利用Python显示时间的方法包括使用time模块、datetime模块、strftime函数、以及pytz库来处理时区。在这些方法中,datetime
模块是最常用的,它提供了日期和时间的操作功能。我们可以使用datetime.now()
来获取当前的日期和时间,并通过strftime
格式化输出。接下来,我们将详细探讨如何使用这些工具来显示和操作时间。
一、TIME模块
time
模块是Python中处理时间最基础的模块之一。它提供了访问时间和日期的多种方法。
-
获取当前时间戳
时间戳是一个浮点数,表示自1970年1月1日以来的秒数。使用
time.time()
可以获取当前的时间戳。import time
current_timestamp = time.time()
print("Current Timestamp:", current_timestamp)
这种方法适用于需要进行时间戳比较或者简单计算的场景。
-
格式化时间
time
模块提供了time.strftime()
函数,可以将时间戳转换为可读的字符串格式。import time
local_time = time.localtime()
formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", local_time)
print("Formatted Time:", formatted_time)
这种方法适用于需要将时间戳转换为易读格式展示的场景。
二、DATETIME模块
datetime
模块是Python中处理日期和时间的标准模块。它提供了类和方法来操作日期和时间。
-
获取当前日期和时间
使用
datetime.now()
可以获取当前的日期和时间。from datetime import datetime
current_datetime = datetime.now()
print("Current Date and Time:", current_datetime)
这种方法适用于需要获取当前时间并进行进一步处理的场景。
-
格式化日期和时间
datetime
对象可以通过strftime()
方法转换为格式化字符串。from datetime import datetime
current_datetime = datetime.now()
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
print("Formatted Date and Time:", formatted_datetime)
这种方法适用于需要以特定格式展示日期和时间的场景。
三、STRFTIME函数
strftime()
函数用于将时间对象格式化为可读字符串。它支持多种格式化指令,用于指定输出格式。
-
常用格式化指令
%Y
:四位数的年份%m
:两位数的月份%d
:两位数的日期%H
:两位数的小时(24小时制)%M
:两位数的分钟%S
:两位数的秒
示例:
from datetime import datetime
current_datetime = datetime.now()
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
print("Formatted Date and Time:", formatted_datetime)
-
自定义格式
可以根据需要自定义日期和时间的格式。例如,将日期和时间格式化为“Day-Month-Year Hour:Minute:Second”格式:
from datetime import datetime
current_datetime = datetime.now()
formatted_datetime = current_datetime.strftime("%d-%m-%Y %H:%M:%S")
print("Custom Formatted Date and Time:", formatted_datetime)
四、PYTZ库
pytz
库用于处理时区问题。它提供了世界各地的时区信息,并可以将时间转换为指定时区。
-
安装pytz
使用
pip
安装pytz
库:pip install pytz
-
使用pytz设置时区
使用
pytz
库可以将时间转换为不同的时区。from datetime import datetime
import pytz
获取当前时间
current_datetime = datetime.now()
设置时区为UTC
utc_timezone = pytz.utc
utc_time = current_datetime.astimezone(utc_timezone)
print("UTC Time:", utc_time)
设置时区为纽约
ny_timezone = pytz.timezone('America/New_York')
ny_time = current_datetime.astimezone(ny_timezone)
print("New York Time:", ny_time)
这种方法适用于需要根据不同地区展示时间的场景。
五、日期和时间的加减操作
datetime
模块还支持日期和时间的加减操作,这在需要进行时间计算时非常有用。
-
加减天数
使用
timedelta
对象可以对日期和时间进行加减操作。from datetime import datetime, timedelta
current_datetime = datetime.now()
print("Current Date and Time:", current_datetime)
加3天
future_date = current_datetime + timedelta(days=3)
print("Future Date (after 3 days):", future_date)
减5天
past_date = current_datetime - timedelta(days=5)
print("Past Date (5 days ago):", past_date)
-
加减小时和分钟
同样,可以通过
timedelta
对象加减小时和分钟。from datetime import datetime, timedelta
current_datetime = datetime.now()
加2小时
future_time = current_datetime + timedelta(hours=2)
print("Future Time (after 2 hours):", future_time)
减30分钟
past_time = current_datetime - timedelta(minutes=30)
print("Past Time (30 minutes ago):", past_time)
六、总结
通过以上的方法,我们可以在Python中有效地获取和显示时间。无论是简单的时间戳获取,还是复杂的时区转换,Python都提供了强大的工具来满足我们的需求。datetime
模块因其丰富的功能和简单的接口,是处理时间和日期的首选。在实际应用中,根据具体需求选择合适的方法,以便更好地处理和展示时间信息。
相关问答FAQs:
如何在Python中获取当前时间和日期?
在Python中,可以使用内置的datetime
模块来获取当前的时间和日期。你可以通过以下代码获取当前时间:
import datetime
now = datetime.datetime.now()
print(now)
这将显示当前的日期和时间,格式为YYYY-MM-DD HH:MM:SS.ssssss
。如果只需要日期或时间,可以分别使用now.date()
和now.time()
来提取。
如何格式化显示Python中的时间和日期?
使用strftime()
方法可以对时间和日期进行格式化,以便以更易读的方式显示。例如,下面的代码可以将当前时间格式化为YYYY-MM-DD HH:MM:SS
格式:
formatted_time = now.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_time)
通过更改格式字符串,可以实现各种显示效果,满足不同需求。
如何在Python中实现定时功能?
如果你想要在Python中创建一个定时器,可以使用time
模块的sleep()
函数。例如,下面的代码将在每隔5秒后显示当前时间:
import time
while True:
print(datetime.datetime.now())
time.sleep(5)
这种方式适合用于需要定时执行的任务,比如定时记录数据或定期检查某个条件。
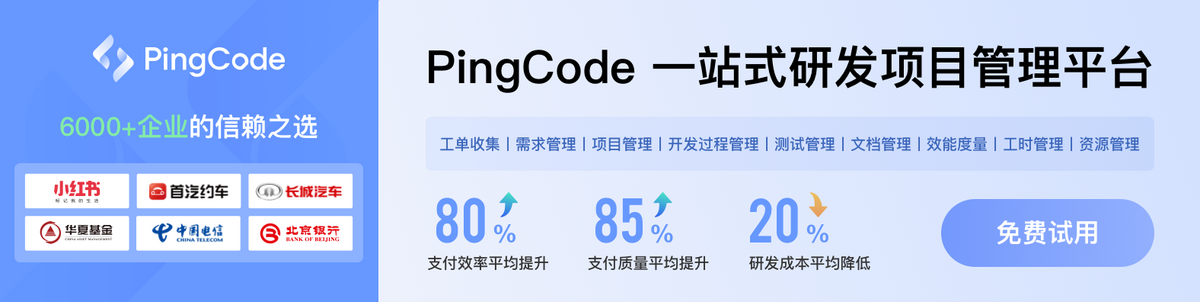