Python检索文件内容的方法包括使用内置函数open()、利用正则表达式、通过外部库如pandas进行数据处理。在这其中,open()函数是最基础且常用的方法,它允许我们以多种模式打开文件并读取内容;正则表达式则提供了一种强大的方式来搜索和匹配文件中的特定模式;而pandas库适用于处理结构化数据文件,如CSV或Excel文件,能够高效地进行数据筛选和检索。
一、OPEN()函数读取文件
Python的内置函数open()
是读取文件内容的基础工具。它具有不同的模式,可以根据需要选择读取文本或二进制数据。
1.1、基本用法
使用open()
函数读取文件内容的基本步骤包括打开文件、读取内容、关闭文件。以下是一个简单的例子:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在这个例子中,'r'
表示以只读模式打开文件。with
语句用于确保文件在使用后被正确关闭。
1.2、按行读取
有时候,我们可能只需要逐行读取文件。这可以通过readline()
或readlines()
方法实现:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
使用strip()
可以去除每行末尾的换行符。
二、正则表达式搜索
正则表达式是一个强大的工具,用于在文本中搜索特定的模式。Python的re
模块提供了正则表达式功能。
2.1、简单匹配
假设我们需要在文件中查找特定的单词:
import re
pattern = r'\bword\b'
with open('example.txt', 'r') as file:
content = file.read()
matches = re.findall(pattern, content)
print(f"Found {len(matches)} matches.")
在这个例子中,\b
是一个单词边界,确保我们匹配的是整个单词而不是单词的一部分。
2.2、复杂模式
我们也可以使用正则表达式匹配更复杂的模式,比如电子邮件地址、电话号码等。
pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
with open('example.txt', 'r') as file:
content = file.read()
emails = re.findall(pattern, content)
print(f"Found emails: {emails}")
三、使用PANDAS库
对于结构化数据文件,pandas
库提供了强大的数据处理能力。
3.1、读取CSV文件
CSV文件是常见的数据文件格式,pandas
提供了便捷的读取方法:
import pandas as pd
df = pd.read_csv('data.csv')
print(df.head())
这个例子中,pd.read_csv()
读取CSV文件并返回一个DataFrame
对象,head()
方法用于显示前几行数据。
3.2、筛选数据
pandas
允许我们方便地筛选数据。例如,我们可以根据某一列的值进行筛选:
filtered_data = df[df['column_name'] == 'value']
print(filtered_data)
这种方法非常适合用于大型数据集的检索和分析。
四、通过OS模块遍历文件
当需要在多个文件中检索内容时,Python的os
模块可以帮助我们遍历目录。
4.1、遍历文件目录
使用os.walk()
可以遍历目录中的所有文件:
import os
for root, dirs, files in os.walk('/path/to/directory'):
for file in files:
if file.endswith('.txt'):
with open(os.path.join(root, file), 'r') as f:
content = f.read()
# Process the file content
这个例子中,我们遍历指定目录下的所有文本文件,并读取其内容。
4.2、文件过滤
可以通过条件过滤特定文件类型或名称:
for root, dirs, files in os.walk('/path/to/directory'):
for file in files:
if 'specific_word' in file:
# Do something with the file
五、多线程和异步读取
在处理大量文件或大文件时,多线程和异步读取可以提高效率。
5.1、多线程读取
使用threading
模块可以在多个线程中并行读取文件:
import threading
def read_file(filename):
with open(filename, 'r') as file:
content = file.read()
# Process content
threads = []
for i in range(10): # Assume we have 10 files
t = threading.Thread(target=read_file, args=(f'file{i}.txt',))
threads.append(t)
t.start()
for t in threads:
t.join()
5.2、异步读取
异步IO可以使用asyncio
和aiofiles
库:
import asyncio
import aiofiles
async def read_file(filename):
async with aiofiles.open(filename, 'r') as file:
content = await file.read()
# Process content
async def main():
tasks = [read_file(f'file{i}.txt') for i in range(10)]
await asyncio.gather(*tasks)
asyncio.run(main())
使用异步IO可以避免阻塞,提高文件读取效率。
六、总结
通过以上各种方法,Python提供了灵活而强大的工具来检索文件内容。无论是简单的文本文件还是复杂的结构化数据文件,都可以通过合适的方法实现高效的检索和处理。在选择具体方法时,应根据具体需求和数据规模来确定,以达到最佳性能和结果。
相关问答FAQs:
如何使用Python读取文件内容?
使用Python读取文件内容非常简单。可以使用内置的open()
函数打开文件,然后使用read()
、readline()
或readlines()
方法读取内容。例如:
with open('文件路径.txt', 'r') as file:
content = file.read()
print(content)
通过这种方式,你可以轻松获取整个文件的内容。
Python中有哪些方法可以搜索文件中的特定文本?
在Python中,可以使用in
关键字结合文件内容进行搜索。先读取文件内容,然后检查特定字符串是否在内容中。例如:
with open('文件路径.txt', 'r') as file:
content = file.read()
if '要搜索的文本' in content:
print('找到文本')
此外,使用正则表达式模块re
也可以进行更复杂的搜索。
如何在Python中逐行检索文件内容?
逐行检索文件内容可以使用for
循环逐行读取文件。这样可以节省内存,并适用于大文件。例如:
with open('文件路径.txt', 'r') as file:
for line in file:
if '要搜索的文本' in line:
print('找到文本:', line)
这种方法可以帮助你逐行处理文件数据,并找到需要的信息。
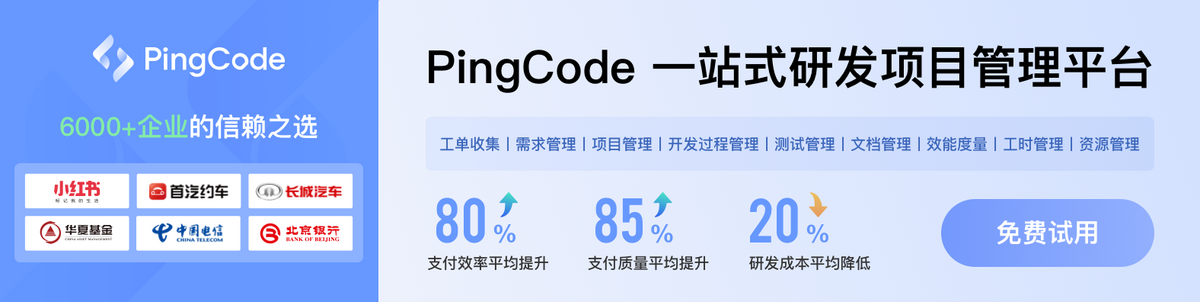