在Python中实现POST请求的方法有多种,包括使用requests库、http.client库、以及urllib库。使用requests库是最简单、最常见的方法,因为它提供了直观的API、易于使用。 下面将详细介绍如何使用requests库实现POST请求,并对其进行详细描述。
使用requests库进行POST请求时,只需导入requests库并调用其post方法即可。requests库提供了简单而强大的方法来处理HTTP请求,使得开发人员无需处理底层的细节。它支持各种HTTP请求方法,并且允许轻松地添加请求头、发送数据以及处理响应。下面将详细讲述requests库的使用,包括发送JSON数据、表单数据、文件上传以及异常处理等。
一、使用REQUESTS库实现POST请求
- 安装和导入requests库
在使用requests库之前,需确保已安装该库。可以通过pip安装:
pip install requests
安装完成后,便可以在Python脚本中导入该库:
import requests
- 发送简单的POST请求
使用requests库发送POST请求非常简单,只需调用requests.post()
方法并传入目标URL即可:
url = 'https://example.com/api'
response = requests.post(url)
这种情况下,我们发送了一个空的POST请求,通常需要在请求中附加数据。
- 发送表单数据
POST请求常用于提交表单数据,可以通过data
参数传递:
url = 'https://example.com/login'
data = {
'username': 'your_username',
'password': 'your_password'
}
response = requests.post(url, data=data)
- 发送JSON数据
在现代Web应用中,POST请求通常以JSON格式发送数据。可以使用json
参数来传递:
url = 'https://example.com/api'
json_data = {
'name': 'John Doe',
'email': 'john.doe@example.com'
}
response = requests.post(url, json=json_data)
requests库会自动将Python字典转换为JSON字符串,并设置适当的请求头。
- 上传文件
POST请求也可用于上传文件。可以使用files
参数实现文件上传:
url = 'https://example.com/upload'
files = {'file': open('report.pdf', 'rb')}
response = requests.post(url, files=files)
确保以二进制模式打开文件。
- 添加请求头
有时需要在请求中添加自定义头信息,可以使用headers
参数:
url = 'https://example.com/api'
headers = {
'Authorization': 'Bearer your_token',
'Content-Type': 'application/json'
}
response = requests.post(url, json=json_data, headers=headers)
- 处理响应
requests.post()
方法返回一个Response
对象,其中包含响应的状态码、内容等信息。可以通过以下方式处理:
if response.status_code == 200:
print('Success:', response.json())
else:
print('Failed:', response.status_code)
- 处理异常
在进行网络请求时,可能会遇到各种异常情况。可以使用try-except块来捕获和处理:
try:
response = requests.post(url, json=json_data)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except Exception as err:
print(f'Other error occurred: {err}')
else:
print('Success:', response.json())
二、使用HTTP.CLIENT库实现POST请求
虽然requests库更为流行,但Python标准库中的http.client模块也能实现POST请求。
- 发送POST请求
可以使用http.client库创建一个HTTP连接并发送POST请求:
import http.client
import json
conn = http.client.HTTPSConnection('example.com')
headers = {'Content-type': 'application/json'}
json_data = json.dumps({'name': 'John Doe', 'email': 'john.doe@example.com'})
conn.request('POST', '/api', json_data, headers)
response = conn.getresponse()
print(response.status, response.reason)
print(response.read().decode())
conn.close()
该方法需要手动处理JSON序列化和请求头设置。
三、使用URLLIB库实现POST请求
urllib库是Python标准库的一部分,也可以用于发送POST请求。
- 发送POST请求
可以使用urllib.request模块发送POST请求:
import urllib.request
import urllib.parse
import json
url = 'https://example.com/api'
data = {'name': 'John Doe', 'email': 'john.doe@example.com'}
headers = {'Content-Type': 'application/json'}
data = json.dumps(data).encode('utf-8')
req = urllib.request.Request(url, data=data, headers=headers)
response = urllib.request.urlopen(req)
print(response.status, response.reason)
print(response.read().decode())
这种方法需要手动处理字节编码以及请求头设置。
四、总结
在Python中实现POST请求的方法多种多样,选择使用何种库取决于具体需求和偏好。requests库以其简洁的API和丰富的功能成为最受欢迎的选择,而标准库中的http.client和urllib则提供了更多底层的控制。通过掌握这些工具,开发者可以轻松地在Python中实现各种复杂的HTTP请求操作。
相关问答FAQs:
如何在Python中发送POST请求?
在Python中,可以使用内置的requests
库轻松发送POST请求。首先,确保已安装该库,可以使用pip install requests
进行安装。发送POST请求的基本代码示例如下:
import requests
url = 'http://example.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=data)
print(response.status_code)
print(response.text)
这段代码将数据以表单形式发送到指定的URL,并返回响应的状态码和内容。
使用POST请求时,如何处理JSON数据?
在发送JSON数据时,可以使用json
参数而不是data
参数。这样,requests
库会自动将数据转换为JSON格式并设置适当的Content-Type
头。示例如下:
import requests
import json
url = 'http://example.com/api'
json_data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=json_data)
print(response.status_code)
print(response.json()) # 如果响应内容是JSON格式
这种方法便于与API交互,特别是在需要发送结构化数据时。
如何处理POST请求的响应?
处理POST请求响应时,可以检查响应状态码以确认请求是否成功。若状态码为200,表示请求成功,其他状态码可能表明错误或其他情况。可以使用response.text
获取文本内容,或者使用response.json()
直接解析JSON格式的响应。例如:
if response.status_code == 200:
data = response.json()
print('成功获取数据:', data)
else:
print('请求失败,状态码:', response.status_code)
这种方式能够有效地帮助开发者进行错误处理和数据提取。
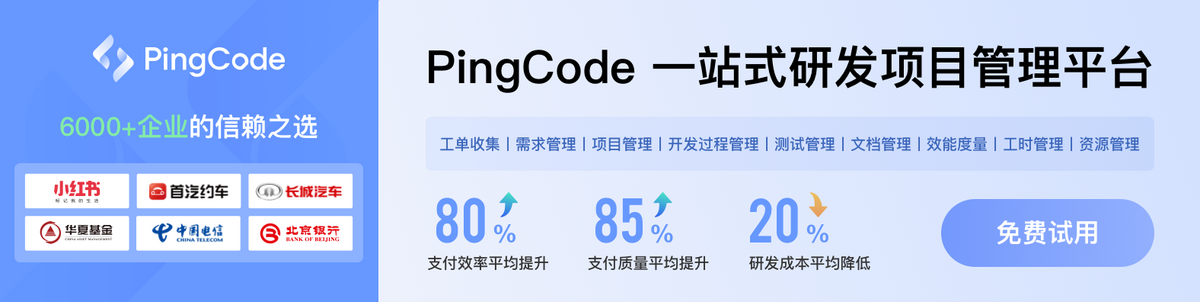