要用Python实现CMD(命令提示符),可以使用subprocess模块、os模块、cmd模块。通过subprocess模块,你可以运行外部命令并获取其输出;os模块提供与操作系统交互的功能;cmd模块则用于创建命令行界面。本文将详细探讨如何利用这些工具实现一个简单的CMD功能,并在此基础上进行扩展。
一、SUBPROCESS模块
subprocess模块是Python中用于生成子进程并与其进行交互的最常用模块之一。它提供了多种方法来启动和管理子进程。
1.1 生成子进程
subprocess模块的核心功能是通过调用subprocess.run()
函数生成子进程。这个函数可以执行一个命令并等待其完成。下面是一个简单的示例:
import subprocess
def run_command(command):
result = subprocess.run(command, shell=True, capture_output=True, text=True)
return result.stdout
output = run_command("echo Hello, World!")
print(output)
在这个示例中,run_command()
函数接收一个命令字符串,使用subprocess.run()
执行该命令并返回其输出。capture_output=True
参数用于捕获命令的标准输出,text=True
则将输出解码为字符串。
1.2 处理命令的输出和错误
subprocess模块还提供了其他功能来处理命令输出和错误。例如,你可以使用stderr
参数捕获标准错误输出:
import subprocess
def run_command(command):
result = subprocess.run(command, shell=True, capture_output=True, text=True)
if result.returncode == 0:
return result.stdout
else:
return result.stderr
output = run_command("ls /nonexistent_directory")
print(output)
在这个示例中,run_command()
函数不仅捕获标准输出,还检查命令的返回码。如果命令执行失败,返回标准错误输出。
1.3 实现交互式命令行
subprocess模块还可以用于创建一个简单的交互式命令行工具:
import subprocess
def interactive_shell():
while True:
command = input("cmd> ")
if command.lower() in ['exit', 'quit']:
break
result = subprocess.run(command, shell=True, capture_output=True, text=True)
print(result.stdout)
interactive_shell()
在这个示例中,interactive_shell()
函数提供一个命令行提示符,允许用户输入命令并查看其输出。输入exit
或quit
将退出循环。
二、OS模块
os模块提供了与操作系统进行交互的功能,例如文件和目录操作、环境变量管理等。
2.1 执行系统命令
os模块的system()
函数可以用于执行简单的系统命令:
import os
def run_command(command):
os.system(command)
run_command("echo Hello, World!")
然而,os.system()
的功能有限,它只执行命令而不捕获其输出。因此,如果你需要获取命令输出,subprocess模块通常是更好的选择。
2.2 文件和目录操作
os模块还提供了一些用于文件和目录操作的函数,这些函数可以与CMD工具结合使用:
import os
def list_directory(path):
with os.scandir(path) as entries:
for entry in entries:
print(entry.name)
list_directory(".")
在这个示例中,list_directory()
函数使用os.scandir()
列出指定目录中的所有文件和子目录。
2.3 管理环境变量
os模块允许你获取和设置环境变量,这在某些情况下可能非常有用:
import os
def print_environment_variable(var_name):
value = os.getenv(var_name)
print(f"{var_name}={value}")
print_environment_variable("PATH")
os.getenv()
函数返回指定环境变量的值。你也可以使用os.environ
字典直接访问和修改环境变量。
三、CMD模块
cmd模块是Python标准库中的一个模块,用于创建命令行接口。它提供了一个基类Cmd
,你可以继承该类并实现自定义命令。
3.1 创建简单的命令行工具
通过继承cmd.Cmd
类,你可以创建一个简单的命令行工具:
import cmd
class MyCmd(cmd.Cmd):
prompt = 'cmd> '
def do_greet(self, line):
print("Hello!")
def do_exit(self, line):
print("Goodbye!")
return True
if __name__ == '__main__':
MyCmd().cmdloop()
在这个示例中,MyCmd
类定义了两个命令:greet
和exit
。do_greet()
方法在用户输入greet
时执行,do_exit()
方法在用户输入exit
时执行并退出命令循环。
3.2 添加更多命令
你可以通过在MyCmd
类中定义更多的方法来扩展命令行工具的功能。例如:
import cmd
class MyCmd(cmd.Cmd):
prompt = 'cmd> '
def do_greet(self, line):
print("Hello!")
def do_exit(self, line):
print("Goodbye!")
return True
def do_echo(self, line):
print(line)
if __name__ == '__main__':
MyCmd().cmdloop()
在这个示例中,do_echo()
方法接收用户输入的参数并将其打印出来。
3.3 实现帮助功能
cmd模块还提供了一个内置的帮助系统。你可以为每个命令定义一个帮助字符串:
import cmd
class MyCmd(cmd.Cmd):
prompt = 'cmd> '
def do_greet(self, line):
"""Greet the user."""
