Python获取实时天气可以通过调用天气API、解析天气网站数据、使用Python库实现。 调用天气API是最常用和最简单的方式,其中之一是使用OpenWeatherMap API,通过注册获取API密钥,然后通过HTTP请求获取天气数据。解析天气网站数据需要使用Python的网络爬虫技术,解析网页HTML结构,提取天气信息。这种方法复杂度较高,不同网站的解析方式不同。最后,可以使用一些Python库,如pyowm,它封装了常见天气API的调用,使得获取天气数据更加方便。以下是详细描述如何使用API来获取实时天气。
一、调用天气API
调用天气API是获取实时天气数据的最直接和常用的方法。市面上有许多提供天气数据的API,其中OpenWeatherMap和WeatherStack是最受欢迎的选择。
1、OpenWeatherMap API
OpenWeatherMap提供了多种天气数据服务,包括当前天气、天气预报、历史天气等。用户需要先在OpenWeatherMap网站注册一个账户,并获取一个API密钥。
使用OpenWeatherMap API获取天气数据的步骤:
- 注册和获取API密钥:在OpenWeatherMap官网注册账户并获取API密钥。
- 构建API请求URL:使用Python的requests库构建API请求URL,包含位置参数(如城市名或地理坐标)和API密钥。
- 发送请求并解析响应:使用requests库发送HTTP GET请求,解析返回的JSON格式数据,提取所需天气信息。
示例代码如下:
import requests
def get_weather(city_name, api_key):
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = f"{base_url}q={city_name}&appid={api_key}"
response = requests.get(complete_url)
weather_data = response.json()
if weather_data["cod"] != "404":
main = weather_data["main"]
wind = weather_data["wind"]
weather_description = weather_data["weather"][0]["description"]
print(f"Temperature: {main['temp']}")
print(f"Humidity: {main['humidity']}")
print(f"Weather Description: {weather_description}")
print(f"Wind Speed: {wind['speed']}")
else:
print("City Not Found!")
api_key = "your_api_key" # 替换为你的API密钥
get_weather("London", api_key)
2、WeatherStack API
WeatherStack是另一个流行的天气API,提供了实时天气数据和历史天气数据。它的使用方式与OpenWeatherMap类似。
- 注册和获取API密钥:在WeatherStack官网注册账户并获取API密钥。
- 构建API请求URL:使用requests库构建API请求URL,包含位置参数和API密钥。
- 发送请求并解析响应:发送HTTP请求,解析返回的JSON数据,提取所需天气信息。
示例代码如下:
import requests
def get_weather(city_name, api_key):
base_url = "http://api.weatherstack.com/current?"
complete_url = f"{base_url}access_key={api_key}&query={city_name}"
response = requests.get(complete_url)
weather_data = response.json()
if "current" in weather_data:
current = weather_data["current"]
print(f"Temperature: {current['temperature']}")
print(f"Weather Description: {current['weather_descriptions'][0]}")
print(f"Wind Speed: {current['wind_speed']}")
print(f"Humidity: {current['humidity']}")
else:
print("City Not Found or API Error!")
api_key = "your_api_key" # 替换为你的API密钥
get_weather("London", api_key)
二、解析天气网站数据
解析天气网站数据需要使用Python的网络爬虫技术。常用的库包括BeautifulSoup和Scrapy。此方法需要对HTML结构有一定的了解,并且要考虑网站的反爬策略。
1、使用BeautifulSoup解析网页
BeautifulSoup是一个方便解析HTML和XML的Python库。可以用它来解析天气网站的页面,提取天气信息。
- 发送HTTP请求获取网页内容:使用requests库发送请求,获取网页HTML。
- 解析HTML结构:使用BeautifulSoup解析HTML,找到包含天气数据的标签。
- 提取天气信息:从标签中提取所需的天气信息。
示例代码如下:
import requests
from bs4 import BeautifulSoup
