Python轨迹线分解主要通过轨迹分段、数据平滑、特征提取等方法来实现。轨迹分段可以帮助识别不同的运动阶段、数据平滑有助于去除噪声、特征提取则是为了更好地分析和理解轨迹。下面将详细介绍如何在Python中实现这些方法,以分解和分析轨迹线。
一、轨迹分段
轨迹分段是对轨迹数据进行预处理的重要步骤。通常,轨迹数据可以通过位置、时间戳等特征进行分段,以便更好地分析不同运动阶段的特性。
1.1 基于速度变化分段
速度是分析轨迹变化的重要指标之一。通过计算每个点的速度变化,可以识别出轨迹中的关键点,并据此分段。
import numpy as np
def calculate_speed(points):
# points 是一个包含(x, y, time)的列表
speeds = []
for i in range(1, len(points)):
dx = points[i][0] - points[i-1][0]
dy = points[i][1] - points[i-1][1]
dt = points[i][2] - points[i-1][2]
speed = np.sqrt(dx<strong>2 + dy</strong>2) / dt
speeds.append(speed)
return speeds
def segment_by_speed(points, threshold):
speeds = calculate_speed(points)
segments = []
current_segment = [points[0]]
for i, speed in enumerate(speeds):
if speed > threshold:
segments.append(current_segment)
current_segment = [points[i+1]]
else:
current_segment.append(points[i+1])
segments.append(current_segment)
return segments
1.2 基于方向变化分段
轨迹的方向变化也是判断分段的一个重要指标。通过计算每个点的方向变化角度,可以识别出轨迹的转折点。
def calculate_direction(points):
directions = []
for i in range(1, len(points)):
dx = points[i][0] - points[i-1][0]
dy = points[i][1] - points[i-1][1]
direction = np.arctan2(dy, dx)
directions.append(direction)
return directions
def segment_by_direction(points, angle_threshold):
directions = calculate_direction(points)
segments = []
current_segment = [points[0]]
for i, direction in enumerate(directions):
if i > 0:
angle_change = abs(directions[i] - directions[i-1])
if angle_change > angle_threshold:
segments.append(current_segment)
current_segment = [points[i+1]]
else:
current_segment.append(points[i+1])
segments.append(current_segment)
return segments
二、数据平滑
在实际应用中,轨迹数据往往受到噪声的影响,因此需要对数据进行平滑处理,以提高分析的准确性。
2.1 移动平均法
移动平均法是最简单也是最常用的数据平滑方法之一。它通过计算相邻点的平均值来平滑数据。
def moving_average(points, window_size):
smoothed_points = []
for i in range(window_size, len(points)):
window = points[i-window_size:i]
avg_x = np.mean([p[0] for p in window])
avg_y = np.mean([p[1] for p in window])
avg_time = np.mean([p[2] for p in window])
smoothed_points.append((avg_x, avg_y, avg_time))
return smoothed_points
2.2 高斯滤波
高斯滤波是一种更为复杂的平滑方法,通过卷积操作对数据进行平滑处理,能够更有效地去除噪声。
from scipy.ndimage import gaussian_filter1d
