使用Python绘制时钟可以通过多种方式实现,常见的方法包括使用Tkinter库构建GUI界面、利用matplotlib绘图库绘制模拟时钟、或使用Pygame进行游戏化展示。每种方法都有其独特的实现步骤和优缺点,其中Tkinter更适合初学者学习GUI编程,matplotlib提供了强大的绘图功能,而Pygame则适合需要动态显示的应用。下面将详细介绍如何使用这些方法绘制时钟。
一、使用TKINTER绘制数字时钟
Tkinter是Python的标准GUI库,提供了简单而强大的界面构建能力。通过Tkinter可以轻松地创建一个数字时钟。
-
基本设置和窗口布局
在开始绘制时钟之前,需要初始化Tkinter窗口并设置其基本属性。首先,导入Tkinter库并创建主窗口。可以通过设置窗口标题、大小以及背景颜色来美化界面。
import tkinter as tk
root = tk.Tk()
root.title("Digital Clock")
root.geometry("400x200")
root.configure(bg='black')
-
显示当前时间
使用Python的
time
模块,可以获取当前系统时间,并通过Tkinter的Label组件显示在窗口上。为了使时钟实时更新,需要使用Tkinter的after方法来定期更新显示的时间。from time import strftime
def time():
string = strftime('%H:%M:%S %p')
label.config(text=string)
label.after(1000, time)
label = tk.Label(root, font=('calibri', 40, 'bold'), background='black', foreground='white')
label.pack(anchor='center')
time()
-
运行主循环
最后,使用Tkinter的主循环方法
mainloop()
来保持窗口运行。root.mainloop()
通过上述步骤,可以创建一个简单的数字时钟。Tkinter的优势在于其简单易用,适合快速开发小型应用。
二、使用MATPLOTLIB绘制模拟时钟
matplotlib是Python中最流行的绘图库之一,可以用于绘制各种复杂的图形,包括模拟时钟。
-
初始化绘图环境
首先,导入必要的库,包括
matplotlib.pyplot
和datetime
模块。接下来,设置绘图的基本参数,如图形大小和背景色。import matplotlib.pyplot as plt
from datetime import datetime
import numpy as np
fig, ax = plt.subplots()
ax.set_facecolor('black')
ax.set_aspect('equal')
-
绘制时钟表盘
使用
matplotlib
的极坐标系,可以很方便地绘制圆形表盘。通过在0到2π的范围内绘制圆周,可以形成表盘的外观。此外,还可以添加刻度和数字标记。clock_face = plt.Circle((0, 0), 1, color='white', fill=False, linewidth=3)
ax.add_artist(clock_face)
for i in range(12):
angle = np.pi / 6 * i
x = np.cos(angle)
y = np.sin(angle)
ax.text(0.9*x, 0.9*y, str(i+1), horizontalalignment='center', verticalalignment='center', color='white')
-
绘制时针、分针和秒针
获取当前时间并计算每个指针的旋转角度。使用
plot
函数绘制指针,并通过set_data
方法实时更新其位置。def update_clock():
now = datetime.now()
second_angle = np.pi/2 - np.pi/30 * now.second
minute_angle = np.pi/2 - np.pi/30 * now.minute
hour_angle = np.pi/2 - np.pi/6 * (now.hour % 12)
second_hand.set_data([0, np.cos(second_angle)], [0, np.sin(second_angle)])
minute_hand.set_data([0, 0.8*np.cos(minute_angle)], [0, 0.8*np.sin(minute_angle)])
hour_hand.set_data([0, 0.5*np.cos(hour_angle)], [0, 0.5*np.sin(hour_angle)])
plt.draw()
second_hand, = ax.plot([0, 1], [0, 0], color='red', linewidth=1)
minute_hand, = ax.plot([0, 1], [0, 0], color='blue', linewidth=2)
hour_hand, = ax.plot([0, 1], [0, 0], color='green', linewidth=3)
-
设置动画效果
使用
matplotlib.animation
模块可以实现动态更新效果。通过定时调用update_clock
函数,可以实现秒针、分针和时针的连续运动。import matplotlib.animation as animation
ani = animation.FuncAnimation(fig, update_clock, interval=1000)
plt.show()
通过这些步骤,可以使用matplotlib绘制一个精美的模拟时钟。该方法的优点在于灵活性高,能够自定义各种细节。
三、使用PYGAME绘制动态时钟
Pygame是一个用于游戏开发的Python库,虽然主要用于创建游戏,但也可以用于绘制动态时钟。
-
初始化Pygame环境
