在Python中求代价函数的过程可以通过多种方法实现,常见的方法包括:手动定义代价函数、使用NumPy库进行向量化操作、利用SciPy库的优化功能。以下将详细介绍如何手动定义代价函数,并使用NumPy库进行向量化操作。
在机器学习中,代价函数是用于评估模型预测值与实际值之间差异的一个函数。常见的代价函数包括均方误差(MSE)、交叉熵损失等。在Python中求代价函数的过程通常涉及以下几个步骤:首先,定义模型的预测函数;其次,根据预测值和实际值计算损失;最后,通过损失函数计算代价。本文将通过示例详细介绍如何在Python中实现这些步骤。
一、定义代价函数
在机器学习中,代价函数用于评估模型的预测效果,其作用是衡量预测值与实际值之间的差异。为了计算代价函数,首先需要定义一个预测函数。例如,对于线性回归模型,预测函数可以表示为:
def predict(X, theta):
return X.dot(theta)
在这个函数中,X
表示输入特征矩阵,theta
表示模型参数。在得到预测值后,可以定义均方误差(MSE)作为代价函数:
def compute_cost(X, y, theta):
m = len(y)
predictions = predict(X, theta)
cost = (1 / (2 * m)) * np.sum(np.square(predictions - y))
return cost
在这个函数中,y
表示实际值,predictions
表示预测值,m
是样本数量。通过计算预测值与实际值的平方差并取平均值,可以得到均方误差。
二、使用NumPy进行向量化操作
NumPy库提供了强大的向量化操作功能,可以显著提高计算效率。在定义代价函数时,利用NumPy的广播机制,可以避免使用显式的循环,从而提高代码的执行速度。
在上面的compute_cost
函数中,np.square(predictions - y)
是一个向量化操作,计算了预测值与实际值之间的平方差。通过使用NumPy的np.sum
函数,可以快速计算所有样本的误差之和。
三、使用SciPy库进行优化
SciPy库提供了许多优化函数,可以用于自动求解最优模型参数。在优化过程中,SciPy库会自动调用用户定义的代价函数,并根据梯度下降等优化算法调整模型参数。
以下是一个使用SciPy进行优化的示例:
from scipy.optimize import minimize
def cost_function(theta, X, y):
m = len(y)
predictions = X.dot(theta)
cost = (1 / (2 * m)) * np.sum(np.square(predictions - y))
return cost
result = minimize(cost_function, initial_theta, args=(X, y), method='BFGS', options={'disp': True})
optimal_theta = result.x
在这个示例中,cost_function
是用户定义的代价函数,initial_theta
是初始模型参数,X
和y
分别表示特征矩阵和实际值。通过调用minimize
函数,可以得到最优模型参数optimal_theta
。
四、应用代价函数于线性回归
在实际应用中,代价函数常用于线性回归的模型训练中。线性回归是一种用于预测目标变量的线性模型,其基本思想是通过最小化代价函数来优化模型参数。在实现线性回归时,常用的代价函数是均方误差(MSE)。
以下是一个实现线性回归的完整示例:
import numpy as np
定义预测函数
def predict(X, theta):
return X.dot(theta)
定义代价函数
def compute_cost(X, y, theta):
m = len(y)
predictions = predict(X, theta)
cost = (1 / (2 * m)) * np.sum(np.square(predictions - y))
return cost
梯度下降算法
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
cost_history = []
for _ in range(num_iters):
predictions = predict(X, theta)
theta -= (alpha / m) * X.T.dot(predictions - y)
cost_history.append(compute_cost(X, y, theta))
return theta, cost_history
初始化参数
X = np.array([[1, 1], [1, 2], [1, 3]])
y = np.array([1, 2, 3])
theta = np.zeros(X.shape[1])
设置学习率和迭代次数
alpha = 0.01
num_iters = 1000
调用梯度下降算法
theta, cost_history = gradient_descent(X, y, theta, alpha, num_iters)
输出结果
print("Optimal Theta:", theta)
print("Cost History:", cost_history[-5:])
在这个示例中,首先定义了预测函数predict
和代价函数compute_cost
,然后通过梯度下降算法gradient_descent
来优化模型参数theta
。在每次迭代中,计算当前的代价并更新参数,直到达到预定的迭代次数。
五、扩展到多元线性回归
多元线性回归是线性回归在多个特征维度上的扩展。在多元线性回归中,每个特征都有对应的参数,代价函数的定义与单变量线性回归类似。通过向量化操作,可以很容易地将代价函数扩展到多元线性回归中。
以下是一个多元线性回归的示例:
import numpy as np
from sklearn.preprocessing import StandardScaler
标准化特征
def normalize_features(X):
scaler = StandardScaler()
return scaler.fit_transform(X)
定义预测函数
def predict(X, theta):
return X.dot(theta)
定义代价函数
def compute_cost(X, y, theta):
m = len(y)
predictions = predict(X, theta)
