一、概述
使用Python获取图像可以通过Web抓取、摄像头捕获、文件读取等多种方式实现。Web抓取是从互联网上下载图像,通常使用库如requests
和BeautifulSoup
;摄像头捕获则是通过设备的摄像头实时获取图像,可以使用OpenCV
库;文件读取是从本地存储中加载图像,常用的库有PIL
(Pillow)和OpenCV
。接下来,我们将详细介绍这几种方法。
二、WEB抓取图像
- 使用Requests库
requests
库是一个简单而强大的HTTP库,可以轻松地从网络上获取图像。首先,我们需要安装该库,可以通过命令pip install requests
进行安装。
import requests
def download_image(url, save_path):
response = requests.get(url)
if response.status_code == 200:
with open(save_path, 'wb') as file:
file.write(response.content)
else:
print("Failed to retrieve image")
使用示例
download_image('https://example.com/image.jpg', 'local_image.jpg')
上面的代码通过requests.get()
方法请求图像的URL,并将返回的内容写入本地文件中。
- 使用BeautifulSoup和Requests
在某些情况下,图像可能嵌入在HTML页面中,这时我们可以使用BeautifulSoup
解析HTML文档,找到图像的URL,再结合requests
下载。
from bs4 import BeautifulSoup
import requests
def fetch_images_from_page(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
images = soup.find_all('img')
image_urls = [img['src'] for img in images]
return image_urls
使用示例
urls = fetch_images_from_page('https://example.com')
for i, url in enumerate(urls):
download_image(url, f'local_image_{i}.jpg')
这里我们利用BeautifulSoup
解析页面并提取所有<img>
标签的src
属性。
三、摄像头捕获图像
- 使用OpenCV库
OpenCV
库是一个强大的计算机视觉库,可以轻松实现摄像头捕获。首先,我们需要安装该库:pip install opencv-python
。
import cv2
def capture_image_from_camera():
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
if ret:
cv2.imwrite('captured_image.jpg', frame)
cap.release()
使用示例
capture_image_from_camera()
此代码通过VideoCapture
对象访问摄像头,并通过read()
方法捕获图像,最后将图像写入文件。
- 显示和捕获实时视频流
使用OpenCV不仅可以捕获单帧图像,还可以显示和处理实时视频流。
def show_video_stream():
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
cv2.imshow('Video Stream', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
使用示例
show_video_stream()
这里使用imshow
显示视频流,通过waitKey
检测按键以便在按下'q'时退出。
四、文件读取图像
- 使用Pillow库
Pillow
(PIL)是Python中用于图像处理的库,支持多种文件格式,使用简单。
from PIL import Image
def open_image(file_path):
image = Image.open(file_path)
image.show()
使用示例
open_image('local_image.jpg')
通过Image.open()
方法加载图像,并调用show()
方法显示。
- 使用OpenCV读取和显示
OpenCV也支持从文件读取图像,并提供丰富的图像处理功能。
def read_and_display_image(file_path):
image = cv2.imread(file_path)
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
使用示例
read_and_display_image('local_image.jpg')
这里使用imread
加载图像并通过imshow
显示。
五、总结
通过Python获取图像的方法多种多样,包括Web抓取、摄像头捕获和文件读取等。对于不同的需求和场景,可以选择合适的库和方法。Web抓取适用于从网络批量下载图像,摄像头捕获适合于实时图像处理,而文件读取则用于本地图像的加载和处理。在实际应用中,这些技术可以组合使用,以实现复杂的图像处理任务。通过不断的学习和实践,可以更好地掌握这些技能,提高图像处理能力。
相关问答FAQs:
如何使用Python从网络获取图像?
可以使用Python的requests
库来从网络获取图像。首先,安装requests
库(如果尚未安装),然后使用requests.get()
方法下载图像,接着将其保存到本地文件系统。例如:
import requests
url = 'https://example.com/image.jpg'
response = requests.get(url)
with open('image.jpg', 'wb') as file:
file.write(response.content)
这样就可以将网络上的图像下载并保存到本地。
Python支持哪些图像处理库?
Python中有多个流行的图像处理库,包括PIL
(Python Imaging Library)、OpenCV
、scikit-image
和imageio
等。PIL
是一个强大的库,适用于图像打开、处理和保存;OpenCV
则更适合计算机视觉任务,支持大量的图像和视频处理功能;scikit-image
提供了一些高级图像处理功能,适合科学计算和图像分析。
如何从本地文件读取图像并显示?
可以使用PIL
库(或其分支Pillow
)来读取本地图像并显示。安装Pillow
后,可以使用以下代码读取并展示图像:
from PIL import Image
import matplotlib.pyplot as plt
image = Image.open('local_image.jpg')
plt.imshow(image)
plt.axis('off') # 不显示坐标轴
plt.show()
这种方法可以轻松地将本地图像展示在屏幕上,适合用于数据可视化和图像分析。
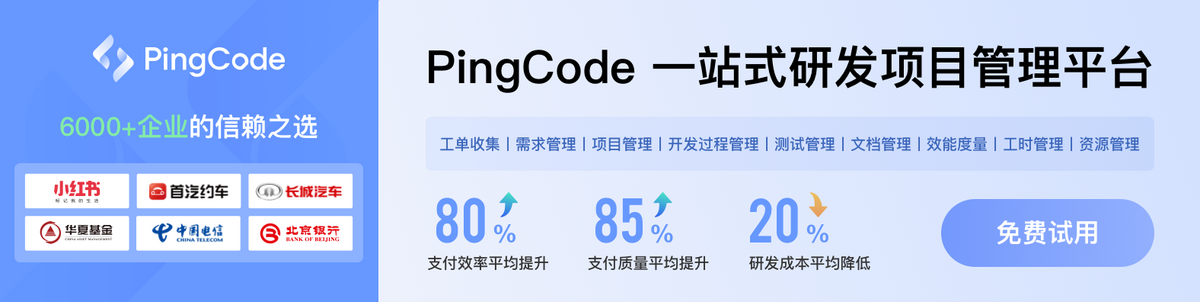