def gaussian_smoothing(points, sigma):
x_coords = [p[0] for p in points]
y_coords = [p[1] for p in points]
times = [p[2] for p in points]
smoothed_x = gaussian_filter1d(x_coords, sigma)
smoothed_y = gaussian_filter1d(y_coords, sigma)
smoothed_points = list(zip(smoothed_x, smoothed_y, times))
return smoothed_points
三、特征提取
通过提取轨迹中的重要特征,可以更好地理解和分析轨迹的运动模式。
3.1 提取速度和加速度
速度和加速度是轨迹分析中的基本特征,通过计算这些特征可以更好地描述轨迹的运动状态。
def extract_speed_and_acceleration(points):
speeds = calculate_speed(points)
accelerations = []
for i in range(1, len(speeds)):
acceleration = (speeds[i] - speeds[i-1]) / (points[i][2] - points[i-1][2])
accelerations.append(acceleration)
return speeds, accelerations
3.2 提取曲率
曲率是描述轨迹形状的重要几何特征。通过计算曲率,可以识别轨迹中的弯曲程度。
def calculate_curvature(points):
curvatures = []
for i in range(1, len(points) - 1):
p1, p2, p3 = points[i-1], points[i], points[i+1]
a = np.linalg.norm(np.array(p2) - np.array(p1))
b = np.linalg.norm(np.array(p3) - np.array(p2))
c = np.linalg.norm(np.array(p3) - np.array(p1))
if a * b * c == 0:
curvature = 0
else:
curvature = 4 * (a*b*c) / ((a+b+c) * (b+c-a) * (c+a-b) * (a+b-c))
curvatures.append(curvature)
return curvatures
四、应用场景和注意事项
轨迹线分解在许多领域都有重要应用,如交通监控、运动分析、机器人路径规划等。在实际应用中,需要根据具体的应用场景选择合适的分解和分析方法。
4.1 应用场景
- 交通监控:通过分解车辆轨迹,可以识别异常行为,如急刹车、急转弯等。
- 运动分析:在运动科学中,可以通过分解运动轨迹来分析运动员的技术动作。
- 路径规划:在机器人领域,轨迹分解有助于规划合理的运动路径,避免障碍物。
4.2 注意事项
- 数据质量:轨迹分解的效果很大程度上取决于数据的质量。在进行数据分析之前,应确保数据的准确性和完整性。
- 参数选择:分段和平滑方法中涉及的参数(如速度阈值、角度阈值、窗口大小等)需要根据具体情况进行调整。
- 计算性能:对于大规模轨迹数据,计算性能可能成为一个瓶颈。在这种情况下,可以考虑使用更高效的算法或并行计算技术。
通过以上方法,可以在Python中有效地分解和分析轨迹线,为进一步的研究和应用提供有力支持。根据具体需求和数据特点,灵活选择和调整分解策略,将有助于获得更有价值的分析结果。
相关问答FAQs:
如何在Python中实现轨迹线的分解?
在Python中,轨迹线的分解通常涉及到将连续的坐标点转换成更简单的线段或曲线。可以使用数学库如NumPy来处理坐标数据,并利用插值或拟合技术来简化轨迹。可以考虑使用scipy库中的插值函数,或者利用matplotlib进行可视化,帮助分析和分解轨迹。
有哪些库可以帮助我分解Python中的轨迹线?
在Python中,常用的库有NumPy、SciPy和Matplotlib。NumPy提供了强大的数组处理功能,SciPy则包含了多种插值和曲线拟合的方法,Matplotlib可以帮助可视化轨迹线的分解效果。此外,Pandas也可以用于处理和分析轨迹数据,方便用户进行数据清洗和操作。
如何判断轨迹线的分解效果是否理想?
评估轨迹线分解效果的标准可以包括:分解后的线段数量、拟合曲线的平滑度以及与原始轨迹的相似度。可以通过计算分解后的线段与原始线段之间的距离,或使用均方根误差(RMSE)等指标进行量化。此外,借助可视化工具展示原始轨迹与分解后的轨迹对比,也是一个有效的评估手段。
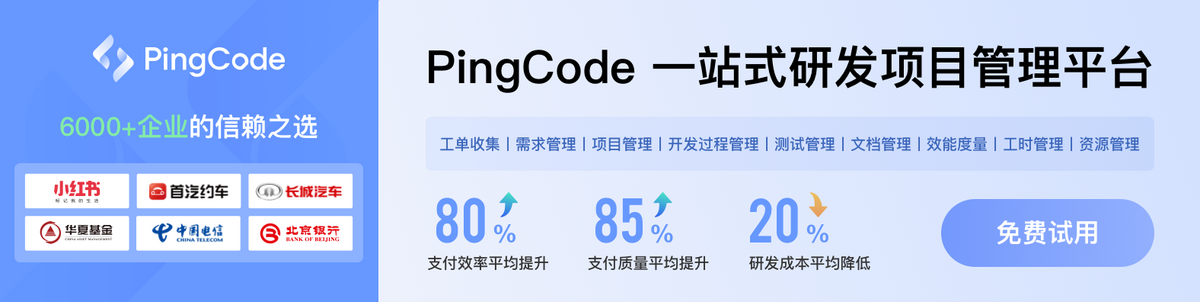