Python批量创建目录的主要方法包括:使用os模块、使用pathlib模块、结合异常处理以确保目录安全创建。在这里,我们将详细探讨如何在Python中高效地批量创建目录。
一、OS模块批量创建目录
os模块是Python标准库的一部分,提供了许多与操作系统进行交互的功能。使用os模块,我们可以轻松地创建目录。
- 使用os.makedirs()创建目录
os.makedirs()函数允许我们递归地创建目录。这意味着如果父目录不存在,该函数会自动创建。
import os
def create_directories(dir_list):
for dir_path in dir_list:
try:
os.makedirs(dir_path, exist_ok=True)
print(f"Directory {dir_path} created successfully")
except Exception as e:
print(f"An error occurred while creating directory {dir_path}: {e}")
示例目录列表
directories = ["./data/2023/jan", "./data/2023/feb", "./data/2023/mar"]
create_directories(directories)
在上述代码中,我们定义了一个create_directories函数,它接收一个目录路径列表,并使用os.makedirs()创建目录。exist_ok=True参数确保如果目录已存在,不会引发异常。
- 检查目录是否存在
在创建目录之前,检查目录是否已存在是一个良好的编程实践。这可以防止不必要的错误。
def create_directories_check(dir_list):
for dir_path in dir_list:
if not os.path.exists(dir_path):
os.makedirs(dir_path)
print(f"Directory {dir_path} created successfully")
else:
print(f"Directory {dir_path} already exists")
通过os.path.exists()函数,我们可以检查目录是否存在,从而避免重复创建。
二、PATHLIB模块批量创建目录
pathlib模块是Python 3.4引入的,用于处理文件系统路径的面向对象的模块。它提供了比os模块更优雅的方式来处理路径。
- 使用Path.mkdir()创建目录
pathlib模块中的Path类提供了mkdir()方法,可以用于创建目录。
from pathlib import Path
def create_directories_pathlib(dir_list):
for dir_path in dir_list:
path = Path(dir_path)
try:
path.mkdir(parents=True, exist_ok=True)
print(f"Directory {dir_path} created successfully")
except Exception as e:
print(f"An error occurred while creating directory {dir_path}: {e}")
示例目录列表
directories = ["./data/2023/apr", "./data/2023/may", "./data/2023/jun"]
create_directories_pathlib(directories)
Path.mkdir()方法的parents=True参数允许创建多级目录,exist_ok=True参数则防止已存在的目录引发异常。
- 结合异常处理
在使用pathlib时,也可以结合异常处理来确保目录创建过程的安全性。
def create_directories_with_exception_handling(dir_list):
for dir_path in dir_list:
path = Path(dir_path)
if not path.exists():
try:
path.mkdir(parents=True)
print(f"Directory {dir_path} created successfully")
except Exception as e:
print(f"An error occurred while creating directory {dir_path}: {e}")
else:
print(f"Directory {dir_path} already exists")
通过这种方式,我们可以更好地处理目录创建过程中可能发生的各种异常,确保程序的鲁棒性。
三、结合文件读取批量创建目录
在实际应用中,目录路径可能存储在文件中。我们可以读取这些文件,并使用上述方法批量创建目录。
def create_directories_from_file(file_path):
with open(file_path, 'r') as file:
dir_list = file.readlines()
dir_list = [dir_path.strip() for dir_path in dir_list]
create_directories(dir_list)
假设目录路径存储在'directories.txt'文件中
create_directories_from_file('directories.txt')
通过读取文件中的目录路径,可以轻松实现批量目录创建。这对于需要动态管理大量目录的项目尤为有用。
四、总结与最佳实践
在Python中批量创建目录的过程中,选择合适的方法和模块是关键。以下是一些最佳实践:
- 使用pathlib模块:对于Python 3.4及以上版本,pathlib模块提供了更现代和直观的路径处理方式。
- 处理异常:在创建目录时,可能会遇到权限不足、路径错误等问题,使用异常处理可以提高程序的健壮性。
- 检查目录存在性:在创建目录之前,检查其是否已存在可以避免重复创建和不必要的错误。
- 批量操作:无论是从文件读取路径还是从数据库获取路径,批量操作都能提高效率。
通过以上方法和实践,您可以在Python中高效地批量创建目录,满足各种项目需求。无论是数据科学项目中的数据存储,还是大型应用中的文件管理,这些方法都能提供强有力的支持。
相关问答FAQs:
在Python中,如何一次性创建多个目录?
可以使用os
模块的makedirs()
函数来批量创建目录。通过传递一个路径列表,您可以在程序中一次性创建多个目录。例如,可以遍历一个目录列表并为每个目录调用makedirs()
,确保所有指定的目录都能成功创建。
是否可以使用Python脚本从文本文件中读取目录名称并创建相应的目录?
是的,您可以编写一个Python脚本,读取包含目录名称的文本文件。使用open()
函数读取文件后,将每行作为目录名称,通过os.makedirs()
函数逐一创建这些目录。这种方法非常适合批量处理大量目录。
在创建目录时,如何处理已存在的目录以避免错误?
使用os.makedirs()
时,可以设置exist_ok=True
参数。这样,当尝试创建已经存在的目录时,程序不会抛出错误,而是正常运行,确保代码的健壮性。此外,您也可以先使用os.path.exists()
检查目录是否存在,再决定是否创建。
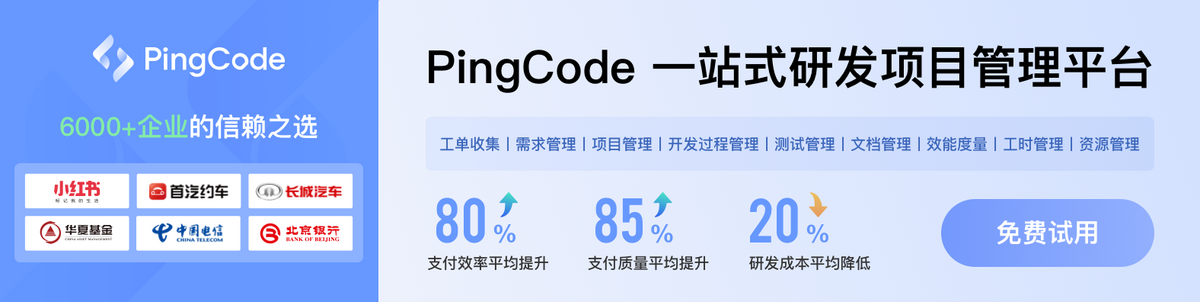