在Python中使用curl有几种方法:使用subprocess
模块调用系统命令、使用pycurl
库、使用requests
库进行HTTP请求。在这三种方法中,requests
库是最为流行和方便的方式,因为它提供了更高级别的API来处理HTTP请求。接下来,我们将详细介绍如何使用这三种方法。
一、使用subprocess
模块
subprocess
模块允许你在Python中执行系统命令,包括curl命令。这种方法直接利用了操作系统的curl命令行工具,因此不需要安装其他库。
1.1 调用curl命令
使用subprocess.run()
函数可以执行curl命令,并捕获其输出。
import subprocess
def use_curl_with_subprocess(url):
result = subprocess.run(['curl', '-X', 'GET', url], capture_output=True, text=True)
print(result.stdout)
use_curl_with_subprocess('http://example.com')
1.2 处理错误
在执行curl命令时,可能会遇到错误。我们可以通过检查返回码来处理这些错误。
import subprocess
def use_curl_with_subprocess_handling(url):
result = subprocess.run(['curl', '-X', 'GET', url], capture_output=True, text=True)
if result.returncode == 0:
print(result.stdout)
else:
print("Error:", result.stderr)
use_curl_with_subprocess_handling('http://example.com')
二、使用pycurl
库
pycurl
是一个Python库,提供了curl的功能,可以直接在Python中使用。它是libcurl的Python接口,适合需要高级配置的用户。
2.1 安装pycurl
首先,你需要安装pycurl
库。可以使用pip安装:
pip install pycurl
2.2 使用pycurl
进行HTTP请求
下面是一个简单的使用pycurl
的例子:
import pycurl
from io import BytesIO
def use_pycurl(url):
buffer = BytesIO()
c = pycurl.Curl()
c.setopt(c.URL, url)
c.setopt(c.WRITEDATA, buffer)
c.perform()
c.close()
body = buffer.getvalue().decode('utf-8')
print(body)
use_pycurl('http://example.com')
2.3 设置选项
pycurl
提供了许多选项来配置HTTP请求,例如设置请求头、超时时间等。
import pycurl
from io import BytesIO
def use_pycurl_with_options(url):
buffer = BytesIO()
c = pycurl.Curl()
c.setopt(c.URL, url)
c.setopt(c.WRITEDATA, buffer)
c.setopt(c.TIMEOUT, 5)
c.setopt(c.HTTPHEADER, ['User-Agent: Mozilla/5.0'])
c.perform()
c.close()
body = buffer.getvalue().decode('utf-8')
print(body)
use_pycurl_with_options('http://example.com')
三、使用requests
库
requests
是一个非常流行的HTTP库,虽然它不是curl的直接替代品,但它提供了更简单的接口来进行HTTP请求。
3.1 安装requests
可以使用pip安装requests
库:
pip install requests
3.2 发送HTTP请求
下面是一个使用requests
库进行HTTP GET请求的例子:
import requests
def use_requests(url):
response = requests.get(url)
print(response.text)
use_requests('http://example.com')
3.3 处理请求参数和头
requests
库允许你轻松添加请求参数和请求头。
import requests
def use_requests_with_params_and_headers(url):
headers = {'User-Agent': 'Mozilla/5.0'}
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get(url, headers=headers, params=params)
print(response.url)
print(response.text)
use_requests_with_params_and_headers('http://example.com')
3.4 处理响应
requests
库提供了对响应的丰富处理方式,包括检查状态码、解析JSON等。
import requests
def handle_response(url):
response = requests.get(url)
if response.status_code == 200:
print("Success!")
print(response.json())
else:
print("Failed with status code:", response.status_code)
handle_response('https://api.github.com')
四、总结
在Python中使用curl主要有三种方法:使用subprocess
模块、pycurl
库和requests
库。如果你的需求只是简单的HTTP请求,requests
库是最简单和直观的选择。而如果你需要更多的控制和功能,pycurl
提供了libcurl的完整功能,可以满足复杂的需求。使用subprocess
模块则适合那些希望直接使用系统curl命令的场景。
通过以上方法,你可以根据自己的需求选择最适合的方式在Python中实现curl功能。
相关问答FAQs:
如何在Python中调用curl命令?
在Python中,可以通过使用subprocess
模块来调用curl命令。可以使用subprocess.run()
或subprocess.Popen()
方法,传入curl命令及其参数,来执行HTTP请求并获取返回结果。例如:
import subprocess
url = "http://example.com"
response = subprocess.run(['curl', url], capture_output=True, text=True)
print(response.stdout)
这种方式可以实现与curl相同的功能,同时也能够处理返回的输出。
Python有替代curl的库吗?
是的,Python中有多个库可以替代curl的功能,如requests
库。这个库提供了更为简便和直观的HTTP请求方式。例如,使用requests库发送GET请求的代码如下:
import requests
response = requests.get("http://example.com")
print(response.text)
使用requests库不仅简化了代码,还提供了更好的错误处理和响应解析功能。
使用curl时如何处理HTTPS请求?
在使用curl进行HTTPS请求时,可以加上-k
或--insecure
选项来忽略SSL证书的验证。示例如下:
curl -k https://example.com
在Python中使用requests
库时,默认会验证SSL证书。如果需要忽略验证,可以设置verify=False
,如下所示:
import requests
response = requests.get("https://example.com", verify=False)
print(response.text)
不过,出于安全考虑,建议在生产环境中尽量避免关闭SSL验证。
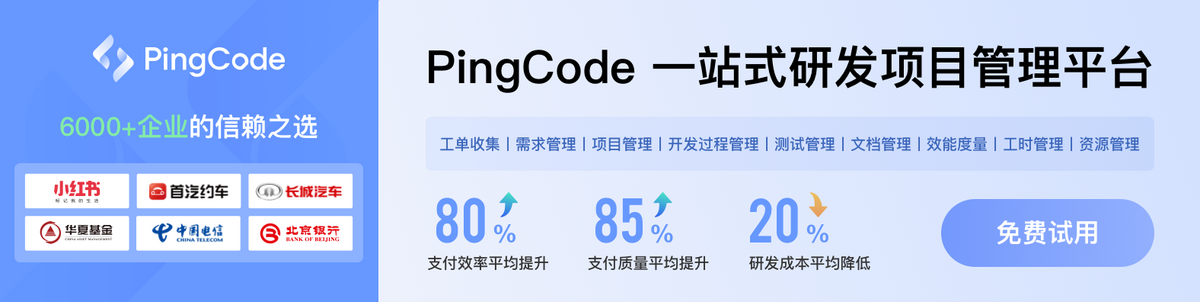