Python使用PyQt绘图的方法包括:使用QPainter类进行自定义绘图、利用QGraphicsView框架、使用Matplotlib与PyQt结合、通过PyQtGraph库进行高效绘图。
在PyQt中,QPainter类是主要的绘图工具。QPainter提供了丰富的功能来绘制各种形状和文本。我们可以通过重写QWidget的paintEvent方法来实现自定义绘图。在此方法中,创建一个QPainter对象,设置绘图颜色、笔刷等属性,然后调用相应的方法来绘制图形。使用QGraphicsView框架可以实现更加复杂的场景管理和绘图。结合Matplotlib可以实现更加专业的数据可视化,而PyQtGraph则提供了高性能的交互式绘图。接下来详细介绍这些方法。
一、QPAINTER类的使用
QPainter是PyQt中最基础的绘图类,用于在控件上绘制各种形状、文本和图像。通过重载控件的paintEvent方法,我们可以创建复杂的图形界面。
1、QPainter的基本使用
QPainter的使用步骤通常包括:
- 创建QPainter对象并开始绘制。
- 设置绘图的颜色、笔刷等。
- 使用QPainter提供的方法绘制各种图形。
- 绘制完成后,结束绘图。
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtGui import QPainter, QColor, QPen
from PyQt5.QtCore import Qt
class DrawingWidget(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('QPainter Demo')
self.setGeometry(100, 100, 600, 400)
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
# 设置画笔
pen = QPen(QColor(Qt.black), 2)
painter.setPen(pen)
# 绘制矩形
painter.drawRect(50, 50, 200, 100)
# 绘制椭圆
painter.drawEllipse(300, 50, 200, 100)
# 绘制直线
painter.drawLine(50, 200, 250, 350)
app = QApplication([])
window = DrawingWidget()
window.show()
app.exec_()
2、绘制路径和文本
除了基本形状,QPainter还允许绘制复杂路径和文本。我们可以使用QPainterPath类来定义复杂的绘图路径。
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
# 绘制文本
painter.drawText(50, 50, "Hello, PyQt5!")
# 绘制路径
path = QPainterPath()
path.moveTo(100, 100)
path.cubicTo(150, 50, 200, 150, 250, 100)
painter.drawPath(path)
二、QGRAPHICSVIEW框架
QGraphicsView框架提供了更加灵活和强大的绘图能力,适用于需要管理复杂场景和多个图形元素的应用。
1、QGraphicsScene和QGraphicsView
QGraphicsScene用于管理所有的图形项,而QGraphicsView用于将这些图形项呈现在界面上。通过这种分离,我们可以实现场景的复杂管理和高效渲染。
from PyQt5.QtWidgets import QApplication, QGraphicsView, QGraphicsScene, QGraphicsEllipseItem
app = QApplication([])
scene = QGraphicsScene()
ellipse = QGraphicsEllipseItem(0, 0, 100, 50)
scene.addItem(ellipse)
view = QGraphicsView(scene)
view.setWindowTitle('QGraphicsView Demo')
view.setGeometry(100, 100, 600, 400)
view.show()
app.exec_()
2、自定义图形项
我们可以通过继承QGraphicsItem类来自定义复杂的图形项,并实现自己的绘制逻辑。
from PyQt5.QtGui import QBrush
class CustomItem(QGraphicsEllipseItem):
def __init__(self, x, y, w, h):
super().__init__(x, y, w, h)
self.setBrush(QBrush(Qt.green))
scene.addItem(CustomItem(150, 150, 100, 50))
三、结合MATPLOTLIB进行绘图
Matplotlib是Python中最流行的数据可视化库之一,通过将其与PyQt结合,我们可以在PyQt应用中实现专业的图表绘制。
1、嵌入Matplotlib图表
我们可以通过FigureCanvasQTAgg将Matplotlib的图表嵌入到PyQt的Widget中。
from PyQt5.QtWidgets import QVBoxLayout
from matplotlib.backends.backend_qt5agg import FigureCanvasQTAgg as FigureCanvas
