在Python中对文件进行排序,可以通过读取文件内容、使用排序算法、将排序结果写入文件等步骤实现。常用的方法包括读取文件内容、使用Python内置的排序函数、排序后的数据写回文件。接下来,我们将详细介绍如何进行这些操作。
一、读取文件内容
在对文件进行排序之前,首先需要读取文件内容。在Python中,可以使用内置的open()
函数来打开文件,并使用readlines()
方法将文件的每一行读取为列表元素。这样可以方便后续的排序操作。
with open('example.txt', 'r') as file:
lines = file.readlines()
二、排序文件内容
一旦获取了文件内容,可以使用Python的内置排序函数sorted()
来对内容进行排序。sorted()
函数支持多种排序方式,包括按字母顺序、数字大小等进行排序。默认情况下,sorted()
函数按字母顺序对字符串进行排序。
sorted_lines = sorted(lines)
自定义排序:如果需要对特定格式的数据(如数字、日期)进行排序,可以通过sorted()
函数的key
参数传入自定义的排序函数。例如,假设文件内容是由数字组成的字符串,可以通过以下方式实现数字排序:
sorted_lines = sorted(lines, key=lambda x: int(x.strip()))
三、将排序结果写入文件
排序完成后,将结果写回文件中。可以创建一个新的文件来保存排序结果,或者覆盖原文件。使用open()
函数以写模式打开文件,并通过writelines()
方法将排序后的内容写入文件。
with open('sorted_example.txt', 'w') as file:
file.writelines(sorted_lines)
四、完整示例及优化技巧
以下是一个完整的示例代码,演示如何从文件读取内容,对其排序,并将结果写入新文件中。同时,一些优化技巧如处理大文件、使用生成器等也将在此部分介绍。
def sort_file(input_file, output_file):
with open(input_file, 'r') as file:
lines = file.readlines()
# 对文件内容进行排序
sorted_lines = sorted(lines, key=lambda x: int(x.strip()))
# 将排序结果写入新文件
with open(output_file, 'w') as file:
file.writelines(sorted_lines)
sort_file('example.txt', 'sorted_example.txt')
处理大文件:当处理大文件时,直接将内容读取到内存中可能导致内存不足。此时可以使用生成器或者逐行读取的方法来优化。以下是使用生成器的示例:
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
def sort_large_file(input_file, output_file):
lines = list(read_large_file(input_file))
sorted_lines = sorted(lines, key=lambda x: int(x.strip()))
with open(output_file, 'w') as file:
file.writelines(sorted_lines)
sort_large_file('large_example.txt', 'sorted_large_example.txt')
五、使用Pandas进行文件排序
对于结构化数据文件(如CSV),使用Pandas库进行排序会更加方便。Pandas提供了强大的数据处理和分析功能,能够轻松读取、排序和保存数据。
import pandas as pd
def sort_csv(input_file, output_file, column_name):
# 读取CSV文件
df = pd.read_csv(input_file)
# 对指定列进行排序
sorted_df = df.sort_values(by=column_name)
# 将排序结果保存到新文件
sorted_df.to_csv(output_file, index=False)
sort_csv('data.csv', 'sorted_data.csv', 'age')
总结:在Python中对文件进行排序主要涉及文件的读取、数据排序和结果写入三个步骤。使用内置的sorted()
函数可以实现基本的排序需求,而对于更复杂的情况,如处理大文件或特定格式的数据,可能需要使用生成器、Pandas库等工具进行优化。通过上述方法,您可以根据具体需求灵活选择合适的解决方案。
相关问答FAQs:
如何使用Python对文本文件中的数据进行排序?
可以通过读取文件内容,将其存储在列表中,然后使用Python内置的sorted()
函数或列表的sort()
方法进行排序。排序完成后,可以将结果写入新的文件中。示例代码如下:
with open('data.txt', 'r') as file:
lines = file.readlines()
sorted_lines = sorted(lines)
with open('sorted_data.txt', 'w') as sorted_file:
sorted_file.writelines(sorted_lines)
Python支持哪些排序算法,哪种适合文件排序?
Python的sorted()
函数和列表的sort()
方法都使用Timsort算法,这是一种高效的排序算法,适合大多数数据集。Timsort在处理有序数据时表现优越,适合对文件内容进行排序,尤其是在数据量较大时。
如果文件内容是以特定格式存储的(如CSV),该如何排序?
对于以CSV格式存储的文件,可以使用csv
模块来读取和排序。可以将文件内容转换为字典或列表的形式,然后依据某一列进行排序。示例代码如下:
import csv
with open('data.csv', 'r') as csvfile:
reader = csv.DictReader(csvfile)
sorted_data = sorted(reader, key=lambda row: row['column_name']) # 根据特定列排序
with open('sorted_data.csv', 'w', newline='') as csvfile:
fieldnames = sorted_data[0].keys()
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
writer.writerows(sorted_data)
通过这种方式,可以更灵活地处理复杂的数据格式。
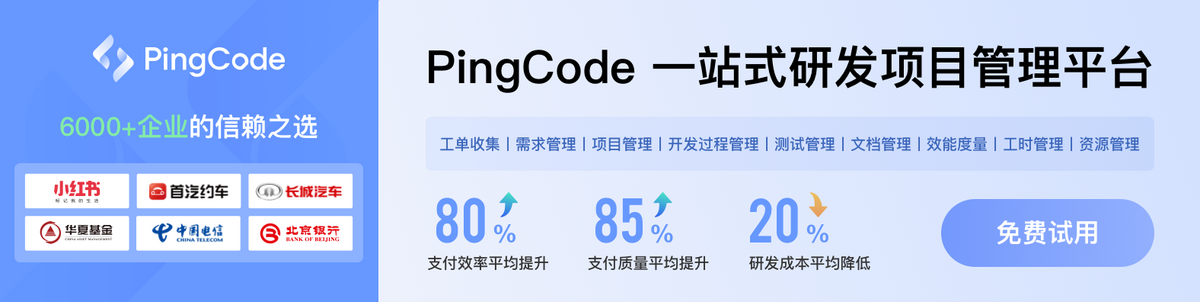