在Python中绘制GML(Geography Markup Language)文件可以使用多个库和工具。其中,读取GML文件、解析其内容、利用合适的可视化库进行绘图是主要步骤。以下是一种详细的方法来实现这一目标。
一、读取和解析GML文件
首先,必须能够读取和解析GML文件。GML是XML格式的地理信息文件,因此可以使用Python的XML处理库,如lxml
或xml.etree.ElementTree
,来解析GML文件。
1. 使用lxml库解析GML
lxml
库是一个功能强大的XML处理库,支持XPath和XSLT等高级功能,非常适合解析复杂的XML结构。
from lxml import etree
def parse_gml(file_path):
with open(file_path, 'rb') as file:
tree = etree.parse(file)
return tree
2. 提取几何数据
GML文件通常包含几何数据,如点、线、面等。解析后,需要提取这些数据,以便进行后续的绘制。
def extract_geometries(tree):
namespaces = {'gml': 'http://www.opengis.net/gml'}
geometries = tree.xpath('//gml:geometryMember', namespaces=namespaces)
extracted_data = []
for geometry in geometries:
# 处理不同类型的几何图形,如点、线、面
if geometry.find('.//gml:Point', namespaces=namespaces) is not None:
point = geometry.find('.//gml:Point/gml:coordinates', namespaces=namespaces).text
extracted_data.append(('Point', point))
elif geometry.find('.//gml:LineString', namespaces=namespaces) is not None:
line = geometry.find('.//gml:LineString/gml:coordinates', namespaces=namespaces).text
extracted_data.append(('LineString', line))
elif geometry.find('.//gml:Polygon', namespaces=namespaces) is not None:
polygon = geometry.find('.//gml:Polygon/gml:outerBoundaryIs/gml:LinearRing/gml:coordinates', namespaces=namespaces).text
extracted_data.append(('Polygon', polygon))
return extracted_data
二、使用matplotlib进行绘图
matplotlib
是Python中一个强大的绘图库,适用于各种图形和地图的绘制。
1. 数据转换
首先需要将提取的几何数据转换成可以绘图的形式。
def convert_coordinates(coordinates):
# GML坐标通常是以空格分隔的经纬度对
points = coordinates.split()
converted_points = [(float(p.split(',')[0]), float(p.split(',')[1])) for p in points]
return converted_points
2. 绘制几何图形
使用matplotlib
绘制从GML文件中提取的几何图形。
import matplotlib.pyplot as plt
def plot_geometries(geometries):
for geom_type, coords in geometries:
points = convert_coordinates(coords)
x, y = zip(*points)
if geom_type == 'Point':
plt.scatter(x, y, label='Point')
elif geom_type == 'LineString':
plt.plot(x, y, label='LineString')
elif geom_type == 'Polygon':
plt.fill(x, y, alpha=0.5, label='Polygon')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title('GML Geometries')
plt.legend()
plt.show()
三、示例与执行
通过上述步骤,您可以解析GML文件并绘制其内容。以下是一个完整的示例,展示如何从GML文件中读取数据并绘制它们。
def main():
file_path = 'path_to_your_gml_file.gml'
tree = parse_gml(file_path)
geometries = extract_geometries(tree)
plot_geometries(geometries)
if __name__ == '__main__':
main()
四、处理复杂的GML文件
实际应用中,GML文件可能包含复杂的几何形状和多种空间对象。为了处理这些情况,可能需要:
1. 扩展数据提取功能
根据GML文件的具体结构,可能需要扩展数据提取逻辑,以支持更复杂的几何形状和属性。例如,支持多段线、多边形的内部环等。
# 示例:提取多边形的内部环
def extract_polygon_interior_rings(polygon_element, namespaces):
