一、编写Python温度转换程序的关键在于理解温度转换公式、熟悉Python基本语法、编写函数实现转换。首先,我们需要了解摄氏度、华氏度和开尔文之间的转换公式:摄氏度到华氏度使用公式 ( F = C \times \frac{9}{5} + 32 );摄氏度到开尔文使用公式 ( K = C + 273.15 )。然后,我们可以利用Python的函数定义功能,将这些公式实现为简单的函数。接下来,我们将详细讨论如何通过编写Python程序来实现这些温度转换。
二、理解温度转换公式
在编写温度转换程序之前,必须理解温度的不同测量单位及其相互转换的公式。以下是主要的温度单位及其转换公式:
-
摄氏度与华氏度之间的转换
- 摄氏度到华氏度:公式为 ( F = C \times \frac{9}{5} + 32 )
- 华氏度到摄氏度:公式为 ( C = (F – 32) \times \frac{5}{9} )
-
摄氏度与开尔文之间的转换
- 摄氏度到开尔文:公式为 ( K = C + 273.15 )
- 开尔文到摄氏度:公式为 ( C = K – 273.15 )
-
华氏度与开尔文之间的转换
- 华氏度到开尔文:首先将华氏度转换为摄氏度,然后再转换为开尔文
- 开尔文到华氏度:首先将开尔文转换为摄氏度,然后再转换为华氏度
通过理解这些公式,我们可以在Python中轻松实现不同温度单位之间的转换。
三、Python基础语法概述
在开始编写温度转换程序之前,熟悉Python的基本语法是非常有用的。以下是一些关键的Python语法概念:
-
变量和数据类型
- Python支持多种数据类型,如整数(int)、浮点数(float)、字符串(str)等。在温度转换程序中,我们主要使用浮点数来表示温度值。
-
函数定义
-
使用关键字
def
可以定义一个函数。函数可以接收参数,并返回计算结果。例如,定义一个函数将摄氏度转换为华氏度:def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
-
-
输入和输出
- 使用
input()
函数可以从用户获取输入,print()
函数用于输出结果。
- 使用
-
条件语句和循环
- 使用
if
、elif
和else
可以实现条件判断。循环可以使用for
或while
。
- 使用
这些基础语法是编写Python程序的基本构件。通过掌握它们,可以更灵活地实现各种功能。
四、编写温度转换程序
有了对温度转换公式和Python基础语法的理解,我们可以开始编写温度转换程序。以下是实现温度转换的步骤:
-
定义转换函数
首先,为每种转换定义一个函数。这些函数将接收输入温度,并返回转换后的温度。例如:
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
def celsius_to_kelvin(celsius):
return celsius + 273.15
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
def fahrenheit_to_kelvin(fahrenheit):
celsius = fahrenheit_to_celsius(fahrenheit)
return celsius_to_kelvin(celsius)
def kelvin_to_fahrenheit(kelvin):
celsius = kelvin_to_celsius(kelvin)
return celsius_to_fahrenheit(celsius)
-
获取用户输入
使用
input()
函数从用户获取温度值和转换类型。可以提示用户选择要进行的转换类型:def get_user_input():
print("选择转换类型:")
print("1: 摄氏度到华氏度")
print("2: 华氏度到摄氏度")
print("3: 摄氏度到开尔文")
print("4: 开尔文到摄氏度")
print("5: 华氏度到开尔文")
print("6: 开尔文到华氏度")
choice = input("输入转换类型编号(1-6):")
temperature = float(input("输入温度值:"))
return choice, temperature
-
执行转换并输出结果
根据用户的选择调用相应的转换函数,并输出结果:
def main():
choice, temperature = get_user_input()
if choice == '1':
result = celsius_to_fahrenheit(temperature)
print(f"{temperature} 摄氏度 = {result} 华氏度")
elif choice == '2':
result = fahrenheit_to_celsius(temperature)
print(f"{temperature} 华氏度 = {result} 摄氏度")
elif choice == '3':
result = celsius_to_kelvin(temperature)
print(f"{temperature} 摄氏度 = {result} 开尔文")
elif choice == '4':
result = kelvin_to_celsius(temperature)
print(f"{temperature} 开尔文 = {result} 摄氏度")
elif choice == '5':
result = fahrenheit_to_kelvin(temperature)
print(f"{temperature} 华氏度 = {result} 开尔文")
elif choice == '6':
result = kelvin_to_fahrenheit(temperature)
print(f"{temperature} 开尔文 = {result} 华氏度")
else:
print("无效选择,请输入1-6之间的数字。")
if __name__ == "__main__":
main()
