在Python中访问类变量的方法包括:使用类名直接访问、通过类实例访问、在类方法中通过cls
访问、在实例方法中通过self
访问。使用类名直接访问类变量是最常见的方法,它确保访问的是类变量而不是实例变量。通过类实例访问类变量尽管可行,但不推荐,因为容易引起混淆。接下来,将详细阐述通过类名直接访问类变量的方法。
使用类名直接访问类变量是一种明确且有效的方式。这种方法的优点是代码可读性高,能够清楚地区分类变量和实例变量。类变量通常用于需要在类的所有实例之间共享数据的场景,例如计数器、常量等。当使用类名访问类变量时,代码会在类的命名空间中查找变量,确保所访问的是类变量而非实例变量。以下是一个简单的示例:
class MyClass:
class_variable = "Hello, World!"
使用类名直接访问类变量
print(MyClass.class_variable)
在这段代码中,通过MyClass.class_variable
直接访问了类变量class_variable
。这种方法非常直观,确保了代码的可读性和可维护性。
接下来,我们将深入探讨Python中访问类变量的不同方法和场景。
一、类变量与实例变量的区别
在深入讨论如何访问类变量之前,了解类变量和实例变量之间的区别非常重要。
1. 类变量
类变量是在类定义中声明的变量,其值对于该类的所有实例是共享的。这意味着如果类变量的值被改变,这一变化将在所有实例中可见。类变量通常用于存储与类本身相关的数据。
class Example:
class_variable = 0 # 类变量
def __init__(self):
Example.class_variable += 1
创建多个实例,类变量会被共享和修改
instance1 = Example()
instance2 = Example()
print(Example.class_variable) # 输出 2
2. 实例变量
实例变量是在类的实例化过程中(通常在__init__
方法中)创建的变量,它们的值对于不同的实例是独立的。
class Example:
def __init__(self, value):
self.instance_variable = value # 实例变量
instance1 = Example(1)
instance2 = Example(2)
print(instance1.instance_variable) # 输出 1
print(instance2.instance_variable) # 输出 2
二、通过类名访问类变量
使用类名访问类变量是一种推荐的方式,因为它直接且不易混淆。如下所示:
class MyClass:
class_variable = "Hello, Python!"
print(MyClass.class_variable)
这种方法的优点在于明确性,代码的意图很清晰:我们正在访问一个类变量。
三、通过实例访问类变量
虽然可以通过实例访问类变量,但这种方式不推荐,因为它可能导致混淆,尤其是在实例变量与类变量名称相同时。
class MyClass:
class_variable = "Hello, Python!"
instance = MyClass()
print(instance.class_variable) # 可以访问,但不推荐
这种方式的潜在问题在于,如果实例中定义了同名的实例变量,会导致访问的混淆。
四、在类方法中访问类变量
类方法使用@classmethod
装饰器定义,通常用于操作类变量。类方法的第一个参数是cls
,它指代类本身。
class MyClass:
class_variable = "Hello, Python!"
@classmethod
def get_class_variable(cls):
return cls.class_variable
print(MyClass.get_class_variable())
在类方法中,使用cls.class_variable
访问类变量是非常自然的,这种方式也确保了代码的一致性和可读性。
五、在实例方法中访问类变量
在实例方法中,可以通过self.__class__
访问类变量。不过,这种方式不如直接使用类名那么清晰。
class MyClass:
class_variable = "Hello, Python!"
