要用Python制作钟表,可以通过设计图形用户界面、运用时间模块、绘制表盘来实现。使用Tkinter模块、PIL库绘制表盘、时间模块获取当前时间是制作钟表的核心步骤。下面将详细介绍如何通过Python实现一个简单的钟表。
一、使用Tkinter创建基本窗口
Python的Tkinter模块是一个常用的图形用户界面库,可以用来创建窗口和各种图形元素。首先需要导入Tkinter模块并创建一个基本的窗口来承载我们的钟表。
import tkinter as tk
创建主窗口
root = tk.Tk()
root.title("Python Clock")
root.geometry("400x400")
二、绘制钟表表盘
为了绘制钟表的表盘,我们可以使用Python Imaging Library (PIL) 来绘制图像。PIL提供了丰富的图像处理功能,可以帮助我们绘制表盘和指针。
from PIL import Image, ImageDraw
创建表盘图像
def create_clock_face():
size = 300
clock_face = Image.new('RGB', (size, size), (255, 255, 255))
draw = ImageDraw.Draw(clock_face)
# 画表盘外圈
draw.ellipse((0, 0, size, size), outline="black", width=4)
# 画刻度
for i in range(12):
angle = i * 30
x1 = size / 2 + (size / 2 - 20) * math.cos(math.radians(angle))
y1 = size / 2 + (size / 2 - 20) * math.sin(math.radians(angle))
x2 = size / 2 + (size / 2 - 10) * math.cos(math.radians(angle))
y2 = size / 2 + (size / 2 - 10) * math.sin(math.radians(angle))
draw.line((x1, y1, x2, y2), fill="black", width=2)
return clock_face
显示表盘
clock_face = create_clock_face()
clock_face.show()
三、获取当前时间
Python的time
模块可以用来获取系统的当前时间。我们可以利用time
模块获取当前的时、分、秒,然后计算指针的角度。
import time
def get_current_time():
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
return hours, minutes, seconds
四、绘制指针
基于当前时间计算指针角度,然后在表盘上绘制时针、分针和秒针。可以使用简单的数学公式来计算每个指针的角度。
import math
绘制指针
def draw_hands(clock_face, hours, minutes, seconds):
size = 300
draw = ImageDraw.Draw(clock_face)
# 计算每个指针的角度
second_angle = seconds * 6
minute_angle = minutes * 6 + seconds * 0.1
hour_angle = hours * 30 + minutes * 0.5
# 画秒针
x = size / 2 + (size / 2 - 30) * math.cos(math.radians(second_angle - 90))
y = size / 2 + (size / 2 - 30) * math.sin(math.radians(second_angle - 90))
draw.line((size / 2, size / 2, x, y), fill="red", width=1)
# 画分针
x = size / 2 + (size / 2 - 40) * math.cos(math.radians(minute_angle - 90))
y = size / 2 + (size / 2 - 40) * math.sin(math.radians(minute_angle - 90))
draw.line((size / 2, size / 2, x, y), fill="black", width=3)
# 画时针
x = size / 2 + (size / 2 - 60) * math.cos(math.radians(hour_angle - 90))
y = size / 2 + (size / 2 - 60) * math.sin(math.radians(hour_angle - 90))
draw.line((size / 2, size / 2, x, y), fill="black", width=5)
return clock_face
五、实时更新钟表
为了让钟表实时更新,我们需要在Tkinter的主循环中定时刷新表盘和指针。可以使用after
方法来安排定时任务。
def update_clock():
hours, minutes, seconds = get_current_time()
clock_face = create_clock_face()
clock_face_with_hands = draw_hands(clock_face, hours, minutes, seconds)
# 更新Tkinter中的图像
tk_image = ImageTk.PhotoImage(clock_face_with_hands)
label.config(image=tk_image)
label.image = tk_image
# 每秒更新一次
root.after(1000, update_clock)
创建Tkinter标签来显示时钟
from PIL import ImageTk
label = tk.Label(root)
label.pack()
启动时钟更新
update_clock()
运行Tkinter主循环
root.mainloop()
通过以上步骤,你可以使用Python制作一个简单的图形化钟表。这个项目展示了如何结合图形用户界面、图像处理和时间管理模块来实现一个功能性的小工具。可以通过不断调整和改进代码,来增强钟表的功能和美观性。
相关问答FAQs:
如何在Python中创建一个简单的钟表应用?
