在Python中,修改远程文件可以通过多种方式实现,常用的方法包括使用SSH连接进行远程文件编辑、使用FTP/SFTP协议上传和下载文件、利用RESTful API与远程服务器进行交互。其中,SSH连接是一种非常常见的方法,因为它提供了安全的加密通信。对于需要频繁修改的文件,使用API可能是更优的选择,因为它可以更好地集成到现有的系统中。下面将详细介绍使用SSH连接和API进行远程文件修改的方法。
一、SSH连接进行远程文件修改
SSH(Secure Shell)是一种加密网络协议,用于在不安全的网络上安全地操作网络服务。通过SSH,我们可以远程登录到服务器,并使用Python的paramiko库执行文件修改操作。
1. 使用paramiko库进行SSH连接
Paramiko是一个用于在Python中实现SSH2协议的模块,它可以让我们通过Python脚本远程执行命令和文件操作。
import paramiko
def modify_remote_file_via_ssh(hostname, port, username, password, remote_file_path, content_to_add):
# 创建SSH客户端
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# 连接到远程服务器
ssh.connect(hostname, port, username, password)
# 使用SFTP打开远程文件
sftp = ssh.open_sftp()
remote_file = sftp.open(remote_file_path, 'a')
# 写入新内容
remote_file.write(content_to_add)
remote_file.flush()
# 关闭文件和连接
remote_file.close()
sftp.close()
ssh.close()
使用示例
hostname = 'your.remote.server'
port = 22
username = 'your_username'
password = 'your_password'
remote_file_path = '/path/to/remote/file.txt'
content_to_add = '\nNew content to add.'
modify_remote_file_via_ssh(hostname, port, username, password, remote_file_path, content_to_add)
2. 安全性和配置
在使用SSH进行远程文件修改时,需要注意以下几点:
- 安全性:确保SSH密钥和密码的安全性,避免泄露。
- 权限:确保远程文件具有可写权限。
- 连接管理:合理管理连接和断开,避免资源泄露。
- 错误处理:添加必要的错误处理代码,以便在连接失败或文件无法打开时进行适当的处理。
二、通过API进行远程文件修改
在某些情况下,远程服务器可能会提供RESTful API用于文件操作,这种方式通常用于Web服务中。RESTful API可以让我们更灵活地操作文件,并且易于与其他系统集成。
1. 使用requests库调用API
通过requests库,我们可以轻松地向服务器发送HTTP请求以修改远程文件。以下是假设服务器提供了一个用于文件修改的API端点的示例:
import requests
def modify_remote_file_via_api(api_endpoint, file_id, new_content):
# 构建请求数据
data = {
'file_id': file_id,
'new_content': new_content
}
# 发送PUT请求以修改文件
response = requests.put(api_endpoint, json=data)
# 检查响应状态
if response.status_code == 200:
print('File modified successfully.')
else:
print('Failed to modify file:', response.text)
使用示例
api_endpoint = 'https://your.api.endpoint/files'
file_id = '12345'
new_content = 'Updated file content.'
modify_remote_file_via_api(api_endpoint, file_id, new_content)
2. 设计API的注意事项
- 认证和授权:确保API请求经过适当的认证和授权,以保护文件的安全性。
- 幂等性:设计API时,应确保PUT请求的幂等性,以便多次重复请求不会造成不一致。
- 错误处理:在API设计中,提供详细的错误信息和状态码,以便客户端能够正确处理错误。
三、使用FTP/SFTP协议进行文件修改
除了SSH和API,FTP(文件传输协议)和SFTP(SSH文件传输协议)也是常用的文件传输协议,可以用于远程文件的上传、下载和修改。
1. 使用ftplib库进行FTP连接
from ftplib import FTP
def modify_remote_file_via_ftp(hostname, username, password, remote_file_path, content_to_add):
# 连接到FTP服务器
ftp = FTP(hostname)
ftp.login(user=username, passwd=password)
# 下载文件并读取内容
lines = []
ftp.retrlines(f'RETR {remote_file_path}', lines.append)
lines.append(content_to_add)
# 上传修改后的文件
with open('temp_file.txt', 'w') as f:
f.write('\n'.join(lines))
with open('temp_file.txt', 'rb') as f:
ftp.storlines(f'STOR {remote_file_path}', f)
# 关闭连接
ftp.quit()
使用示例
hostname = 'your.ftp.server'
username = 'your_username'
password = 'your_password'
remote_file_path = '/path/to/remote/file.txt'
content_to_add = 'Additional content.'