首先,导入Pygame库并初始化。接下来,设置窗口大小和背景颜色。
import pygame
import time
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption('Analog Clock')
-
加载和显示时钟背景
可以使用Pygame绘制一个简单的时钟背景或加载一张时钟图片作为背景。
background = pygame.Surface(screen.get_size())
background = background.convert()
background.fill((255, 255, 255))
-
绘制指针
使用Pygame的
draw.line
方法绘制指针。通过获取当前时间并计算每个指针的旋转角度,实时更新指针的位置。def draw_hand(angle, length, width, color):
x = 200 + length * np.cos(angle)
y = 200 - length * np.sin(angle)
pygame.draw.line(screen, color, (200, 200), (x, y), width)
def update_clock():
current_time = time.localtime()
second_angle = np.pi/2 - np.pi/30 * current_time.tm_sec
minute_angle = np.pi/2 - np.pi/30 * current_time.tm_min
hour_angle = np.pi/2 - np.pi/6 * (current_time.tm_hour % 12)
draw_hand(second_angle, 180, 1, (255, 0, 0))
draw_hand(minute_angle, 150, 3, (0, 0, 255))
draw_hand(hour_angle, 100, 6, (0, 255, 0))
-
主循环
使用Pygame的主循环保持程序运行,并不断刷新屏幕以显示动态时钟。
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.blit(background, (0, 0))
update_clock()
pygame.display.flip()
pygame.time.delay(1000)
pygame.quit()
Pygame的优点在于其动态更新和丰富的图形处理能力,非常适合需要复杂动画效果的应用。
总结,使用Python绘制时钟提供了丰富多样的选择,不同的方法适用于不同的应用场景。通过以上介绍,希望能帮助读者选择适合自己项目的方法,并成功实现时钟的绘制。
相关问答FAQs:
如何在Python中绘制一个简单的时钟?
要绘制一个简单的时钟,您可以使用Python的matplotlib
库。首先,确保您安装了matplotlib
。然后,您可以利用极坐标系绘制时钟的表盘和指针。以下是一个简单的代码示例:
import numpy as np
import matplotlib.pyplot as plt
from datetime import datetime
def draw_clock():
plt.figure(figsize=(6, 6))
plt.subplot(111, polar=True)
plt.gca().set_theta_zero_location('N')
plt.gca().set_theta_direction(-1)
# 画表盘
for i in range(12):
angle = np.deg2rad(i * 30)
plt.text(angle, 1.1, str(i + 1), horizontalalignment='center', verticalalignment='center', fontsize=15)
# 获取当前时间
now = datetime.now()
hours = now.hour % 12 + now.minute / 60
minutes = now.minute + now.second / 60
seconds = now.second
# 画指针
hour_angle = np.deg2rad(hours * 30)
minute_angle = np.deg2rad(minutes * 6)
second_angle = np.deg2rad(seconds * 6)
plt.plot([0, hour_angle], [0, 0.5], color='black', linewidth=5)
plt.plot([0, minute_angle], [0, 0.8], color='blue', linewidth=3)
plt.plot([0, second_angle], [0, 0.9], color='red', linewidth=1)
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.axis('off')
plt.show()
draw_clock()
绘制时钟时,如何处理时区问题?
在绘制时钟时,如果您需要显示特定时区的时间,可以使用pytz
库来处理时区问题。通过该库,您可以将当前时间转换为目标时区的时间。例如:
import pytz
timezone = pytz.timezone('Asia/Shanghai')
now = datetime.now(timezone)
这样,您就可以根据需要获取并绘制不同时区的时间。
使用Python绘制时钟是否需要图形界面库?
不一定。虽然使用图形界面库(如tkinter
或pygame
)可以创建更复杂和互动的时钟应用,但如果您的目的是简单地绘制时钟图形,matplotlib
已经足够。它提供了极好的绘图功能,可以方便地生成静态图像。如果您需要实时更新的时钟,可能需要考虑使用图形界面库。
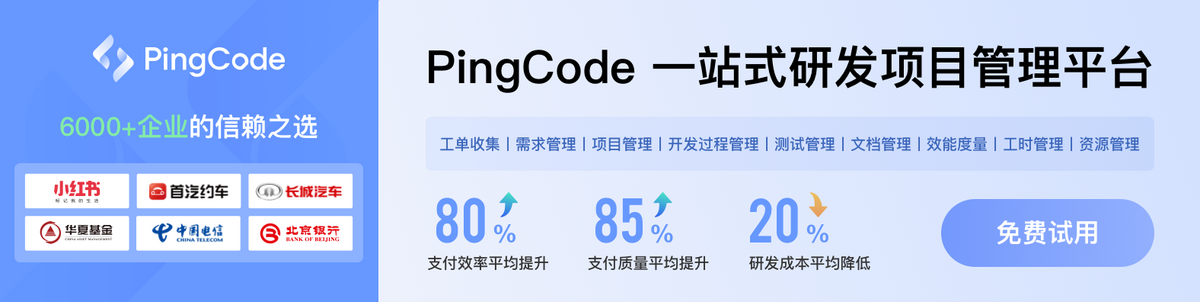