在Python中测试运算时间可以通过多种方法实现,包括使用time模块、timeit模块、以及cProfile模块。每种方法都有其独特的优点,适用于不同的场景。 其中,使用time模块是最简单和直接的方法,而timeit模块则提供了更精确的计时工具,并且可以自动处理一些常见的计时问题。cProfile模块则适用于分析整个程序的性能。接下来,将详细介绍每种方法的使用细节。
一、使用TIME模块
使用time模块是测试代码执行时间的最简单方法。它主要通过记录代码块执行前后的时间差来计算执行时间。
-
基本用法
使用time模块的time()函数可以获取当前时间戳,结合start和end时间戳的差值就能得到代码块的执行时间。
import time
start_time = time.time()
需要测试的代码块
end_time = time.time()
execution_time = end_time - start_time
print(f"Execution time: {execution_time} seconds")
-
示例
例如,计算一个列表中所有元素的和:
import time
def sum_of_list(lst):
total = 0
for number in lst:
total += number
return total
numbers = list(range(1000000))
start_time = time.time()
result = sum_of_list(numbers)
end_time = time.time()
print(f"Sum: {result}")
print(f"Execution time: {end_time - start_time} seconds")
详细描述: 通过time模块的基本用法,可以快速测量一个函数的执行时间。然而,这种方法在处理复杂的函数或需要多次测试时可能不够精确,因为它只测量一次执行时间,没有考虑到外部环境的影响。
二、使用TIMEIT模块
timeit模块是Python提供的一个更为精确的测量小块代码执行时间的工具。它避免了一些常见的计时陷阱,比如系统时钟的分辨率和垃圾回收的影响。
-
基本用法
timeit模块提供了一个简单的timeit()函数来测量代码片段的执行时间。默认情况下,它会执行100万次以获得更准确的结果。
import timeit
execution_time = timeit.timeit('"-".join(str(n) for n in range(100))', number=10000)
print(f"Execution time: {execution_time} seconds")
-
使用装饰器
可以使用timeit模块的装饰器来测量函数的执行时间。
import timeit
@timeit.timeit
def test_function():
return "-".join(str(n) for n in range(100))
print(f"Execution time: {test_function()} seconds")
-
命令行使用
timeit模块也可以直接在命令行中使用,用于测试脚本或模块的执行时间。
python -m timeit '"-".join(str(n) for n in range(100))'
详细描述: 使用timeit模块可以测量代码执行时间的平均值,减少单次测量可能带来的误差。这对于需要多次运行以获得更准确结果的场景尤其有效。
三、使用CPROFILE模块
cProfile模块适用于对整个程序进行性能分析。它能够提供函数调用次数、每次调用时间以及总时间等详细信息。
-
基本用法
使用cProfile模块可以很方便地分析整个程序的性能,帮助定位性能瓶颈。
import cProfile
def test_function():
return "-".join(str(n) for n in range(100))
cProfile.run('test_function()')
-
分析输出
cProfile的输出包含了函数名、调用次数、递归次数、每次调用的时间以及累计时间等信息。通过这些信息,可以很容易地找到程序中的性能瓶颈。
-
结合pstats模块
可以结合pstats模块对cProfile的输出进行更详细的分析和排序。
import cProfile
import pstats
def test_function():
return "-".join(str(n) for n in range(100))
profile = cProfile.Profile()
profile.enable()
test_function()
profile.disable()
stats = pstats.Stats(profile)
stats.sort_stats('time').print_stats(10)
详细描述: cProfile模块不仅可以测量函数的执行时间,还可以提供调用次数和调用链的信息。这对于需要进行深入性能分析的场景非常有用。
四、使用其他第三方工具
除了标准库提供的工具外,还有许多第三方工具可以用于测量Python代码的执行时间。
-
line_profiler
line_profiler是一个以行为单位进行性能分析的工具,适用于需要精确到代码行级别的性能分析。
pip install line_profiler
使用示例:
@profile
def test_function():
total = 0
for i in range(100000):
total += i
return total
执行:
kernprof -l -v script.py
-
pyinstrument
pyinstrument是一个简单但功能强大的性能分析工具,适用于快速了解代码的性能瓶颈。
pip install pyinstrument
使用示例:
from pyinstrument import Profiler
profiler = Profiler()
profiler.start()
def test_function():
total = 0
for i in range(100000):
total += i
return total
test_function()
profiler.stop()
print(profiler.output_text(unicode=True, color=True))
详细描述: 这些第三方工具可以提供更为详细和多样化的性能分析报告,适用于需要深入分析程序性能的场景。
五、选择合适的工具
不同的工具适用于不同的场景,选择合适的工具可以更高效地完成性能测试。
-
简单的代码片段
对于简单的代码片段,使用time或timeit模块即可。
-
整个程序的性能分析
对于需要分析整个程序性能的场景,cProfile模块是一个很好的选择。
-
深入分析
对于需要深入分析某些函数或代码块的性能瓶颈,line_profiler和pyinstrument是不错的选择。
总结: 在Python中测试运算时间有多种方法可供选择,包括标准库提供的time、timeit和cProfile模块,以及第三方工具line_profiler和pyinstrument。选择合适的工具可以帮助我们更有效地进行性能分析和优化。每种方法都有其独特的优势,适用于不同的应用场景。
相关问答FAQs:
如何在Python中测量一段代码的执行时间?
在Python中,可以使用time
模块中的time()
函数来测量代码的执行时间。通过在代码开始和结束时记录时间戳,可以计算出代码的总运行时间。例如:
import time
start_time = time.time()
# 需要测试的代码
end_time = time.time()
print(f"代码执行时间:{end_time - start_time}秒")
这种方法简单易用,适合快速测试小段代码的性能。
使用哪些工具可以更方便地测试Python代码的性能?
除了time
模块,timeit
模块提供了更精准的性能测试,尤其适合小段代码。它通过多次运行代码来减少偶然因素的影响。使用示例:
import timeit
execution_time = timeit.timeit('your_code_here', number=1000)
print(f"平均执行时间:{execution_time / 1000}秒")
这种方法能够提供更可靠的性能评估。
在Python中如何分析代码性能瓶颈?
要深入分析代码性能,可以使用cProfile
模块。该模块能提供详细的性能报告,包括每个函数的调用次数和耗时。使用方式如下:
import cProfile
def your_function():
# 需要分析的代码
cProfile.run('your_function()')
通过分析输出结果,您可以识别出性能瓶颈,从而进行优化。
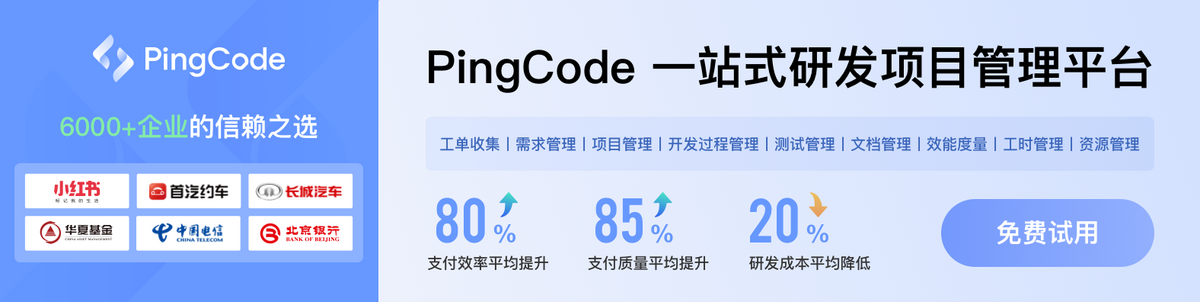