在Python中,实现装饰器的方法包括:定义一个装饰器函数、使用@
符号应用装饰器、处理带参数的装饰器、链式装饰器。装饰器本质上是一个高阶函数,能够在不修改原始函数代码的情况下,扩展或改变函数的行为。下面,我将详细介绍如何在Python中实现装饰器。
一、定义装饰器函数
装饰器本质上是一个接受函数作为参数并返回一个新函数的函数。在Python中,装饰器通常用来在函数执行前后添加额外的行为。
def my_decorator(func):
def wrapper():
print("Before function execution")
result = func()
print("After function execution")
return result
return wrapper
在这个示例中,my_decorator
是一个简单的装饰器,它在被装饰的函数执行之前和之后打印信息。
二、使用@
符号应用装饰器
Python提供了@
语法糖来更方便地应用装饰器。这种语法糖可以让代码更加简洁和易读。
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个示例中,say_hello
函数被my_decorator
装饰器装饰。当调用say_hello
时,实际上执行的是wrapper
函数。
三、处理带参数的装饰器
当被装饰的函数需要接受参数时,装饰器需要进行相应的调整,以确保参数能够正确传递。
def my_decorator(func):
def wrapper(*args, kwargs):
print("Before function execution")
result = func(*args, kwargs)
print("After function execution")
return result
return wrapper
@my_decorator
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
在这个示例中,wrapper
函数使用*args
和kwargs
来接受任意数量的参数,从而确保被装饰的函数可以接受任何数量的参数。
四、链式装饰器
Python允许使用多个装饰器装饰同一个函数,这称为链式装饰器。被装饰的函数将依次经过每个装饰器的处理。
def decorator_one(func):
def wrapper(*args, kwargs):
print("Decorator One")
return func(*args, kwargs)
return wrapper
def decorator_two(func):
def wrapper(*args, kwargs):
print("Decorator Two")
return func(*args, kwargs)
return wrapper
@decorator_one
@decorator_two
def say_goodbye():
print("Goodbye!")
say_goodbye()
在这个示例中,say_goodbye
函数首先被decorator_two
装饰,然后被decorator_one
装饰。执行顺序是从下到上。
五、使用类实现装饰器
装饰器不仅可以用函数实现,也可以用类实现。这种方法可以在装饰器中维护状态。
class MyDecorator:
def __init__(self, func):
self.func = func
def __call__(self, *args, kwargs):
print("Before function execution")
result = self.func(*args, kwargs)
print("After function execution")
return result
@MyDecorator
def greet(name):
print(f"Hello, {name}!")
greet("Bob")
在这个示例中,MyDecorator
类实现了__call__
方法,因此可以像函数一样调用。类装饰器的优点在于能够在实例中保存状态或数据。
六、装饰器的实际应用场景
1、日志记录
装饰器常用于记录函数的调用日志,包括函数名、参数、返回值和执行时间等信息。
import time
def log_execution_time(func):
def wrapper(*args, kwargs):
start_time = time.time()
result = func(*args, kwargs)
end_time = time.time()
print(f"Function {func.__name__} executed in {end_time - start_time:.4f} seconds")
return result
return wrapper
@log_execution_time
def compute():
for _ in range(1000000):
pass
compute()
2、权限校验
在Web应用中,装饰器常用于验证用户是否有权限执行某个操作。
def check_permission(permission):
def decorator(func):
def wrapper(*args, kwargs):
user_permission = "user"
if user_permission != permission:
print("Permission denied")
return
return func(*args, kwargs)
return wrapper
return decorator
@check_permission("admin")
def delete_user():
print("User deleted")
delete_user()
3、缓存结果
装饰器可以用于缓存函数的计算结果,以提高性能。
def cache(func):
cached_results = {}
def wrapper(*args):
if args in cached_results:
return cached_results[args]
result = func(*args)
cached_results[args] = result
return result
return wrapper
@cache
def fibonacci(n):
if n in (0, 1):
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(35))
七、装饰器的注意事项
1、维护函数元数据
使用装饰器时,原始函数的元数据(如函数名、文档字符串)可能会丢失。为了解决这个问题,可以使用functools.wraps
装饰wrapper
函数。
import functools
def my_decorator(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
print("Before function execution")
result = func(*args, kwargs)
print("After function execution")
return result
return wrapper
2、装饰器的顺序
当使用多个装饰器时,装饰器的应用顺序是从下到上。因此,要注意装饰器的顺序可能会影响最终的行为。
八、总结
装饰器是Python中一个强大而灵活的特性,可以用来增强和修改函数的行为。通过理解装饰器的基本原理和实现方法,可以在实际开发中更好地利用这一特性,提高代码的可复用性和可维护性。装饰器的应用场景广泛,包括日志记录、权限校验、结果缓存等。通过结合不同的装饰器,可以实现复杂的功能,同时保持代码的简洁和清晰。
相关问答FAQs:
装饰器在Python中的作用是什么?
装饰器是Python中的一种设计模式,允许在不修改函数代码的前提下,向函数添加额外的功能。它们通常用于日志记录、权限检查、缓存等场景。通过使用装饰器,您可以轻松地增强或修改函数的行为,提升代码的复用性和可读性。
如何定义一个简单的装饰器?
定义一个简单的装饰器通常涉及创建一个嵌套函数。外层函数接收被装饰的函数作为参数,内层函数实现增强功能,最后返回内层函数。以下是一个基本示例:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个例子中,say_hello
函数被my_decorator
装饰,调用say_hello
时会同时执行装饰器中的代码。
装饰器可以接受参数吗?
是的,装饰器可以接收参数。这种情况下,需要再嵌套一层函数。外层函数接受装饰器参数,内层函数接收被装饰的函数。以下是一个示例:
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(num_times=3)
def say_hello(name):
print(f"Hello, {name}!")
say_hello("Alice")
这个装饰器会在调用say_hello
时重复打印问候语指定的次数。
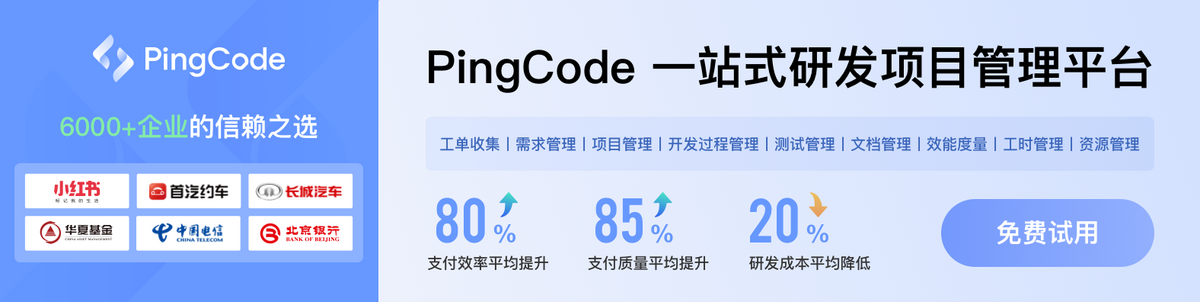