使用Python判断输出的方法主要包括:条件语句、循环语句、函数返回值、异常处理。这些方法可以帮助程序根据不同的条件作出不同的反应,从而实现更复杂的逻辑。条件语句是最基本的判断方式,通过if、elif和else语句来实现多种条件判断。
条件语句是Python中用于判断输出的基本方法。通过条件语句,我们可以让程序根据不同的条件执行不同的代码块。例如,通过if语句,可以判断一个数是否为正数。如果条件为真,执行相应的代码块;否则,执行else部分的代码。条件语句的强大之处在于可以嵌套使用,通过多个if和elif语句,可以实现复杂的条件判断。
一、条件语句
条件语句是Python中最基础的控制结构,它们允许程序根据特定条件执行不同的代码块。Python主要使用if、elif和else来实现条件判断。
1. IF语句
if语句用于判断一个条件是否为真,如果为真,则执行紧随其后的代码块。基本语法如下:
if condition:
# execute block of code
例如,要判断一个数字是否为正数,可以使用以下代码:
number = 10
if number > 0:
print("This is a positive number.")
2. IF-ELSE语句
if-else语句用于在条件为假时执行另一组代码。基本语法如下:
if condition:
# execute block of code if condition is true
else:
# execute block of code if condition is false
例如:
number = -5
if number > 0:
print("This is a positive number.")
else:
print("This is not a positive number.")
3. IF-ELIF-ELSE语句
if-elif-else语句用于检查多个条件。基本语法如下:
if condition1:
# execute block of code if condition1 is true
elif condition2:
# execute block of code if condition2 is true
else:
# execute block of code if none of the above conditions are true
例如:
number = 0
if number > 0:
print("This is a positive number.")
elif number == 0:
print("This is zero.")
else:
print("This is a negative number.")
二、循环语句
循环语句用于重复执行一段代码,直到条件不再满足。Python支持for循环和while循环。
1. FOR循环
for循环用于遍历序列(如列表、元组或字符串)。基本语法如下:
for variable in sequence:
# execute block of code
例如:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
2. WHILE循环
while循环在给定条件为真时重复执行代码块。基本语法如下:
while condition:
# execute block of code
例如:
count = 0
while count < 5:
print(count)
count += 1
三、函数返回值
函数是Python中实现代码复用的重要手段。通过函数的返回值,我们可以根据不同的输入条件返回不同的输出。
1. 定义函数
函数通过def关键字定义。基本语法如下:
def function_name(parameters):
# function body
return value
例如:
def is_even(number):
if number % 2 == 0:
return True
else:
return False
print(is_even(4)) # Output: True
2. 使用函数返回值
函数的返回值可以用于进一步的条件判断或计算。例如:
def max_of_two(a, b):
if a > b:
return a
else:
return b
result = max_of_two(5, 10)
print("The maximum number is:", result)
四、异常处理
异常处理用于捕获和处理程序运行时可能出现的错误,以避免程序崩溃。Python使用try、except、finally等关键字实现异常处理。
1. TRY-EXCEPT语句
try-except语句用于捕获异常并处理。基本语法如下:
try:
# block of code that may cause an exception
except ExceptionType:
# block of code to handle the exception
例如:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
2. TRY-EXCEPT-ELSE-FINALLY语句
try-except-else-finally语句提供了更细粒度的异常处理机制。基本语法如下:
try:
# block of code that may cause an exception
except ExceptionType1:
# block of code to handle ExceptionType1
except ExceptionType2:
# block of code to handle ExceptionType2
else:
# block of code to be executed if no exceptions occur
finally:
# block of code to be executed no matter what
例如:
try:
file = open("example.txt", "r")
content = file.read()
except FileNotFoundError:
print("File not found.")
else:
print(content)
finally:
if 'file' in locals():
file.close()
五、复杂条件判断
复杂条件判断结合了条件语句和逻辑运算符(如and、or、not)以实现更复杂的逻辑判断。
1. 使用逻辑运算符
逻辑运算符允许在条件语句中组合多个条件。基本语法如下:
if condition1 and condition2:
# execute block of code if both conditions are true
if condition1 or condition2:
# execute block of code if at least one condition is true
if not condition:
# execute block of code if condition is false
例如:
age = 25
has_license = True
if age >= 18 and has_license:
print("You can drive.")
else:
print("You cannot drive.")