def get_class_variable(self):
return self.__class__.class_variable
instance = MyClass()
print(instance.get_class_variable())
这种方法虽然可行,但不如直接使用类名访问来得直观。
六、类变量的使用场景
类变量在一些特定的场景下非常有用,例如:
1. 计数实例
可以使用类变量来跟踪一个类创建了多少实例。
class Example:
instance_count = 0
def __init__(self):
Example.instance_count += 1
print(Example.instance_count) # 输出 0
instance1 = Example()
instance2 = Example()
print(Example.instance_count) # 输出 2
2. 常量定义
类变量可以用来定义常量,这些常量在整个应用程序中是共享的。
class MathConstants:
PI = 3.14159
E = 2.71828
print(MathConstants.PI)
print(MathConstants.E)
七、类变量的修改与继承
在类继承的情况下,子类会继承父类的类变量。如果在子类中修改类变量,修改将仅限于子类,不会影响父类。
class Parent:
class_variable = "Parent"
class Child(Parent):
pass
print(Parent.class_variable) # 输出 "Parent"
print(Child.class_variable) # 输出 "Parent"
Child.class_variable = "Child"
print(Parent.class_variable) # 输出 "Parent"
print(Child.class_variable) # 输出 "Child"
这种行为允许在子类中自定义类变量,而不会影响父类的定义。
八、类变量的线程安全问题
在多线程环境中,类变量的修改可能会引发线程安全问题。为避免此类问题,通常需要使用线程锁来同步对类变量的访问。
import threading
class ThreadSafeExample:
class_variable = 0
lock = threading.Lock()
@classmethod
def increment(cls):
with cls.lock:
cls.class_variable += 1
在线程中安全地修改类变量
threads = []
for _ in range(10):
thread = threading.Thread(target=ThreadSafeExample.increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(ThreadSafeExample.class_variable) # 输出 10
在这个示例中,使用锁确保了对类变量的安全修改,避免了数据竞争。
九、总结
类变量在Python中提供了一种共享数据的机制,可以用于多种场景。通过类名访问类变量是最推荐的方法,因为它清晰且不易混淆。在多线程环境中,注意类变量的修改可能导致线程安全问题,应该使用适当的同步机制。理解类变量的使用和限制有助于编写更清晰和高效的Python代码。
相关问答FAQs:
如何在Python中创建类变量?
在Python中,类变量是定义在类体内但在任何方法之外的变量。要创建类变量,只需在类的定义中赋值即可。例如:
class MyClass:
class_variable = 0 # 这是一个类变量
def increment_class_variable(self):
MyClass.class_variable += 1
在这个例子中,class_variable
就是一个类变量,所有类的实例都共享这一变量。
访问类变量时需要注意什么?
访问类变量时,可以通过类名或实例来访问。使用类名访问时,推荐的方式是 MyClass.class_variable
,这样可以清晰地表明它是一个类变量。虽然也可以通过实例访问,例如instance.class_variable
,但这种方式可能会引起混淆,特别是在类变量和实例变量同名时。
如何修改类变量的值?
要修改类变量的值,可以直接通过类名进行赋值,也可以通过实例的方法来修改。例如:
class MyClass:
class_variable = 0
@classmethod
def update_class_variable(cls, value):
cls.class_variable = value
MyClass.update_class_variable(5) # 修改类变量的值
使用类方法可以有效地修改类变量,并确保在所有实例中反映这一变化。
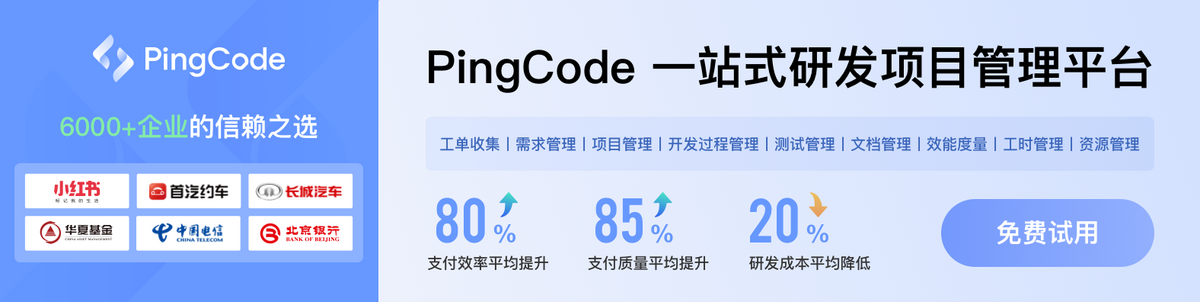