在Python中实现语音识别,可以通过使用一些强大的库,如SpeechRecognition、PyDub和PyAudio。主要步骤包括:安装必要的库、录制音频、处理音频文件、调用语音识别API、解析识别结果。其中,最关键的一步是选择合适的语音识别引擎,Google Web Speech API 是一个常用的选择,因为它易于使用且准确率高。下面详细介绍如何使用这些工具和库来实现语音识别。
一、安装必要的库
在开始编写代码之前,确保安装了一些必要的库。SpeechRecognition库是一个流行的Python库,用于执行语音识别任务。你可以通过以下命令安装它:
pip install SpeechRecognition
此外,还需要安装PyAudio库来处理音频流:
pip install PyAudio
如果需要处理音频文件,还可以安装PyDub库:
pip install pydub
二、录制音频
在语音识别过程中,首先需要录制音频输入。可以使用PyAudio库来录制音频。以下是一个简单的例子,展示了如何录制音频:
import pyaudio
import wave
def record_audio(filename, duration=5):
chunk = 1024 # Record in chunks of 1024 samples
sample_format = pyaudio.paInt16 # 16 bits per sample
channels = 2
fs = 44100 # Record at 44100 samples per second
p = pyaudio.PyAudio() # Create an interface to PortAudio
print('Recording')
stream = p.open(format=sample_format,
channels=channels,
rate=fs,
frames_per_buffer=chunk,
input=True)
frames = [] # Initialize array to store frames
# Store data in chunks for the specified duration
for _ in range(0, int(fs / chunk * duration)):
data = stream.read(chunk)
frames.append(data)
# Stop and close the stream
stream.stop_stream()
stream.close()
# Terminate the PortAudio interface
p.terminate()
print('Finished recording')
# Save the recorded data as a WAV file
wf = wave.open(filename, 'wb')
wf.setnchannels(channels)
wf.setsampwidth(p.get_sample_size(sample_format))
wf.setframerate(fs)
wf.writeframes(b''.join(frames))
wf.close()
record_audio('output.wav', duration=5)
这段代码会录制5秒的音频,并将其保存为WAV文件。
三、处理音频文件
在录制或获取音频文件后,可能需要对音频进行一些处理,以确保其格式和质量适合语音识别任务。使用PyDub库可以方便地转换音频格式、调整音量或剪辑音频。
from pydub import AudioSegment
def convert_audio(input_file, output_file):
audio = AudioSegment.from_file(input_file)
audio.export(output_file, format="wav")
convert_audio('input.mp3', 'output.wav')
这个函数可以将MP3文件转换为WAV格式。
四、调用语音识别API
使用SpeechRecognition库,可以轻松调用不同的语音识别API。以下是一个使用Google Web Speech API的例子:
import speech_recognition as sr
def recognize_speech_from_file(file_path):
recognizer = sr.Recognizer()
with sr.AudioFile(file_path) as source:
audio = recognizer.record(source) # Read the entire audio file
try:
# Use the Google Web Speech API
text = recognizer.recognize_google(audio)
print(f"Recognized text: {text}")
except sr.UnknownValueError:
print("Google Speech Recognition could not understand the audio")
except sr.RequestError as e:
print(f"Could not request results from Google Speech Recognition service; {e}")
recognize_speech_from_file('output.wav')
在这里,recognize_google
方法将音频文件中的语音转换为文本。
五、解析识别结果
语音识别的最终步骤是解析识别结果。通常,识别引擎会返回一个文本字符串,您可以根据应用的需求进行处理。例如,可以将识别的文本存储在数据库中,或用于自然语言处理任务。
以下是一个解析和处理识别结果的例子:
def process_recognized_text(text):
# 简单地将识别文本输出
print("Processed text:", text)
在识别完成后调用
recognized_text = recognize_speech_from_file('output.wav')
process_recognized_text(recognized_text)
在这个例子中,识别文本被简单地打印输出,但在实际应用中,您可能会将其用于更复杂的任务。
通过以上步骤,您可以在Python中实现一个基本的语音识别系统。根据具体的项目需求,您还可以探索其他语音识别引擎或库,如Microsoft Azure Speech、IBM Watson Speech to Text或Mozilla DeepSpeech,以获得更高的准确率或特定功能。
相关问答FAQs:
如何在Python中进行语音识别的基本步骤是什么?
在Python中实现语音识别的基本步骤通常包括安装所需的库、设置音频输入源、使用语音识别模块进行处理。常用的库包括SpeechRecognition和pyaudio。首先,确保安装这些库,然后通过编写代码来捕捉音频并将其转换为文本。
使用Python进行语音识别时,有哪些常用的库和工具?
在Python中,有几个流行的库可以用于语音识别。其中最常用的是SpeechRecognition,它支持多种识别引擎和API。此外,pyaudio库用于捕获音频流,Google的Web Speech API也是一个受欢迎的选择。其他选择包括CMU Sphinx和Microsoft Azure的语音服务。
如何提高Python语音识别的准确性?
提高语音识别准确性的方法包括使用高质量的麦克风,确保环境安静,调整语音识别模型的参数,以及在需要时使用特定领域的语言模型。此外,进行适当的音频预处理(如去噪)也能显著提升识别效果。定期更新模型和使用自定义词汇表也是有助于提高准确性的策略。
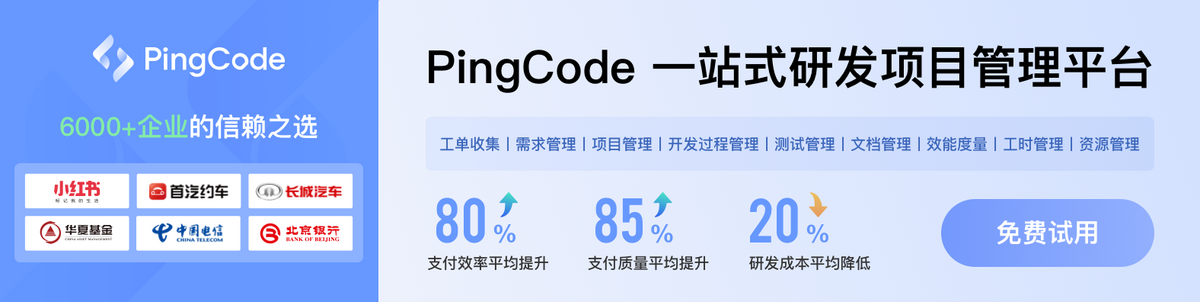