要创建一个简单的钟表应用,您可以使用Python的Tkinter库来构建图形用户界面。首先,安装Tkinter库(通常预装在Python中),然后使用Label
组件来显示时间。通过time
模块获取当前时间并使用after
方法定时更新显示。示例代码如下:
import tkinter as tk
import time
def update_time():
current_time = time.strftime('%H:%M:%S')
label.config(text=current_time)
label.after(1000, update_time)
root = tk.Tk()
label = tk.Label(root, font=('calibri', 40), background='black', foreground='white')
label.pack(anchor='center')
update_time()
root.mainloop()
在Python中如何实现一个带有秒针和分针的模拟钟表?
实现带有秒针和分针的模拟钟表需要使用更为复杂的图形绘制。您可以使用matplotlib
库的pyplot
模块来绘制时钟的各个部分。通过计算每个指针的角度,根据当前时间来动态更新它们的位置。首先,确保安装了matplotlib
库,然后可以参考以下示例代码:
import matplotlib.pyplot as plt
import numpy as np
import time
def draw_clock():
plt.figure(figsize=(6, 6))
plt.xlim(-1.5, 1.5)
plt.ylim(-1.5, 1.5)
plt.gca().set_aspect('equal', adjustable='box')
# 画钟表的圆形边框
circle = plt.Circle((0, 0), 1, color='blue', fill=False)
plt.gca().add_artist(circle)
while True:
current_time = time.localtime()
seconds = current_time.tm_sec
minutes = current_time.tm_min
hours = current_time.tm_hour % 12
# 计算指针的角度
second_angle = (seconds / 60) * 360
minute_angle = (minutes / 60 + seconds / 3600) * 360
hour_angle = (hours / 12 + minutes / 720) * 360
plt.cla() # 清除之前的画面
plt.gca().add_artist(circle)
# 画秒针、分针和时针
plt.plot([0, np.sin(np.radians(second_angle))],
[0, np.cos(np.radians(second_angle))], color='red', linewidth=2)
plt.plot([0, np.sin(np.radians(minute_angle))],
[0, np.cos(np.radians(minute_angle))], color='green', linewidth=4)
plt.plot([0, np.sin(np.radians(hour_angle))],
[0, np.cos(np.radians(hour_angle))], color='blue', linewidth=6)
plt.pause(1) # 每秒更新一次
draw_clock()
使用Python制作钟表需要掌握哪些基本知识?
制作钟表的过程涉及多个编程知识点。首先,了解Python的基础语法和数据结构是必不可少的。其次,熟悉图形用户界面(GUI)库,比如Tkinter或Pygame,可以帮助您实现可视化效果。此外,掌握时间处理的相关模块,如time
和datetime
,也将使您更容易获取和格式化时间。最后,学习简单的数学计算(如角度转换)将有助于您设计更复杂的钟表功能。
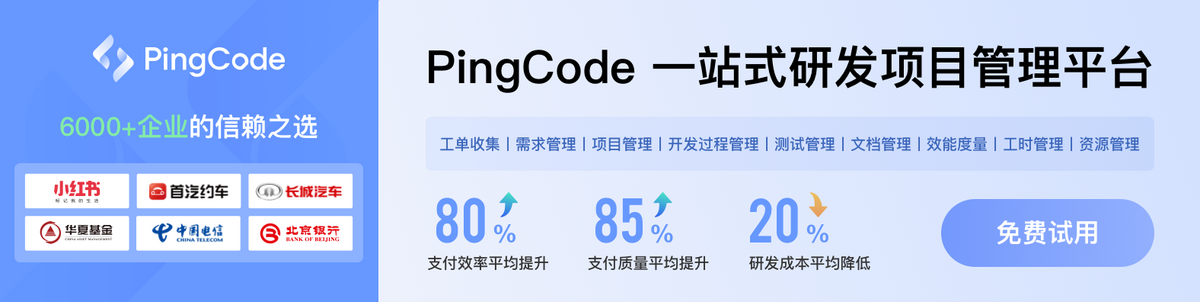