2. 嵌套条件语句
嵌套条件语句用于在一个条件语句内部包含另一个条件语句。基本语法如下:
if condition1:
if condition2:
# execute block of code if both conditions are true
例如:
number = -5
if number >= 0:
if number == 0:
print("Zero")
else:
print("Positive number")
else:
print("Negative number")
六、实用示例
在实际应用中,Python的判断输出功能被广泛应用于数据处理、用户输入验证、自动化任务等领域。以下是一些常见应用示例。
1. 用户输入验证
用户输入验证用于确保用户输入的数据符合预期格式和范围。
def get_positive_number():
while True:
try:
number = float(input("Enter a positive number: "))
if number > 0:
return number
else:
print("The number must be positive.")
except ValueError:
print("Invalid input. Please enter a numeric value.")
positive_number = get_positive_number()
print("You entered:", positive_number)
2. 数据处理
在数据处理过程中,判断输出功能用于数据清洗、过滤等操作。
data = [1, -2, 3, -4, 5]
Filter out negative numbers
positive_data = [num for num in data if num > 0]
print("Positive numbers:", positive_data)
3. 自动化任务
在自动化任务中,判断输出功能用于根据不同的条件执行不同的自动化步骤。
import time
def perform_task(condition):
if condition == "start":
print("Task started.")
elif condition == "process":
print("Task in progress...")
time.sleep(2)
elif condition == "end":
print("Task completed.")
else:
print("Unknown condition.")
perform_task("start")
perform_task("process")
perform_task("end")
通过这些方法,Python程序可以根据不同的条件进行判断和输出,从而实现灵活复杂的功能。无论是在开发简单的脚本还是复杂的应用程序,掌握Python的判断输出功能都是至关重要的。
相关问答FAQs:
如何在Python中判断一个数是正数、负数还是零?
在Python中,可以使用简单的条件语句来判断一个数是正数、负数还是零。以下是一个示例代码:
num = float(input("请输入一个数字: "))
if num > 0:
print("该数字是正数。")
elif num < 0:
print("该数字是负数。")
else:
print("该数字是零。")
通过这种方式,用户可以输入不同的数字并查看相应的输出结果。
Python中如何根据条件输出不同的结果?
在Python中,使用if
、elif
和else
语句可以根据不同的条件输出不同的结果。例如,可以根据用户的输入决定输出不同的消息。以下是示例:
age = int(input("请输入您的年龄: "))
if age < 18:
print("您是未成年人。")
elif age < 65:
print("您是成年人。")
else:
print("您是老年人。")
这种方式使得程序更具交互性和灵活性。
如何在Python中使用逻辑运算符进行复杂判断?
逻辑运算符如and
和or
可以用于组合多个条件进行复杂判断。例如,判断一个数是否在特定范围内可以这样实现:
num = float(input("请输入一个数字: "))
if num >= 10 and num <= 20:
print("该数字在10到20之间。")
elif num < 10:
print("该数字小于10。")
else:
print("该数字大于20。")
使用逻辑运算符可以使条件判断更加精确,适合更复杂的应用场景。
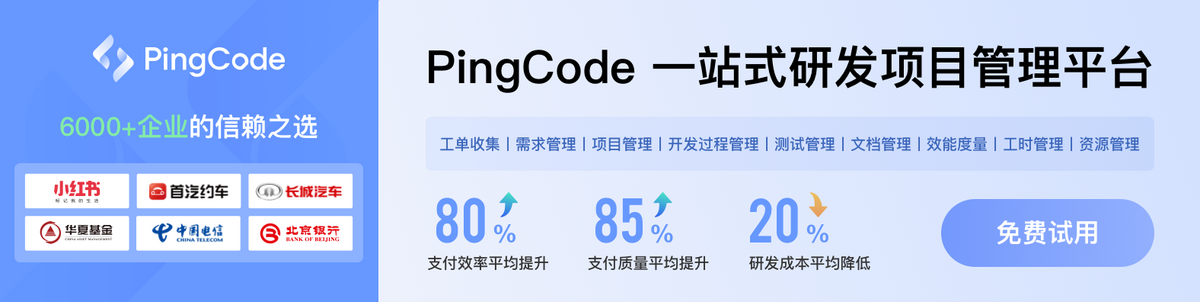