cost = (1 / (2 * m)) * np.sum(np.square(predictions - y))
return cost
梯度下降算法
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
cost_history = []
for _ in range(num_iters):
predictions = predict(X, theta)
theta -= (alpha / m) * X.T.dot(predictions - y)
cost_history.append(compute_cost(X, y, theta))
return theta, cost_history
初始化参数
X = np.array([[2104, 3], [1600, 3], [2400, 3], [1416, 2], [3000, 4]])
y = np.array([399900, 329900, 369000, 232000, 539900])
X = normalize_features(X)
X = np.c_[np.ones(X.shape[0]), X] # 添加截距项
theta = np.zeros(X.shape[1])
设置学习率和迭代次数
alpha = 0.01
num_iters = 1000
调用梯度下降算法
theta, cost_history = gradient_descent(X, y, theta, alpha, num_iters)
输出结果
print("Optimal Theta:", theta)
print("Cost History:", cost_history[-5:])
在这个示例中,首先对特征进行了标准化处理,以确保每个特征在同一尺度上。在标准化之后,通过添加截距项来扩展特征矩阵。接着,定义预测函数和代价函数,并通过梯度下降算法优化模型参数。
六、代价函数在其他模型中的应用
代价函数不仅可以用于线性回归,还可以应用于其他模型中,如逻辑回归、支持向量机、神经网络等。在这些模型中,代价函数的定义和优化方法可能有所不同,但其核心思想是相同的:通过最小化代价函数来提高模型的预测性能。
在逻辑回归中,常用的代价函数是交叉熵损失。在神经网络中,代价函数可以是均方误差、交叉熵损失等。在支持向量机中,代价函数通常是合页损失。
下面是逻辑回归中交叉熵损失的示例:
import numpy as np
定义预测函数
def sigmoid(z):
return 1 / (1 + np.exp(-z))
定义代价函数
def compute_cost(X, y, theta):
m = len(y)
predictions = sigmoid(X.dot(theta))
cost = -(1 / m) * (y.T.dot(np.log(predictions)) + (1 - y).T.dot(np.log(1 - predictions)))
return cost
梯度下降算法
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
cost_history = []
for _ in range(num_iters):
predictions = sigmoid(X.dot(theta))
theta -= (alpha / m) * X.T.dot(predictions - y)
cost_history.append(compute_cost(X, y, theta))
return theta, cost_history
初始化参数
X = np.array([[1, 2], [1, 3], [1, 4]])
y = np.array([0, 1, 0])
theta = np.zeros(X.shape[1])
设置学习率和迭代次数
alpha = 0.01
num_iters = 1000
调用梯度下降算法
theta, cost_history = gradient_descent(X, y, theta, alpha, num_iters)
输出结果
print("Optimal Theta:", theta)
print("Cost History:", cost_history[-5:])
在这个示例中,首先定义了sigmoid
函数用于计算预测值,然后定义了交叉熵损失作为代价函数,通过梯度下降算法优化模型参数。
七、总结
通过本文的介绍,我们详细探讨了在Python中求代价函数的过程,包括手动定义代价函数、使用NumPy进行向量化操作,以及利用SciPy库进行优化。在机器学习中,代价函数是一个重要的概念,它用于评估模型的预测效果,并指导模型参数的优化。无论是线性回归、逻辑回归,还是其他复杂模型,代价函数的求解都具有重要的意义。通过本文的示例,读者可以更好地理解代价函数的定义和应用,并在实际项目中灵活运用这些知识。
相关问答FAQs:
如何在Python中实现代价函数的计算?
代价函数通常用于评估机器学习模型的性能。在Python中,可以使用NumPy库来计算代价函数。首先,定义你的模型输出和实际标签,然后使用公式来计算代价。比如,对于线性回归,代价函数是均方误差(MSE),可以用以下公式实现:
import numpy as np
def compute_cost(y_true, y_pred):
return np.mean((y_true - y_pred) ** 2)
这段代码通过输入真实值和预测值来返回代价。
代价函数的选择对模型训练有何影响?
代价函数的选择对模型训练的效果至关重要。不同的任务可能需要不同的代价函数,例如分类问题常用交叉熵损失,而回归问题则常用均方误差。选择合适的代价函数可以帮助算法更快收敛,提升模型的准确性。
如何可视化代价函数的变化?
可视化代价函数的变化可以帮助你更好地理解模型的训练过程。在Python中,可以使用Matplotlib库来绘制代价函数随迭代次数变化的图形。记录每次迭代的代价值,并在训练完成后绘制图表,能够清晰地看到模型的收敛趋势。例如:
import matplotlib.pyplot as plt
costs = [] # 假设这里存储每次迭代的代价
plt.plot(costs)
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.title('Cost Function Over Iterations')
plt.show()
通过这种方式,你可以直观地分析模型的训练情况。
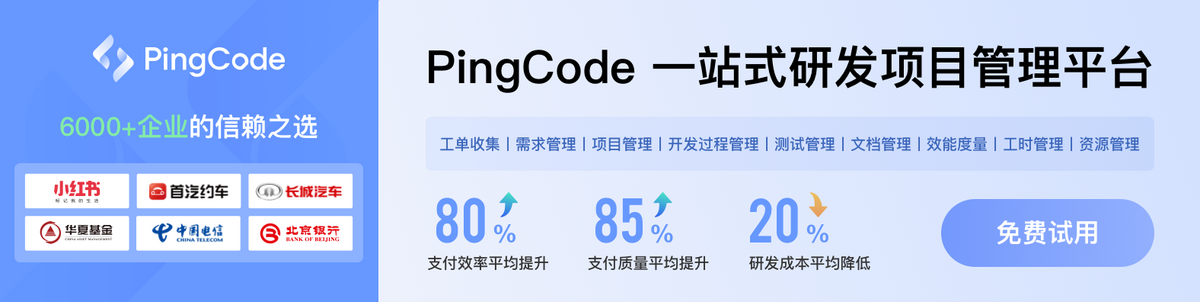