开头段落:
使用Python来ping的方式包括使用os库调用系统命令、使用subprocess模块、利用ping3库等。其中,使用subprocess模块是一个较为灵活和强大的方法。通过subprocess模块,Python可以执行系统命令,并获取命令的输出结果,这使得它非常适合用于需要与操作系统进行交互的任务。使用subprocess模块时,可以通过调用subprocess.run
或subprocess.Popen
来运行ping命令,并通过捕获标准输出、标准错误等方式来获取ping操作的结果。这种方式不仅可以实现基本的ping功能,还可以通过解析输出结果实现更复杂的网络监控和分析。
一、OS库调用系统命令
使用Python的os库是实现ping功能的最直接方法之一。通过调用系统的ping命令,Python可以轻松地实现网络连通性测试。
- 使用os.system方法
os.system是最简单的调用系统命令的方法。它执行命令并将输出直接显示在终端中。虽然简单,但它无法获取命令的输出结果。
import os
def ping_using_os(host):
response = os.system(f"ping -c 1 {host}")
return response == 0
- 使用os.popen方法
os.popen可以用于执行命令并捕获其输出。通过读取命令的输出,可以对结果进行更详细的分析。
import os
def ping_using_os_popen(host):
response = os.popen(f"ping -c 1 {host}").read()
return "1 received" in response
二、SUBPROCESS模块
Subprocess模块提供了更为强大的方式来执行系统命令,并能更好地控制输入输出流。
- 使用subprocess.run
subprocess.run是Python 3.5引入的新方法,它提供了一个简单而又强大的接口来执行命令并获取其输出。
import subprocess
def ping_using_subprocess_run(host):
try:
output = subprocess.run(["ping", "-c", "1", host], capture_output=True, text=True, check=True)
return "1 received" in output.stdout
except subprocess.CalledProcessError:
return False
- 使用subprocess.Popen
对于需要更复杂交互的场景,可以使用subprocess.Popen,它提供了对进程的更细粒度控制。
import subprocess
def ping_using_subprocess_popen(host):
process = subprocess.Popen(["ping", "-c", "1", host], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
return "1 received" in stdout.decode()
三、PING3库
ping3是一个轻量级的Python库,专门用于实现ping操作。它不依赖系统命令,因此可以跨平台使用。
- 安装ping3库
首先,需要通过pip安装ping3库。
pip install ping3
- 使用ping3库
ping3库提供了简单易用的接口来执行ping操作,并可以直接返回响应时间。
from ping3 import ping
def ping_using_ping3(host):
response_time = ping(host)
return response_time is not None
四、PING结果的解析与分析
在实际应用中,ping的结果不仅仅用于判断网络连通性,还可以用于分析网络性能。
- 解析ping输出
通过解析ping命令的输出,可以获取更多信息,如响应时间、丢包率等。
import subprocess
def parse_ping_output(host):
try:
output = subprocess.run(["ping", "-c", "4", host], capture_output=True, text=True, check=True)
lines = output.stdout.split('\n')
stats_line = lines[-3]
stats = stats_line.split(',')
packet_loss = stats[2].split('%')[0].strip()
return f"Packet Loss: {packet_loss}%"
except subprocess.CalledProcessError:
return "Ping failed"
- 分析网络性能
通过多次ping操作,可以统计网络的平均响应时间、抖动等指标,从而分析网络性能。
import statistics
from ping3 import ping
def analyze_network_performance(host, count=10):
response_times = []
for _ in range(count):
response_time = ping(host)
if response_time is not None:
response_times.append(response_time)
if response_times:
avg_time = statistics.mean(response_times)
jitter = statistics.stdev(response_times)
return f"Average Response Time: {avg_time:.2f} ms, Jitter: {jitter:.2f} ms"
else:
return "Ping failed"
五、跨平台的注意事项
在使用Python进行ping操作时,不同操作系统之间可能存在一些差异。
- Windows与Unix系统的区别
在Windows系统中,ping命令的参数可能与Unix系统有所不同。例如,在Windows中,使用-n
指定发送的包数,而在Unix中使用-c
。
import platform
import subprocess
