在Python中设置金额单位可以通过使用字符串格式化、使用库函数、创建自定义函数等方式实现。具体方法包括:使用字符串格式化设置金额单位、使用locale模块处理本地化货币格式、利用第三方库如Babel进行货币格式化。本文将详细介绍这些方法,并结合实际应用场景进行说明。
一、使用字符串格式化设置金额单位
在Python中,字符串格式化是一种简单而有效的方式来设置金额单位。通过格式化字符串,我们可以将数字格式化为带有货币符号和千位分隔符的字符串。
1.1 使用f-string格式化
f-string是Python 3.6引入的一种格式化字符串的方式,它支持在字符串中直接嵌入表达式。我们可以使用它来格式化金额。
amount = 1234567.89
formatted_amount = f"${amount:,.2f}"
print(formatted_amount) # 输出: $1,234,567.89
在上面的例子中,:,.2f
表示将数字格式化为带有千位分隔符并保留两位小数的浮点数。
1.2 使用format方法
format方法是Python提供的另一种字符串格式化方式,它与f-string类似。
amount = 1234567.89
formatted_amount = "${:,.2f}".format(amount)
print(formatted_amount) # 输出: $1,234,567.89
二、使用locale模块处理本地化货币格式
locale模块用于处理与语言和地区相关的操作,包括货币格式化。通过设置适当的区域设置,我们可以格式化金额以匹配特定国家/地区的货币格式。
2.1 设置区域和格式化货币
首先,我们需要导入locale模块并设置所需的区域。
import locale
设置区域为美国
locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
amount = 1234567.89
formatted_amount = locale.currency(amount, grouping=True)
print(formatted_amount) # 输出: $1,234,567.89
通过调用locale.currency()
函数,我们可以根据设置的区域格式化金额。grouping=True
参数用于启用千位分隔符。
2.2 处理不同国家的货币格式
locale模块支持多种语言和地区的货币格式。我们只需更改区域设置,即可格式化不同国家的货币。
import locale
设置区域为德国
locale.setlocale(locale.LC_ALL, 'de_DE.UTF-8')
amount = 1234567.89
formatted_amount = locale.currency(amount, grouping=True)
print(formatted_amount) # 输出: 1.234.567,89 €
在这个例子中,我们将区域设置为德国,金额格式化为德国的货币格式。
三、利用第三方库Babel进行货币格式化
Babel是一个国际化和本地化库,支持多种语言和地区的货币格式化。它提供了更灵活和强大的功能来处理金额单位。
3.1 安装Babel库
首先,我们需要安装Babel库。可以通过pip命令进行安装:
pip install Babel
3.2 使用Babel格式化货币
Babel提供了一个名为format_currency
的函数,用于格式化货币。
from babel.numbers import format_currency
amount = 1234567.89
formatted_amount = format_currency(amount, 'USD', locale='en_US')
print(formatted_amount) # 输出: $1,234,567.89
在这个例子中,我们使用format_currency
函数格式化美元金额。
3.3 支持多种货币和区域
Babel支持多种货币和区域设置,只需更改货币代码和区域参数即可。
from babel.numbers import format_currency
amount = 1234567.89
格式化为欧元
formatted_amount_eur = format_currency(amount, 'EUR', locale='de_DE')
