要在Python中创建一个骰子模拟,可以使用随机数生成、定义函数和控制循环等方法。随机数生成、定义函数和控制循环是实现骰子模拟的关键。 下面将详细介绍如何在Python中实现一个简单的骰子模拟。
一、随机数生成
在模拟骰子时,随机数生成是一个核心部分。Python的random
模块提供了生成随机数的功能,这使得我们可以轻松地模拟骰子掷出的结果。
- 使用
random.randint()
函数
random.randint(a, b)
函数可以生成一个包含在区间[a, b]内的随机整数。对于一个6面的骰子,我们可以使用random.randint(1, 6)
来模拟一次掷骰子的结果。
import random
def roll_dice():
return random.randint(1, 6)
在这个代码段中,我们定义了一个名为roll_dice
的函数,它每次调用时都会返回一个1到6之间的随机整数,模拟掷骰子的结果。
- 随机种子控制
有时候我们需要控制随机数生成器,以便在需要时重现相同的随机序列。这可以通过random.seed()
函数来实现。
import random
random.seed(0) # 设置随机种子
print(roll_dice())
通过设置相同的种子,roll_dice()
函数将每次生成相同的结果序列。这在调试和测试中非常有用。
二、定义函数
为了提高代码的可读性和重用性,我们可以将骰子的模拟过程封装到一个函数中。这样不仅能够简化主程序的复杂度,还能使代码结构更加清晰。
- 创建一个完整的骰子模拟函数
我们可以创建一个函数,它能够模拟多次掷骰子,并返回每次掷骰子的结果。
def roll_multiple_dice(num_rolls):
results = []
for _ in range(num_rolls):
results.append(roll_dice())
return results
在这个函数中,我们传入一个参数num_rolls
,表示我们希望模拟掷骰子的次数。函数内部使用一个循环来调用roll_dice
函数,并将结果存储在一个列表中,最终返回所有结果。
- 结果分析功能
除了简单的模拟掷骰子结果,我们还可以增加一些功能来分析这些结果。例如,我们可以计算每个数字出现的频率。
def analyze_results(results):
frequency = {i: results.count(i) for i in range(1, 7)}
return frequency
这个函数接收一个包含多个掷骰子结果的列表results
,并返回一个字典,其中键是骰子的面值,值是该面值在结果中出现的次数。
三、控制循环
在实现一个骰子模拟程序时,控制循环的使用可以帮助我们实现重复操作和用户交互。
- 使用循环实现用户交互
我们可以设计一个程序,使用户可以选择是否继续掷骰子。
def main():
while True:
num_rolls = int(input("Enter the number of dice rolls: "))
results = roll_multiple_dice(num_rolls)
print("Results:", results)
print("Analysis:", analyze_results(results))
cont = input("Do you want to roll again? (y/n): ")
if cont.lower() != 'y':
break
if __name__ == "__main__":
main()
在这个程序中,我们使用一个while
循环不断提示用户输入要模拟的掷骰子次数,并输出结果和分析。如果用户选择不再继续,循环终止,程序结束。
- 添加异常处理
在用户输入的过程中,可能会出现错误输入(例如输入非整数的情况)。我们可以通过异常处理来增强程序的健壮性。
def main():
while True:
try:
num_rolls = int(input("Enter the number of dice rolls: "))
results = roll_multiple_dice(num_rolls)
print("Results:", results)
print("Analysis:", analyze_results(results))
except ValueError:
print("Please enter a valid number.")
