在Python中删除目录可以通过多种方式实现,最常见的方法包括使用os
模块和shutil
模块。使用os模块中的rmdir函数删除空目录、使用shutil模块中的rmtree函数递归删除非空目录、确保目标目录存在是删除目录前的重要一步。下面将详细介绍这些方法及其使用场景。
一、使用OS模块删除空目录
os
模块是Python标准库的一部分,它提供了与操作系统交互的功能。要删除一个空目录,可以使用os.rmdir()
函数。
1. 使用os.rmdir()函数
os.rmdir(path)
用于删除指定路径的空目录。如果目录不为空,调用此函数将引发OSError
。
import os
def remove_empty_directory(dir_path):
try:
os.rmdir(dir_path)
print(f"Successfully removed the directory: {dir_path}")
except OSError as e:
print(f"Error: {dir_path} : {e.strerror}")
示例
remove_empty_directory('/path/to/empty/directory')
在使用os.rmdir()
时,需要确保目标目录是空的,否则会引发错误。对于这种情况,可以使用os.listdir()
检查目录内容。
二、使用SHUTIL模块递归删除非空目录
shutil
模块提供了更高级的文件操作功能,包括递归删除目录。对于包含文件或子目录的非空目录,可以使用shutil.rmtree()
函数。
1. 使用shutil.rmtree()函数
shutil.rmtree(path)
用于递归删除目录及其所有内容,包括文件和子目录。
import shutil
def remove_directory_recursively(dir_path):
try:
shutil.rmtree(dir_path)
print(f"Successfully removed the directory and all its contents: {dir_path}")
except Exception as e:
print(f"Error: {e}")
示例
remove_directory_recursively('/path/to/non-empty/directory')
shutil.rmtree()
功能强大,适用于删除包含多层嵌套内容的目录。但在使用时需要谨慎,以免误删重要数据。
三、确保目录存在
在删除目录之前,最好检查目录是否存在,以避免不必要的错误。这可以通过os.path.exists()
函数实现。
1. 使用os.path.exists()检查目录
import os
import shutil
def safe_remove_directory(dir_path):
if os.path.exists(dir_path):
try:
shutil.rmtree(dir_path)
print(f"Successfully removed the directory: {dir_path}")
except Exception as e:
print(f"Error: {e}")
else:
print(f"The directory does not exist: {dir_path}")
示例
safe_remove_directory('/path/to/check/directory')
通过以上方法,可以更加安全和有效地删除目录。
四、处理删除过程中的异常
在删除目录时,可能会遇到各种异常情况,例如文件权限问题、文件正在使用等。因此,在编写删除目录的代码时,需要考虑这些异常并进行适当处理。
1. 捕获和处理异常
在使用os.rmdir()
或shutil.rmtree()
时,可以通过try-except
块捕获异常并进行相应处理。
import os
import shutil
def robust_remove_directory(dir_path):
try:
if os.path.exists(dir_path):
shutil.rmtree(dir_path)
print(f"Successfully removed the directory: {dir_path}")
else:
print(f"The directory does not exist: {dir_path}")
except PermissionError as pe:
print(f"Permission denied: {dir_path} : {pe}")
except FileNotFoundError as fnfe:
print(f"File not found: {dir_path} : {fnfe}")
except Exception as e:
print(f"An error occurred: {e}")
示例
robust_remove_directory('/path/to/handle/exceptions')
通过这种方式,可以提高代码的健壮性,并为用户提供更详细的错误信息。
五、删除目录的实际应用场景
删除目录的操作在实际应用中非常常见,以下是一些常见的应用场景:
1. 清理临时文件
在数据处理和文件操作中,经常需要创建临时文件和目录。处理完成后,删除这些临时文件和目录以释放存储空间。
import tempfile
import os
import shutil
def clean_temp_files():
temp_dir = tempfile.mkdtemp()
# 在这里进行一些操作
# 操作完成后删除临时目录
shutil.rmtree(temp_dir)
print(f"Temporary directory {temp_dir} removed")
示例
clean_temp_files()
2. 自动化脚本中的目录管理
在自动化脚本中,常常需要在每次运行前清理旧的输出目录,以确保每次运行时得到干净的输出。
import os
import shutil
def prepare_output_directory(output_dir):
if os.path.exists(output_dir):
shutil.rmtree(output_dir)
os.makedirs(output_dir)
print(f"Prepared a clean output directory: {output_dir}")
示例
prepare_output_directory('/path/to/output/directory')
通过这些示例,可以看到删除目录在实际开发中的重要性和应用广泛性。确保在删除目录时,做好检查和异常处理,以避免不必要的数据丢失和错误发生。
相关问答FAQs:
如何在Python中删除一个非空目录?
在Python中,可以使用shutil
模块中的rmtree()
函数来删除一个非空目录。该函数会递归地删除目录及其所有内容。示例代码如下:
import shutil
shutil.rmtree('your_directory_path')
确保在使用此方法时,目录中的所有数据都会被永久删除,因此在执行此操作之前务必备份重要文件。
使用Python删除目录时需要注意哪些权限问题?
在删除目录之前,确保你有足够的权限来执行该操作。如果你尝试删除一个你没有权限访问的目录,Python会抛出权限错误。可以通过更改目录权限或以管理员身份运行脚本来解决此问题。
如何安全地删除目录以避免误删文件?
为避免误删文件,可以在删除之前先检查目录是否为空。可以使用os.listdir()
函数来列出目录中的所有文件和子目录,如果返回的列表为空,则可以安全地删除。例如:
import os
import shutil
directory = 'your_directory_path'
if not os.listdir(directory):
os.rmdir(directory) # 仅删除空目录
else:
print("目录不为空,无法删除。")
这种方式可以确保你只删除那些不再需要的空目录。
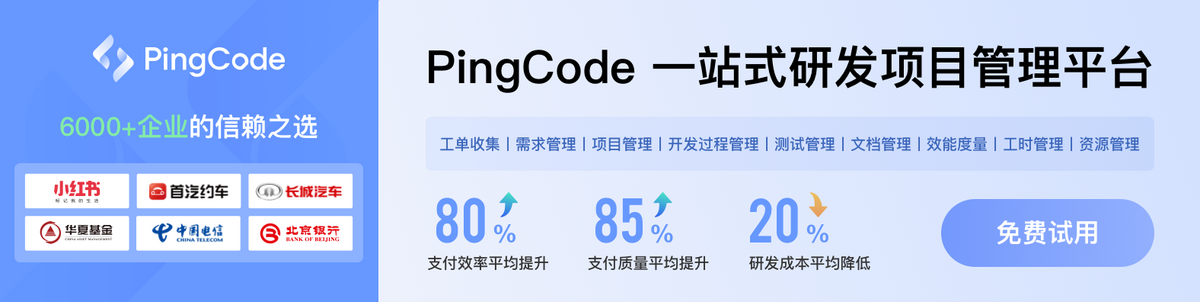