def cross_platform_ping(host):
param = "-n" if platform.system().lower() == "windows" else "-c"
try:
output = subprocess.run(["ping", param, "1", host], capture_output=True, text=True, check=True)
return "1 received" in output.stdout
except subprocess.CalledProcessError:
return False
- 处理权限问题
在某些系统中,执行ping命令可能需要管理员权限,特别是在Windows中。
六、实用案例
通过整合上述方法,可以实现一个功能丰富的网络监控工具。
- 实现一个简单的网络监控工具
可以使用Python实现一个简单的网络监控工具,用于监控一组IP地址的连通性。
import time
from ping3 import ping
def network_monitor(hosts, interval=5):
while True:
for host in hosts:
response_time = ping(host)
if response_time is None:
print(f"{host} is unreachable")
else:
print(f"{host} responded in {response_time:.2f} ms")
time.sleep(interval)
hosts = ["8.8.8.8", "1.1.1.1"]
network_monitor(hosts)
- 扩展功能:日志记录与报警
可以为网络监控工具添加日志记录和报警功能,以便在网络出现问题时及时通知。
import logging
import smtplib
from email.mime.text import MIMEText
logging.basicConfig(filename='network_monitor.log', level=logging.INFO)
def send_alert(email, message):
msg = MIMEText(message)
msg['Subject'] = 'Network Alert'
msg['From'] = 'monitor@example.com'
msg['To'] = email
with smtplib.SMTP('smtp.example.com') as server:
server.login('username', 'password')
server.send_message(msg)
def network_monitor_with_alert(hosts, interval=5, alert_email='admin@example.com'):
while True:
for host in hosts:
response_time = ping(host)
if response_time is None:
message = f"{host} is unreachable"
logging.info(message)
send_alert(alert_email, message)
else:
message = f"{host} responded in {response_time:.2f} ms"
logging.info(message)
time.sleep(interval)
network_monitor_with_alert(hosts)
通过使用Python的os、subprocess模块以及ping3库,可以灵活地实现ping操作,并进行更深入的网络性能分析和监控。这些技术为开发网络监控和管理工具提供了坚实的基础。
相关问答FAQs:
如何在Python中执行Ping操作?
在Python中,可以使用subprocess
模块来执行ping命令。这个模块允许你生成新的进程,连接到他们的输入/输出/错误管道,并获得返回码。例如,使用以下代码可以实现ping功能:
import subprocess
def ping(host):
command = ['ping', '-c', '4', host] # '-c 4'表示发送4个ping包
result = subprocess.run(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
return result.stdout.decode()
print(ping('google.com'))
此代码将返回对指定主机的ping结果。
在Python中是否有现成的库可以进行Ping操作?
是的,Python中有多个第三方库可以简化Ping操作。一个常用的库是ping3
,它提供了简单的接口来进行ping测试。可以通过以下命令安装:
pip install ping3
使用示例如下:
from ping3 import ping
response = ping('google.com')
print(f'Ping response time: {response} seconds')
这个库不仅易于使用,还提供了更多的功能,如设置超时和获取IP地址等。
如何在Python中处理Ping命令的异常情况?
在执行Ping操作时,可能会遇到网络问题或目标主机不可达的情况。为了提高代码的健壮性,建议使用异常处理机制。以下是一个示例:
import subprocess
def ping(host):
try:
command = ['ping', '-c', '4', host]
result = subprocess.run(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, check=True)
return result.stdout.decode()
except subprocess.CalledProcessError:
return f"Ping to {host} failed."
except Exception as e:
return str(e)
print(ping('invalid.host'))
这样,代码将能够捕获并处理ping过程中可能出现的错误,提供更友好的用户体验。
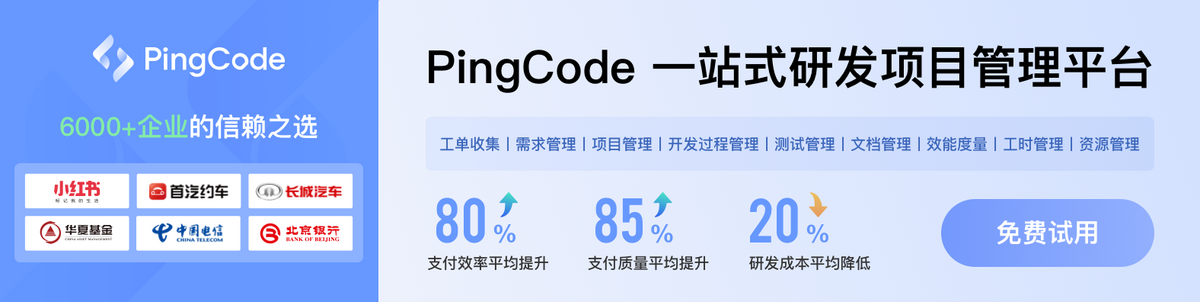