modify_remote_file_via_ftp(hostname, username, password, remote_file_path, content_to_add)
2. 使用pysftp库进行SFTP连接
import pysftp
def modify_remote_file_via_sftp(hostname, username, password, remote_file_path, content_to_add):
# 设置连接选项
cnopts = pysftp.CnOpts()
cnopts.hostkeys = None
# 连接到SFTP服务器
with pysftp.Connection(hostname, username=username, password=password, cnopts=cnopts) as sftp:
# 下载文件并读取内容
with sftp.open(remote_file_path, 'r') as remote_file:
lines = remote_file.readlines()
# 添加新内容
lines.append(content_to_add + '\n')
# 上传修改后的文件
with sftp.open(remote_file_path, 'w') as remote_file:
remote_file.writelines(lines)
使用示例
hostname = 'your.sftp.server'
username = 'your_username'
password = 'your_password'
remote_file_path = '/path/to/remote/file.txt'
content_to_add = 'SFTP additional content.'
modify_remote_file_via_sftp(hostname, username, password, remote_file_path, content_to_add)
四、总结
在Python中修改远程文件可以通过SSH、API和FTP/SFTP等多种方式实现。选择合适的方法取决于具体的需求和环境。SSH适用于需要安全加密的命令行操作,API适用于Web服务的集成,而FTP/SFTP则适用于传统的文件传输。无论哪种方法,都应注意安全性、权限管理和错误处理,以确保操作的可靠性和安全性。
相关问答FAQs:
如何通过Python远程修改文件?
要通过Python远程修改文件,您可以使用SSH协议和一些流行的库,如Paramiko。Paramiko允许您连接到远程服务器,并使用SFTP或执行命令来编辑文件。您需要确保已安装Paramiko库,并且有权限访问远程服务器。
在远程服务器上修改文件时需要注意哪些安全措施?
在进行远程文件修改时,确保使用强密码或SSH密钥进行身份验证,以保护您的服务器免受未经授权的访问。此外,考虑在防火墙中限制对SSH端口的访问,确保仅允许特定IP地址连接。定期更新您的系统和软件以修补安全漏洞也是非常重要的。
可以使用哪些Python库来处理远程文件修改?
除了Paramiko,您还可以使用其他库,如Fabric和Plumbum。这些库提供了高层次的接口,简化了远程操作的过程。Fabric专注于执行命令和文件传输,而Plumbum则以更轻量的方式提供命令行和远程操作的功能。
如果遇到权限错误,该如何解决?
在尝试修改远程文件时,如果出现权限错误,首先需要检查当前用户是否具有适当的文件访问权限。您可能需要使用chmod
命令更改文件权限,或者联系系统管理员以获取所需的权限。此外,确保您以正确的用户身份连接到远程服务器,以避免权限不足的问题。
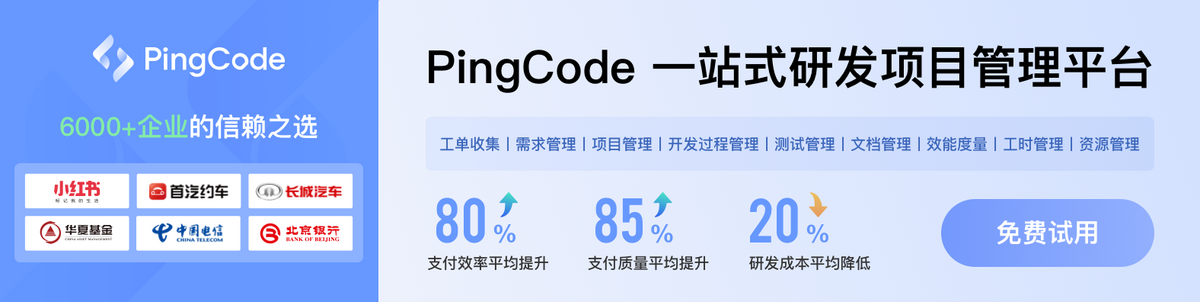