continue
cont = input("Do you want to roll again? (y/n): ")
if cont.lower() != 'y':
break
if __name__ == "__main__":
main()
通过在输入部分加入try-except
块,我们可以捕获用户输入的异常情况,并提示用户重新输入正确的值。
四、扩展功能
在完成基本的骰子模拟功能后,我们可以考虑添加一些扩展功能,以增强程序的实用性和趣味性。
- 多面骰子支持
除了传统的6面骰子,我们还可以支持模拟其他面数的骰子,比如4面、8面、12面等。
def roll_custom_dice(sides):
return random.randint(1, sides)
def roll_multiple_custom_dice(sides, num_rolls):
results = []
for _ in range(num_rolls):
results.append(roll_custom_dice(sides))
return results
在这个扩展中,我们定义了roll_custom_dice
函数,它接收一个参数sides
,用于指定骰子的面数。然后,我们可以通过roll_multiple_custom_dice
函数来模拟多次掷这种自定义面数的骰子。
- 图形化结果展示
为了更直观地展示结果,我们可以使用Python的图形库(如matplotlib
)绘制结果的柱状图。
import matplotlib.pyplot as plt
def plot_results(frequency):
faces = list(frequency.keys())
counts = list(frequency.values())
plt.bar(faces, counts, tick_label=faces)
plt.xlabel('Dice Face')
plt.ylabel('Frequency')
plt.title('Dice Roll Results')
plt.show()
在这个扩展中,我们定义了plot_results
函数,接收一个频率字典作为输入,并使用matplotlib
库绘制柱状图。这可以帮助用户更直观地理解结果分布。
- 保存结果到文件
有时候,我们可能需要将模拟结果保存到文件中,以便进行进一步分析或记录。
def save_results_to_file(results, filename='dice_results.txt'):
with open(filename, 'w') as file:
for result in results:
file.write(str(result) + '\n')
在这个扩展中,我们定义了save_results_to_file
函数,它接收一个结果列表和一个可选的文件名参数,将结果逐行写入指定的文件中。
通过这些步骤,我们可以在Python中创建一个功能丰富的骰子模拟程序。这不仅可以帮助我们理解随机数生成和函数定义的基本原理,还可以通过扩展功能来提高程序的实用性和趣味性。
相关问答FAQs:
如何使用Python模拟骰子的投掷过程?
在Python中,可以使用random
模块来模拟骰子的投掷。你可以通过调用random.randint(1, 6)
函数来生成一个1到6之间的随机整数,代表骰子的点数。以下是一个简单的示例代码:
import random
def roll_dice():
return random.randint(1, 6)
# 投掷骰子并打印结果
result = roll_dice()
print(f"你投掷的骰子点数是: {result}")
如何在Python中创建多个骰子投掷的功能?
如果你想要一次投掷多个骰子,可以修改函数以接受骰子的数量参数。以下是一个示例:
def roll_multiple_dice(num_dice):
results = [random.randint(1, 6) for _ in range(num_dice)]
return results
# 投掷3个骰子并打印结果
results = roll_multiple_dice(3)
print(f"你投掷的骰子点数是: {results}")
这种方式可以灵活地设置投掷的骰子数量。
如何在Python中显示骰子图形?
为了让骰子的展示更加生动,你可以使用ASCII艺术或图形库(如matplotlib
)来显示骰子的点数。以下是一个使用ASCII艺术的简单示例:
def display_dice_face(value):
dice_faces = {
1: "-----\n| |\n| o |\n| |\n-----",
2: "-----\n|o |\n| |\n| o|\n-----",
3: "-----\n|o |\n| o |\n| o|\n-----",
4: "-----\n|o o|\n| |\n|o o|\n-----",
5: "-----\n|o o|\n| o |\n|o o|\n-----",
6: "-----\n|o o|\n|o o|\n|o o|\n-----"
}
return dice_faces[value]
# 显示骰子面
dice_value = roll_dice()
print(display_dice_face(dice_value))
以上代码可以根据投掷的结果展示对应的骰子面。
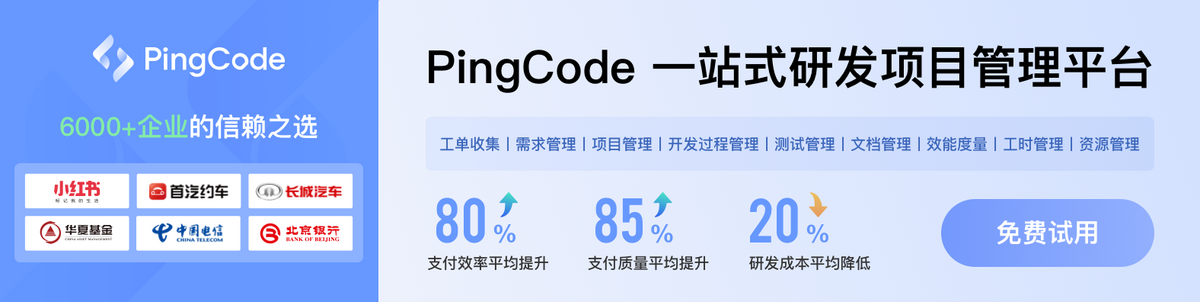