print("Hello!")
def do_exit(self, line):
"""Exit the command line interface."""
print("Goodbye!")
return True
def do_echo(self, line):
"""Echo the input back to the user."""
print(line)
if __name__ == '__main__':
MyCmd().cmdloop()
在这个示例中,do_greet()
、do_exit()
和do_echo()
方法都有一个帮助字符串。当用户输入help
命令时,cmd模块将显示这些帮助信息。
四、综合示例:使用Python实现一个简单的CMD工具
通过结合subprocess、os和cmd模块的功能,我们可以实现一个更复杂的CMD工具。
import cmd
import subprocess
import os
class SimpleCmd(cmd.Cmd):
prompt = 'cmd> '
def do_run(self, line):
"""Run a system command."""
try:
result = subprocess.run(line, shell=True, capture_output=True, text=True)
print(result.stdout)
except Exception as e:
print(f"Error: {e}")
def do_list(self, line):
"""List files in a directory."""
path = line if line else '.'
try:
with os.scandir(path) as entries:
for entry in entries:
print(entry.name)
except FileNotFoundError:
print(f"Directory not found: {path}")
def do_setenv(self, line):
"""Set an environment variable."""
try:
var, value = line.split('=')
os.environ[var.strip()] = value.strip()
print(f"Environment variable {var.strip()} set to {value.strip()}")
except ValueError:
print("Usage: setenv VAR=VALUE")
def do_getenv(self, line):
"""Get an environment variable."""
value = os.getenv(line.strip())
if value:
print(f"{line.strip()}={value}")
else:
print(f"Environment variable not found: {line.strip()}")
def do_exit(self, line):
"""Exit the command line interface."""
print("Goodbye!")
return True
if __name__ == '__main__':
SimpleCmd().cmdloop()
在这个示例中,SimpleCmd
类实现了多个命令,包括运行系统命令、列出目录内容、设置和获取环境变量。通过这种方式,你可以创建一个功能强大的CMD工具,适用于各种任务和自动化需求。
总结
Python提供了多个模块来实现CMD工具。subprocess模块适用于运行外部命令并处理其输出,os模块则提供了与操作系统交互的功能,而cmd模块则用于创建命令行接口。通过结合这些模块的功能,你可以创建一个功能丰富的CMD工具,满足各种需求。希望本文的内容能够帮助你更好地理解和使用这些模块来实现自己的CMD工具。
相关问答FAQs:
如何在Python中执行系统命令?
Python提供了多个模块来执行系统命令,其中最常用的是subprocess
模块。通过这个模块,你可以运行外部命令,获取输出,甚至处理输入。以下是一个简单的示例代码:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这个代码将执行ls -l
命令,并打印出其输出结果。
用Python实现cmd的主要应用场景有哪些?
Python实现cmd的应用场景非常广泛,例如自动化脚本、文件处理、系统监控等。它可以帮助用户执行批量命令、分析命令行工具的输出,甚至在数据处理和网络爬虫中调用外部程序。
如何处理Python中cmd命令的错误?
在使用subprocess
模块时,可以通过check=True
参数来捕获错误并抛出异常。这样可以更方便地处理命令执行失败的情况。以下是一个示例:
try:
subprocess.run(['wrong_command'], check=True)
except subprocess.CalledProcessError as e:
print(f"Command failed with error: {e}")
这段代码会在命令执行失败时,捕获异常并输出错误信息。
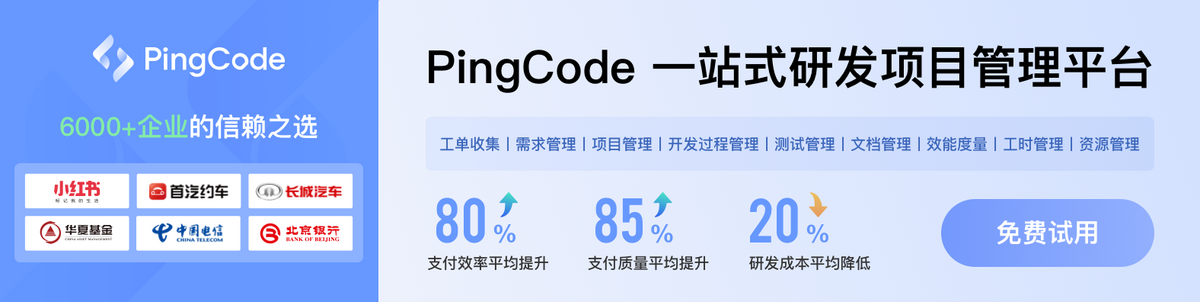