通过以上步骤,我们可以成功地编写一个Python程序,实现摄氏度、华氏度和开尔文之间的温度转换。该程序通过简单的用户输入和输出交互,提供了多种温度单位的转换功能。
五、优化和扩展
在完成基本的温度转换程序后,我们可以考虑如何优化和扩展程序,以提高其功能性和用户体验。
-
增加输入验证
为了提高程序的健壮性,可以在获取用户输入时增加验证,确保输入的温度值和选择的转换类型是有效的。例如:
def get_valid_input(prompt, valid_choices):
while True:
choice = input(prompt)
if choice in valid_choices:
return choice
else:
print("输入无效,请重新输入。")
def get_user_input():
print("选择转换类型:")
print("1: 摄氏度到华氏度")
print("2: 华氏度到摄氏度")
print("3: 摄氏度到开尔文")
print("4: 开尔文到摄氏度")
print("5: 华氏度到开尔文")
print("6: 开尔文到华氏度")
choice = get_valid_input("输入转换类型编号(1-6):", ['1', '2', '3', '4', '5', '6'])
temperature = float(input("输入温度值:"))
return choice, temperature
-
添加循环以实现重复转换
为了提高用户体验,可以添加一个循环,使得用户在完成一次转换后可以选择继续转换或退出程序:
def main():
while True:
choice, temperature = get_user_input()
if choice == '1':
result = celsius_to_fahrenheit(temperature)
print(f"{temperature} 摄氏度 = {result} 华氏度")
elif choice == '2':
result = fahrenheit_to_celsius(temperature)
print(f"{temperature} 华氏度 = {result} 摄氏度")
elif choice == '3':
result = celsius_to_kelvin(temperature)
print(f"{temperature} 摄氏度 = {result} 开尔文")
elif choice == '4':
result = kelvin_to_celsius(temperature)
print(f"{temperature} 开尔文 = {result} 摄氏度")
elif choice == '5':
result = fahrenheit_to_kelvin(temperature)
print(f"{temperature} 华氏度 = {result} 开尔文")
elif choice == '6':
result = kelvin_to_fahrenheit(temperature)
print(f"{temperature} 开尔文 = {result} 华氏度")
continue_choice = input("是否继续转换?(y/n):")
if continue_choice.lower() != 'y':
break
if __name__ == "__main__":
main()
-
扩展功能
我们还可以扩展该程序以支持更多的功能,例如:
- 批量转换:允许用户输入多个温度值,并对每个值执行转换。
- 图形用户界面(GUI):使用Python的GUI库(如Tkinter)构建一个简单的图形界面,使程序更加用户友好。
- 记录转换历史:保存每次转换的输入和输出,以供将来参考。
通过这些扩展,温度转换程序可以变得更加实用和多功能。
总结,通过理解温度转换公式、掌握Python基础语法,我们可以编写一个功能完善的温度转换程序。通过优化输入验证和增加循环功能,可以提高程序的健壮性和用户体验。此外,通过扩展功能,程序可以满足更多用户需求。
相关问答FAQs:
如何使用Python实现摄氏度与华氏度之间的转换?
在Python中,可以使用简单的公式来进行摄氏度和华氏度之间的转换。摄氏度转华氏度的公式是 F = C * 9/5 + 32
,而华氏度转摄氏度的公式是 C = (F - 32) * 5/9
。您可以创建一个函数来接收输入并返回转换后的值。例如:
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
在Python中如何处理用户输入的温度转换?
为了处理用户输入,您可以使用 input()
函数获取用户输入的温度,并根据需要进行转换。您可以提供一个简单的菜单让用户选择转换方向。示例代码如下:
temp = float(input("请输入温度:"))
unit = input("请输入单位(C表示摄氏度,F表示华氏度):").strip().upper()
if unit == 'C':
print(f"{temp} 摄氏度 = {celsius_to_fahrenheit(temp)} 华氏度")
elif unit == 'F':
print(f"{temp} 华氏度 = {fahrenheit_to_celsius(temp)} 摄氏度")
else:
print("无效的单位,请输入C或F。")
使用Python进行温度转换时,可以处理哪些常见的错误?
在进行温度转换时,常见的错误包括用户输入非数字字符或不正确的单位。通过使用 try...except
语句,您可以捕获这些错误并提示用户重新输入。例如:
try:
temp = float(input("请输入温度:"))
unit = input("请输入单位(C表示摄氏度,F表示华氏度):").strip().upper()
# 进行转换逻辑...
except ValueError:
print("请输入有效的数字温度。")
通过这些示例,您可以轻松实现温度转换,并确保程序在处理用户输入时更加健壮。
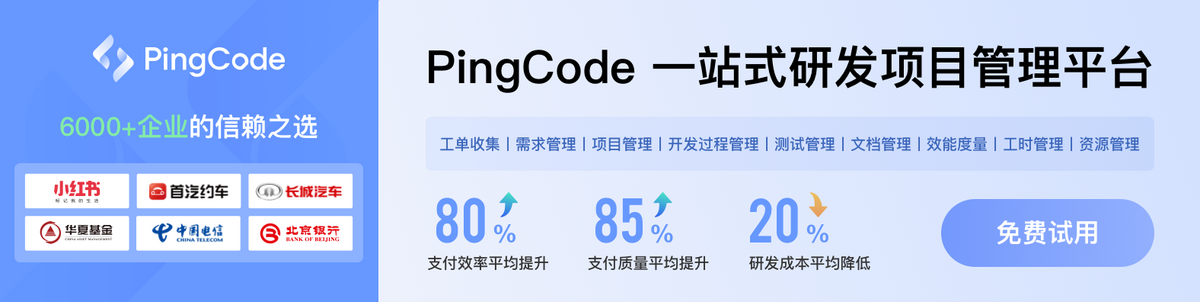