status_code) # 打印响应状态码
print(response.json()) # 打印响应内容
如何处理Python中的接口请求异常?
在进行接口请求时,可能会遇到各种异常情况,例如网络连接失败或请求超时。通过使用try-except
结构,可以有效地处理这些异常。示例代码如下:
import requests
try:
response = requests.get('https://api.example.com/data', timeout=5)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.HTTPError as err:
print(f'HTTP错误: {err}')
except requests.exceptions.ConnectionError:
print('连接错误,请检查网络连接。')
except requests.exceptions.Timeout:
print('请求超时,请重试。')
except Exception as err:
print(f'发生了其他错误: {err}')
通过以上示例,可以有效地进行接口请求和异常处理,确保程序的健壮性。
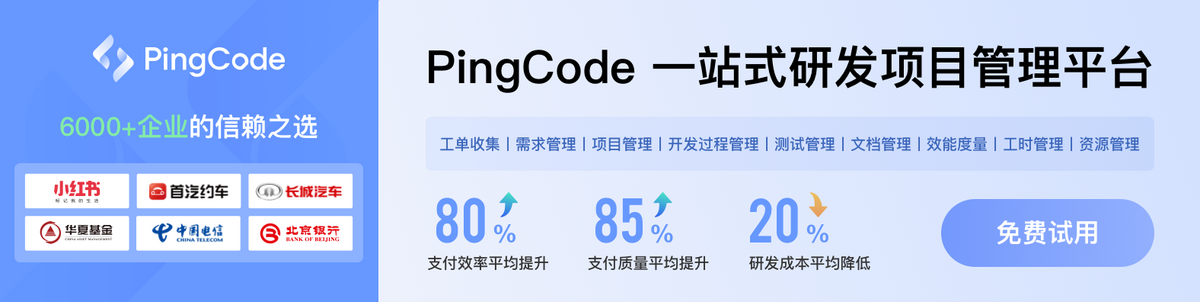