from matplotlib.figure import Figure
class MplWidget(QWidget):
def __init__(self):
super().__init__()
self.canvas = FigureCanvas(Figure())
self.axes = self.canvas.figure.add_subplot(111)
layout = QVBoxLayout()
layout.addWidget(self.canvas)
self.setLayout(layout)
def plot_example():
widget = MplWidget()
widget.axes.plot([0, 1, 2], [0, 1, 4])
widget.show()
return widget
app = QApplication([])
widget = plot_example()
app.exec_()
2、动态更新图表
通过Matplotlib与PyQt结合,我们可以实现实时数据的动态更新。
import numpy as np
def update_plot(widget):
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x)
widget.axes.cla()
widget.axes.plot(x, y)
widget.canvas.draw()
假设有一个定时器或其他机制来调用update_plot以更新图表
四、使用PYQTGRAPH库进行高效绘图
PyQtGraph是一个专为快速绘图而设计的库,适用于需要高性能和交互式绘图的应用。
1、基本绘图
PyQtGraph提供了一些简单的API用于快速绘制线条、点和图像。
import pyqtgraph as pg
app = QApplication([])
plot_widget = pg.plot(title="PyQtGraph Example")
plot_widget.plot([0, 1, 2, 3, 4], [10, 1, 3, 7, 5])
app.exec_()
2、交互式功能
PyQtGraph内置了许多交互式功能,如缩放、平移、鼠标交互等。
plot_widget = pg.PlotWidget()
plot_data_item = plot_widget.plot([0, 1, 2, 3, 4], [10, 1, 3, 7, 5], pen=pg.mkPen('r', width=2))
允许鼠标交互
plot_widget.setMouseEnabled(x=True, y=True)
五、总结与建议
在选择绘图工具时,需要根据实际应用的需求来决定。QPainter适用于简单的自定义控件绘图,QGraphicsView适合管理复杂的场景,Matplotlib擅长数据可视化,而PyQtGraph则在高性能和交互式绘图方面有优势。在实际开发中,可以根据应用的性能要求和复杂度选择合适的工具,并结合多个工具以获得最佳效果。
相关问答FAQs:
如何在PyQt中创建一个基本的绘图应用程序?
在PyQt中创建绘图应用程序需要使用QWidget或QMainWindow作为基础窗口,并重写其paintEvent方法。在paintEvent中,可以使用QPainter类进行绘图操作。通过设置画笔和画刷的属性,可以绘制线条、矩形、圆形等基本图形。以下是一个简单的示例代码,展示如何创建一个窗口并在其中绘制一个矩形:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.QtGui import QPainter, QColor
class DrawingApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("绘图应用程序")
self.setGeometry(100, 100, 600, 400)
def paintEvent(self, event):
painter = QPainter(self)
painter.setBrush(QColor(255, 0, 0))
painter.drawRect(50, 50, 200, 100)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = DrawingApp()
window.show()
sys.exit(app.exec_())
在PyQt中如何实现交互式绘图?
交互式绘图可以通过重写鼠标事件处理函数来实现,如mousePressEvent、mouseMoveEvent和mouseReleaseEvent。通过记录鼠标的坐标,可以在窗口中绘制用户拖动的线条或形状。结合QPainter的使用,用户可以在界面上进行自由绘制。例如,可以通过在mousePressEvent中记录起始点,在mouseMoveEvent中绘制线条,在mouseReleaseEvent中完成绘制。
PyQt绘图时如何优化性能?
为了提高绘图性能,可以考虑使用双缓冲技术。这种方法通过在内存中创建一个完整的图像,并在绘制完成后将其显示到屏幕上,从而减少闪烁现象。此外,使用QPixmap来存储绘制的内容也是一个有效的方式,这样可以在需要更新时只重绘变化的部分,而不是每次都重新绘制整个窗口。合理使用setViewport更新区域也可以显著提升性能。
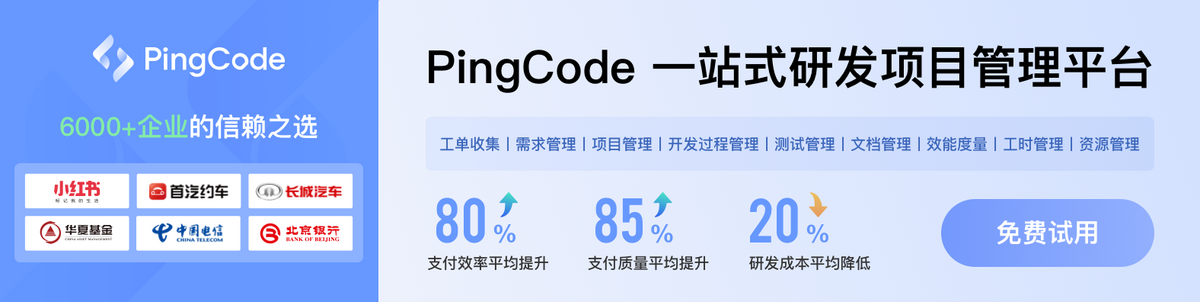