def get_weather_from_website(city_name):
url = f"https://www.example.com/weather/{city_name}" # 替换为实际天气网站的URL
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
temperature = soup.find("span", class_="temperature").text
weather_description = soup.find("div", class_="weather-description").text
print(f"Temperature: {temperature}")
print(f"Weather Description: {weather_description}")
get_weather_from_website("London")
2、使用Scrapy进行爬虫
Scrapy是一个功能强大的爬虫框架,适用于大规模数据采集。它可以配置爬虫规则,从多个页面提取数据。
- 创建Scrapy项目:使用Scrapy命令行工具创建项目。
- 定义爬虫规则:在爬虫文件中定义爬取的URL和提取数据的规则。
- 解析和提取数据:使用Scrapy提供的解析工具提取天气数据。
由于Scrapy的使用较为复杂,这里不提供详细代码示例,但可以参考Scrapy官方文档进行学习。
三、使用Python库
一些Python库封装了天气API的调用,使得获取天气数据更加方便。这些库通常提供了简单的接口,直接调用即可获取天气信息。
1、使用pyowm库
pyowm是一个封装了OpenWeatherMap API的Python库,简化了API调用过程。使用pyowm可以快速获取天气数据。
- 安装pyowm库:使用pip命令安装pyowm库。
- 使用pyowm获取天气数据:创建pyowm对象,调用相关方法获取天气信息。
示例代码如下:
from pyowm import OWM
def get_weather_with_pyowm(city_name, api_key):
owm = OWM(api_key)
mgr = owm.weather_manager()
observation = mgr.weather_at_place(city_name)
weather = observation.weather
print(f"Temperature: {weather.temperature('celsius')['temp']}")
print(f"Weather Description: {weather.detailed_status}")
print(f"Wind Speed: {weather.wind()['speed']}")
print(f"Humidity: {weather.humidity}")
api_key = "your_api_key" # 替换为你的API密钥
get_weather_with_pyowm("London", api_key)
2、使用weather-api库
weather-api是另一个方便的库,支持多个天气API。它提供了统一的接口,可以根据需要选择不同的API。
- 安装weather-api库:使用pip命令安装weather-api库。
- 配置API和获取天气数据:选择所需的API,配置API密钥,调用相关方法获取天气信息。
示例代码如下:
from weather import Weather, Unit
def get_weather_with_weather_api(city_name):
weather = Weather(unit=Unit.CELSIUS)
location = weather.lookup_by_location(city_name)
condition = location.condition
print(f"Temperature: {condition.temp}")
print(f"Weather Description: {condition.text}")
get_weather_with_weather_api("London")
四、总结
获取实时天气数据在Python中有多种实现方式。调用天气API是最常用的方式,简单方便,适合大多数应用场景。解析天气网站数据需要一定的网页解析技术,适合于获取特定网站的数据。使用Python库则提供了更高的抽象层次,简化了API调用过程。根据需求选择合适的方式,可以有效获取所需的天气信息。
相关问答FAQs:
如何使用Python获取实时天气数据?
可以通过调用天气API来获取实时天气数据。常用的天气API包括OpenWeatherMap、WeatherAPI和WeatherStack等。你需要注册一个账号,获取API密钥,然后使用Python的requests库发送HTTP请求,解析返回的JSON数据来提取所需的天气信息。
使用哪些库可以简化获取天气的过程?
可以使用requests
库来发送网络请求,json
库来处理返回的数据。如果希望更方便,可以考虑使用第三方库如pyowm
,它是OpenWeatherMap的一个Python封装库,提供了更简洁的接口和方法来获取天气信息。
如何处理获取到的天气数据并进行可视化?
获取到的天气数据通常是以JSON格式返回,可以使用Python的pandas
库将其转换为数据框格式,方便进行数据分析和处理。为了可视化,可以使用matplotlib
或seaborn
等库,将温度、湿度、风速等信息通过图表形式展示,以便于更直观地理解天气变化。
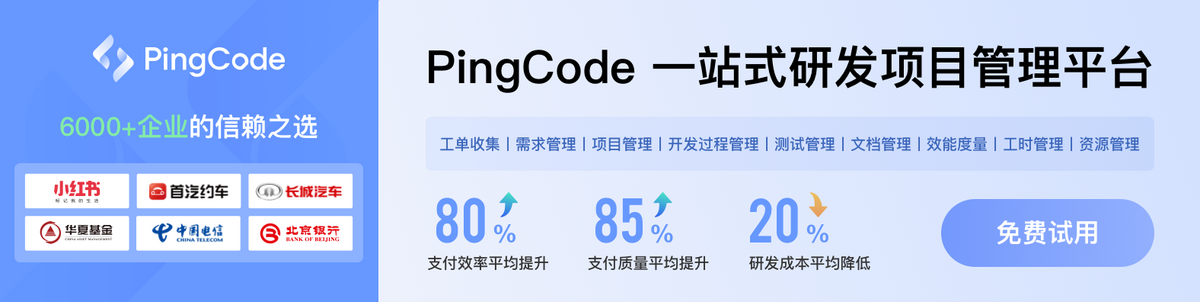