interior_rings = polygon_element.findall('.//gml:innerBoundaryIs/gml:LinearRing/gml:coordinates', namespaces=namespaces)
interior_coords = [ring.text for ring in interior_rings]
return interior_coords
2. 增强绘图功能
为了更好地展示复杂的几何形状,可以在绘图时添加更多的样式和注释。可以使用matplotlib
的高级功能,如颜色映射、透明度设置、标签等。
def plot_geometries_with_styles(geometries):
for geom_type, coords in geometries:
points = convert_coordinates(coords)
x, y = zip(*points)
if geom_type == 'Point':
plt.scatter(x, y, c='red', edgecolor='k', label='Point', s=100)
elif geom_type == 'LineString':
plt.plot(x, y, c='blue', linewidth=2, label='LineString')
elif geom_type == 'Polygon':
plt.fill(x, y, alpha=0.5, label='Polygon', facecolor='green', edgecolor='black')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title('Styled GML Geometries')
plt.legend(loc='upper right')
plt.grid(True)
plt.show()
五、其他可视化工具
除了matplotlib
,还有其他可视化工具可以用于GML数据的绘制:
1. 使用geopandas
和shapely
geopandas
是一个专门用于地理数据处理的库,shapely
则用于处理几何对象。结合使用,可以方便地处理和可视化GML文件。
import geopandas as gpd
from shapely.wkt import loads
def convert_gml_to_geodataframe(geometries):
geoms = []
for geom_type, coords in geometries:
if geom_type == 'Point':
geoms.append(loads(f'POINT({coords})'))
elif geom_type == 'LineString':
geoms.append(loads(f'LINESTRING({coords})'))
elif geom_type == 'Polygon':
geoms.append(loads(f'POLYGON(({coords}))'))
return gpd.GeoDataFrame(geometry=geoms)
def plot_with_geopandas(gdf):
gdf.plot()
plt.show()
在main函数中使用
gdf = convert_gml_to_geodataframe(geometries)
plot_with_geopandas(gdf)
2. 使用folium
进行交互式地图
folium
可以用来创建交互式地图,适合展示地理数据。
import folium
def plot_geometries_on_interactive_map(geometries):
m = folium.Map(location=[0, 0], zoom_start=2)
for geom_type, coords in geometries:
points = convert_coordinates(coords)
if geom_type == 'Point':
folium.Marker(location=points[0]).add_to(m)
elif geom_type == 'LineString':
folium.PolyLine(locations=points, color='blue').add_to(m)
elif geom_type == 'Polygon':
folium.Polygon(locations=points, color='green', fill=True).add_to(m)
return m
在main函数中使用
interactive_map = plot_geometries_on_interactive_map(geometries)
interactive_map.save('gml_map.html')
通过这些步骤和工具,您可以在Python中轻松地解析并绘制GML文件。根据需求,您可以选择不同的库和方法,以最佳方式展示地理数据。
相关问答FAQs:
如何使用Python读取GML文件并提取数据?
要读取GML文件,可以使用网络分析库如networkx
或xml.etree.ElementTree
。首先安装相关库,并使用networkx.read_gml()
函数加载GML文件。提取数据后,可以以字典或其他格式进行处理,方便后续分析。
绘制GML文件中图形的最佳库是什么?
常用的库包括matplotlib
和networkx
。networkx
可以直接处理图形数据,并与matplotlib
结合使用,绘制出清晰的图形。通过networkx.draw()
函数,可以快速将GML文件中的图形可视化,支持多种绘制选项。
在Python中如何自定义GML图形的外观?
在使用networkx
绘制图形时,可以通过参数自定义节点和边的样式。例如,可以设置节点的颜色、大小和形状,边的颜色和宽度等。使用matplotlib
的设置功能,可以进一步调整图形的布局和整体风格,使其更符合需求。
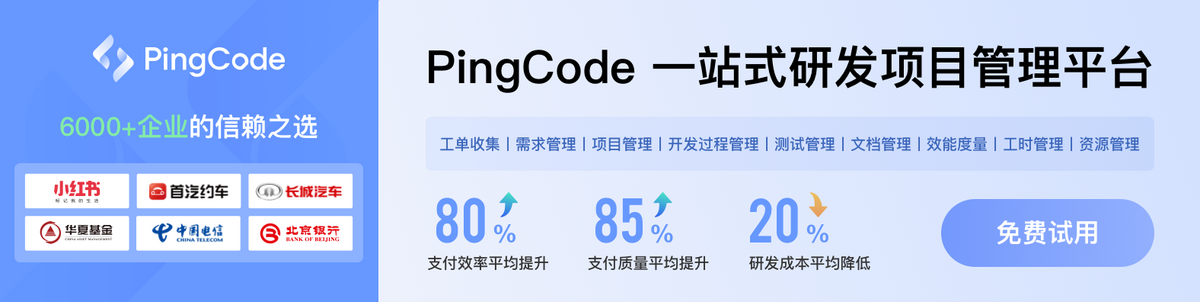