Python编写货币转换可以通过使用API获取实时汇率、使用开源库进行计算、实现简单的计算功能。其中,使用API获取实时汇率是最常见的方法,因为它能确保转换的准确性和及时性。通过调用外部API服务,如Forex或Open Exchange Rates,可以轻松获取最新的汇率数据,并将其用于货币转换。同时,Python中有多个开源库(如forex-python)可以帮助简化这一过程。下面,我将详细介绍如何实现这些方法。
一、使用API获取实时汇率
使用API获取实时汇率是实现货币转换的最直接和精确的方法。以下是一个使用Forex Python库与API结合的示例:
1、选择合适的API
首先,选择一个提供汇率数据的API。常用的API包括:
- Open Exchange Rates:提供全球多种货币的汇率数据。需要注册并获取API密钥。
- Fixer.io:提供欧元为基准的汇率数据,同样需要API密钥。
- Currency Layer:提供美元为基准的实时汇率数据。
这些API通常提供不同的价格计划,免费计划可能会有一些限制,如请求频率或可用货币数量。
2、获取API密钥并编写代码
一旦选择了API,并获得API密钥,就可以开始编写代码。以下是一个使用requests库与API进行交互的示例:
import requests
def get_exchange_rate(api_key, base_currency, target_currency):
url = f"https://openexchangerates.org/api/latest.json?app_id={api_key}&base={base_currency}"
response = requests.get(url)
data = response.json()
rate = data['rates'][target_currency]
return rate
def convert_currency(api_key, amount, base_currency, target_currency):
rate = get_exchange_rate(api_key, base_currency, target_currency)
converted_amount = amount * rate
return converted_amount
示例用法
api_key = 'YOUR_API_KEY'
amount = 100
base_currency = 'USD'
target_currency = 'EUR'
converted_amount = convert_currency(api_key, amount, base_currency, target_currency)
print(f"{amount} {base_currency} is equal to {converted_amount} {target_currency}")
在上面的代码中,使用了requests
库来进行HTTP请求,以获取汇率数据。get_exchange_rate
函数通过API获取指定货币对的汇率,而convert_currency
函数则根据该汇率将指定金额转换为目标货币。
二、使用开源库进行计算
使用Python开源库可以大大简化货币转换的过程。其中,forex-python
是一个流行的选择。
1、安装forex-python库
首先,通过pip安装forex-python库:
pip install forex-python
2、使用forex-python库进行货币转换
以下是一个使用forex-python库实现货币转换的示例:
from forex_python.converter import CurrencyRates
def convert_currency(amount, base_currency, target_currency):
c = CurrencyRates()
converted_amount = c.convert(base_currency, target_currency, amount)
return converted_amount
示例用法
amount = 100
base_currency = 'USD'
target_currency = 'EUR'
converted_amount = convert_currency(amount, base_currency, target_currency)
print(f"{amount} {base_currency} is equal to {converted_amount} {target_currency}")
在这个示例中,CurrencyRates
类用于获取货币汇率并进行转换,代码简洁且易于理解。
三、实现简单的计算功能
如果不需要实时汇率,可以选择手动输入汇率并进行简单的计算。这种方法适用于汇率较为稳定或不需要频繁更新的场景。
1、定义汇率并编写转换函数
以下是一个简单的手动汇率货币转换示例:
def convert_currency(amount, base_currency, target_currency, exchange_rate):
converted_amount = amount * exchange_rate
return converted_amount
示例用法
amount = 100
base_currency = 'USD'
target_currency = 'EUR'
exchange_rate = 0.85 # 手动设置汇率
converted_amount = convert_currency(amount, base_currency, target_currency, exchange_rate)
print(f"{amount} {base_currency} is equal to {converted_amount} {target_currency}")
在这个示例中,exchange_rate
参数用于指定汇率,convert_currency
函数则根据该汇率进行转换。
四、考虑汇率波动和更新机制
对于需要频繁更新的应用程序,考虑定期获取最新汇率并更新转换逻辑。例如,可以使用定时任务定期调用API,并将最新汇率存储在本地数据库中,以便在不调用API的情况下进行快速转换。
五、实现多货币转换功能
如果需要支持多货币转换,可以扩展上述代码以支持多个货币对。以下是一个实现多货币转换的示例:
from forex_python.converter import CurrencyRates
def convert_currency(amount, base_currency, target_currencies):
c = CurrencyRates()
results = {}
for target_currency in target_currencies:
converted_amount = c.convert(base_currency, target_currency, amount)
results[target_currency] = converted_amount
return results
示例用法
amount = 100
base_currency = 'USD'
target_currencies = ['EUR', 'GBP', 'JPY']
converted_amounts = convert_currency(amount, base_currency, target_currencies)
for currency, converted_amount in converted_amounts.items():
print(f"{amount} {base_currency} is equal to {converted_amount} {currency}")
在这个示例中,convert_currency
函数接收一个货币列表,并将指定金额转换为每种目标货币。这种方法适用于需要同时查看多种货币转换结果的场景。
六、总结
编写货币转换程序时,重要的是选择合适的方法和工具。使用API获取实时汇率是最为精确和常用的方法,而结合Python库如forex-python
可以大大简化开发过程。同时,考虑到汇率的波动性,定期更新汇率数据是确保转换准确性的关键。此外,手动输入汇率适用于汇率稳定的场景。无论选择哪种方法,都应根据具体需求进行灵活实现。
相关问答FAQs:
如何在Python中实现货币转换功能?
要在Python中实现货币转换,您可以使用汇率API来获取实时汇率数据。首先,您需要选择一个API提供者并注册以获取API密钥。使用requests
库获取数据后,可以根据输入的金额和货币类型进行计算,最后输出转换后的金额。
有哪些Python库可以帮助进行货币转换?
在Python中,有几个库可以帮助您进行货币转换,例如forex-python
和currencyconverter
。这些库提供了简单易用的接口,可以获取实时汇率并进行转换。只需安装相关库,并调用相应的函数即可轻松完成货币转换。
如何处理货币转换中的异常情况?
在编写货币转换程序时,处理异常情况非常重要。您需要考虑网络请求失败、无效的货币代码和负数金额等问题。通过使用try-except
语句捕获异常,可以确保程序的稳健性,并提供用户友好的错误提示。这样,用户在输入错误信息时,程序能够正常提示并引导用户进行修正。
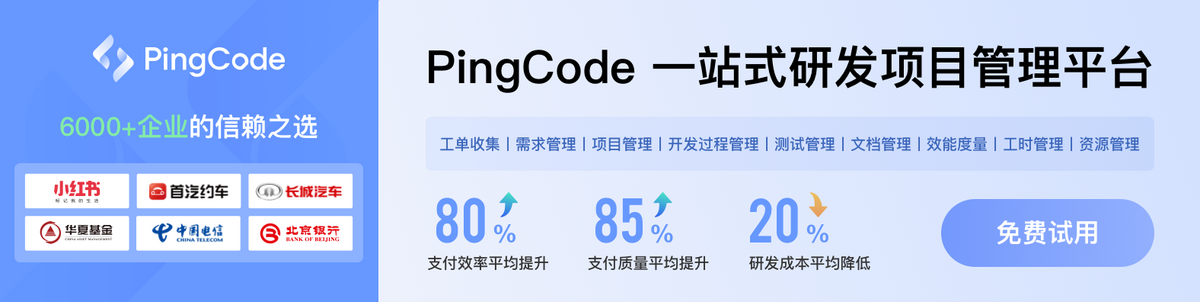