在Python爬虫中实现多次请求的关键在于:使用循环结构、处理会话管理、错误处理与重试机制、优化请求效率。 其中,循环结构是实现多次请求的基础,通过循环可以轻松遍历多个URL或数据集;错误处理与重试机制则确保爬虫在遇到临时网络问题或目标网站异常时能够自动重试,而不是立即停止;使用会话管理则可以在多次请求中保持登录状态或其他会话信息。接下来,我们详细探讨如何实现这些关键要素。
一、循环结构
循环结构是实现多次请求的基础。在Python中,可以使用for
循环或while
循环来遍历多个URL或数据集。
1. 使用for
循环
当我们需要对一个已知列表中的每个URL进行请求时,for
循环是最简便的方法。例如,当我们有一个URL列表时,可以这样实现:
import requests
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
for url in urls:
response = requests.get(url)
if response.status_code == 200:
print(f"Successfully fetched {url}")
else:
print(f"Failed to fetch {url}")
在上述代码中,for
循环遍历urls
列表中的每个元素,并对其进行HTTP请求。请求结果通过状态码判断并输出。
2. 使用while
循环
当我们需要根据某些条件进行多次请求时,while
循环是一个很好的选择。例如,可以用它来实现对某个页面的多次尝试,直到成功为止:
import requests
url = 'http://example.com/page'
max_attempts = 5
attempt = 0
while attempt < max_attempts:
attempt += 1
response = requests.get(url)
if response.status_code == 200:
print("Successfully fetched the page")
break
else:
print(f"Attempt {attempt} failed, retrying...")
在这个例子中,while
循环会在请求失败时自动重试,最多尝试max_attempts
次。
二、会话管理
在爬虫过程中,有时需要在多次请求中保持会话信息,例如保持登录状态。这时,可以使用requests
库中的Session
对象。
1. 使用Session
对象
Session
对象可以在多次请求中保持某些参数,例如cookies、headers等。这对于需要登录的站点尤为重要。例如:
import requests
session = requests.Session()
login_url = 'http://example.com/login'
data = {'username': 'user', 'password': 'pass'}
session.post(login_url, data=data)
protected_url = 'http://example.com/protected'
response = session.get(protected_url)
if response.status_code == 200:
print("Accessed protected content")
在这个例子中,使用Session
对象可以在登录后直接访问受保护的页面,而无需在每次请求时重新登录。
三、错误处理与重试机制
在网络请求过程中,可能会遇到各种错误,例如超时、连接错误等。为了使爬虫更加健壮,可以加入错误处理和重试机制。
1. 错误处理
可以使用try-except
语句来捕获异常,并根据需要进行处理。例如:
import requests
url = 'http://example.com/page'
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"Other error occurred: {err}")
else:
print("Successfully fetched the page")
在这个例子中,raise_for_status()
方法会在HTTP请求返回错误码时抛出异常,try-except
语句则捕获并处理这些异常。
2. 重试机制
可以在捕获到异常时,自动进行重试。例如,结合while
循环:
import requests
import time
url = 'http://example.com/page'
max_attempts = 5
attempt = 0
delay = 5 # seconds
while attempt < max_attempts:
attempt += 1
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Attempt {attempt} failed: {e}, retrying in {delay} seconds...")
time.sleep(delay)
else:
print("Successfully fetched the page")
break
在这里,time.sleep()
用于在每次重试前等待一段时间,避免对服务器造成过大压力。
四、优化请求效率
为了提高爬虫的效率,可以采用多线程或异步请求等技术。
1. 多线程
多线程可以让爬虫同时进行多个请求,从而提高效率。可以使用concurrent.futures
库中的ThreadPoolExecutor
来实现:
import requests
from concurrent.futures import ThreadPoolExecutor
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
def fetch(url):
response = requests.get(url)
return response.status_code
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(fetch, urls)
for result in results:
print(f"Fetched with status code: {result}")
在这个例子中,ThreadPoolExecutor
会创建多个线程来同时处理urls
列表中的请求。
2. 异步请求
异步请求也是一种提高效率的方法,可以使用aiohttp
库来实现:
import aiohttp
import asyncio
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
async def fetch(session, url):
async with session.get(url) as response:
return response.status
async def main():
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(f"Fetched with status code: {result}")
asyncio.run(main())
在这里,aiohttp
库的异步特性允许程序在等待网络响应时执行其他操作,从而提高效率。
通过这些技术,Python爬虫可以在多次请求中实现高效、稳定的运行,适用于各种复杂的网络环境和需求。
相关问答FAQs:
如何提高Python爬虫的抓取效率?
在进行多次抓取时,效率是一个重要的考量因素。可以通过使用异步请求库(如aiohttp)或多线程(如concurrent.futures模块)来提高抓取效率。此外,合理设置请求间隔时间,以避免被目标网站封禁也是很重要的。
在Python爬虫中如何处理反爬虫机制?
许多网站会使用反爬虫技术来保护其内容。为了绕过这些机制,可以考虑使用代理服务器、随机化请求头、模拟浏览器行为(通过selenium等库)以及进行数据抓取时的IP轮换等策略。
如何管理Python爬虫的请求频率?
适当的请求频率管理可以有效减少被封禁的风险。可以使用time.sleep()方法在请求之间设置延迟,或者利用第三方库如scrapy中的AutoThrottle功能,自动调整请求频率,确保在不影响网站正常运行的情况下进行抓取。
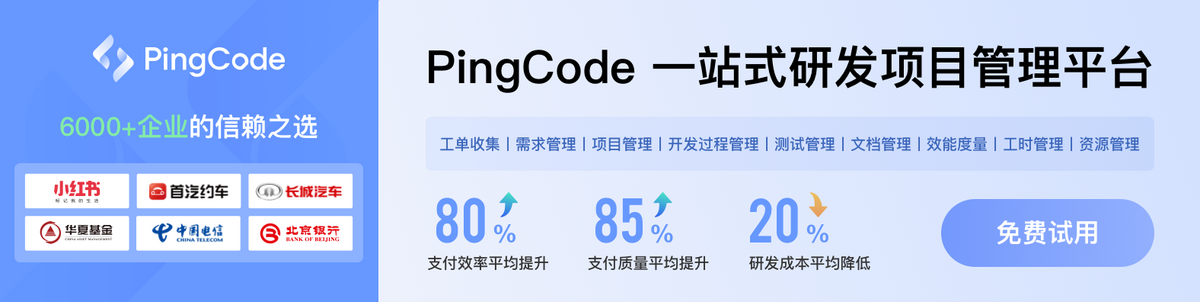