print(formatted_amount_eur) # 输出: 1.234.567,89 €
格式化为日元
formatted_amount_jpy = format_currency(amount, 'JPY', locale='ja_JP')
print(formatted_amount_jpy) # 输出: ¥1,234,568
四、创建自定义函数进行金额格式化
除了使用内置模块和第三方库,我们还可以创建自定义函数来格式化金额。这种方式可以根据具体需求灵活调整格式。
4.1 创建简单的金额格式化函数
def format_amount(amount, currency_symbol='$', decimal_places=2):
formatted_amount = f"{currency_symbol}{amount:,.{decimal_places}f}"
return formatted_amount
amount = 1234567.89
formatted_amount = format_amount(amount)
print(formatted_amount) # 输出: $1,234,567.89
这个函数接受金额、货币符号和小数位数作为参数,并返回格式化后的字符串。
4.2 扩展自定义函数以支持更多选项
我们可以扩展自定义函数,以支持更多的选项,比如千位分隔符和负数格式化。
def format_amount(amount, currency_symbol='$', decimal_places=2, use_grouping=True, negative_format='({})'):
if use_grouping:
formatted_amount = f"{currency_symbol}{amount:,.{decimal_places}f}"
else:
formatted_amount = f"{currency_symbol}{amount:.{decimal_places}f}"
if amount < 0:
formatted_amount = negative_format.format(formatted_amount)
return formatted_amount
amount = -1234567.89
formatted_amount = format_amount(amount)
print(formatted_amount) # 输出: ($1,234,567.89)
在这个扩展函数中,我们增加了对负数格式和千位分隔符的支持。
五、总结
在Python中设置金额单位有多种方式,每种方式都有其优缺点。字符串格式化简单易用,但不支持本地化;locale模块可以处理本地化货币格式,但可能需要手动安装区域设置;Babel库功能强大,适合需要支持多种语言和货币的应用;而自定义函数则提供了最大的灵活性,可以根据具体需求进行调整。
在实际应用中,选择哪种方式取决于项目的具体需求和复杂性。如果只是简单的格式化,字符串格式化可能已经足够;如果需要支持多语言和多货币,Babel库是一个不错的选择。无论选择哪种方式,确保金额的显示符合用户的预期和使用习惯是最重要的。
相关问答FAQs:
如何在Python中自定义金额单位格式?
在Python中,可以使用locale
模块或Babel
库来设置金额的单位格式。locale
模块允许你根据地区设置货币格式,而Babel
库则提供了更强大的国际化支持,包括货币符号和格式的自定义。使用这些工具可以确保金额在输出时符合用户的地区习惯。
Python中有没有推荐的库用于金额格式化?
是的,Babel
库是一个非常流行的选择。它不仅支持金额的格式化,还能够处理日期、时间和文本的国际化。通过安装Babel
,你可以轻松地格式化货币,并根据不同地区显示相应的货币符号。例如,可以使用format_currency
函数来格式化金额,确保输出符合用户的期望。
如何处理Python中金额的四舍五入问题?
在Python中处理金额时,可以使用Decimal
模块来避免浮点数运算的不精确性。Decimal
支持四舍五入,能够精确控制小数位数和舍入方式。通过设置适当的上下文,可以确保金额计算的准确性,从而避免因浮点数误差导致的金额显示不正确的问题。
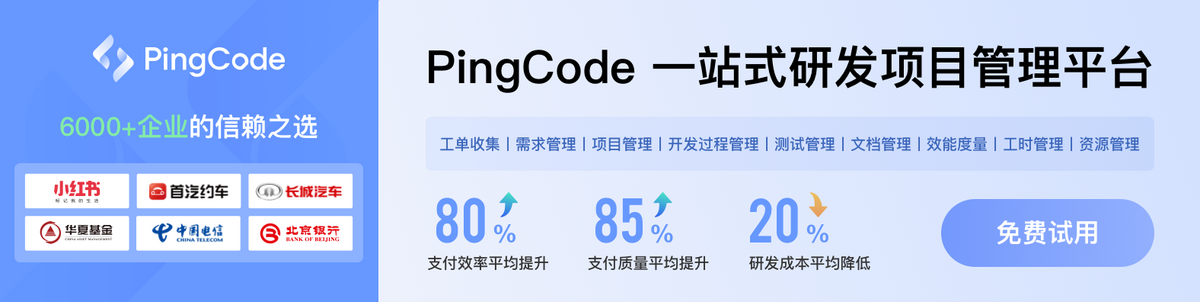