使用Python传输文字可以通过多种方式实现,如使用套接字通信、HTTP请求或消息队列等。其中,使用套接字是最常用的方法,因为它可以在两台计算机之间建立直接连接,实现高效的数据传输。下面将详细介绍如何通过套接字传输文字。
Python是一种高级编程语言,提供了丰富的库和模块,可以方便地实现文字传输。通过使用Python的内置库,如socket
、requests
和http.server
,开发者可以在不同的应用场景中实现文字的传输。在这里,我们将重点介绍如何通过套接字来实现文字传输,因为它是一种底层、灵活且高效的方式。
一、使用SOCKET实现文字传输
1.1 创建服务器
在Python中,可以使用socket
库创建一个服务器来接收和发送文字。首先,需要创建一个套接字对象并绑定到指定的地址和端口。
import socket
def create_server(host='localhost', port=12345):
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((host, port))
server_socket.listen(1)
print(f"Server listening on {host}:{port}")
while True:
client_socket, client_address = server_socket.accept()
print(f"Connection from {client_address}")
data = client_socket.recv(1024).decode('utf-8')
print(f"Received: {data}")
client_socket.sendall("Message received".encode('utf-8'))
client_socket.close()
Run the server
create_server()
1.2 创建客户端
客户端同样使用socket
库进行连接和数据传输。客户端创建一个套接字对象,并连接到服务器的地址和端口。
import socket
def create_client(host='localhost', port=12345, message="Hello, Server!"):
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((host, port))
client_socket.sendall(message.encode('utf-8'))
response = client_socket.recv(1024).decode('utf-8')
print(f"Received from server: {response}")
client_socket.close()
Run the client
create_client()
二、使用HTTP请求传输文字
2.1 使用FLASK搭建简单HTTP服务器
Flask是一个轻量级的Python Web框架,可以快速搭建一个HTTP服务器来处理文字传输。
from flask import Flask, request
app = Flask(__name__)
@app.route('/send', methods=['POST'])
def send_message():
data = request.json
print(f"Received message: {data['message']}")
return {"status": "Message received"}
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=5000)
2.2 使用REQUESTS发送HTTP请求
requests
库是Python中常用的HTTP请求库,可以方便地发送POST请求。
import requests
def send_message(url="http://localhost:5000/send", message="Hello, HTTP Server!"):
response = requests.post(url, json={"message": message})
print(response.json())
Send a message
send_message()
三、使用消息队列传输文字
3.1 使用RABBITMQ
RabbitMQ是一种消息队列系统,适合在分布式系统中传输文字和其他数据。可以使用pika
库与RabbitMQ进行交互。
import pika
def send_message_rabbitmq(message="Hello, RabbitMQ!"):
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='hello')
channel.basic_publish(exchange='', routing_key='hello', body=message)
print(f"Sent '{message}'")
connection.close()
def receive_message_rabbitmq():
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='hello')
def callback(ch, method, properties, body):
print(f"Received {body.decode('utf-8')}")
channel.basic_consume(queue='hello', on_message_callback=callback, auto_ack=True)
print('Waiting for messages. To exit press CTRL+C')
channel.start_consuming()
Send a message
send_message_rabbitmq()
Receive messages
receive_message_rabbitmq() # Uncomment to run the receiver
四、通过文件传输文字
4.1 使用FTP协议
FTP(File Transfer Protocol)是一种用于文件传输的协议,可以使用Python的ftplib
库进行实现。
from ftplib import FTP
def upload_file_to_ftp(server='ftp.example.com', username='user', password='pass', filepath='file.txt'):
ftp = FTP(server)
ftp.login(user=username, passwd=password)
with open(filepath, 'rb') as file:
ftp.storbinary(f'STOR {filepath}', file)
ftp.quit()
print(f"Uploaded {filepath} to FTP server")
def download_file_from_ftp(server='ftp.example.com', username='user', password='pass', filepath='file.txt'):
ftp = FTP(server)
ftp.login(user=username, passwd=password)
with open(f'downloaded_{filepath}', 'wb') as file:
ftp.retrbinary(f'RETR {filepath}', file.write)
ftp.quit()
print(f"Downloaded {filepath} from FTP server")
Upload a file
upload_file_to_ftp()
Download a file
download_file_from_ftp()
五、总结
通过以上几种方式,可以在Python中实现文字的传输。套接字提供了底层、高效的通信方式,适合在需要实时传输的情况下使用;HTTP请求适合在Web应用中使用;消息队列系统如RabbitMQ适合在分布式系统中使用,而FTP协议则适合于文件的上传和下载。每种方法都有其优点和适用的场景,开发者可以根据具体需求选择适合的传输方式。
相关问答FAQs:
如何使用Python实现文字的网络传输?
在Python中,可以通过多种方式实现文字的网络传输。常用的方法包括使用socket编程、HTTP请求或使用第三方库如Flask或Django来创建API。使用socket时,您可以创建一个简单的客户端和服务器架构,通过网络发送和接收文本数据。HTTP请求可以通过requests库轻松实现,将文字发送到RESTful API。对于更复杂的应用,Flask和Django提供了强大的框架来处理文字的传输和存储。
在Python中是否可以加密传输的文字?
是的,Python提供了多种库来加密和解密数据,如cryptography和PyCryptodome。这些库允许开发者在传输文字之前对其进行加密,确保传输过程中的数据安全性。您可以使用对称加密或非对称加密算法,根据需要选择合适的方案。加密后的文字在网络传输时,即使被截获,也难以被理解。
如何在Python中处理大批量文字的传输?
处理大批量文字的传输时,可以考虑使用流式传输技术。这种方法允许将数据分块传输,从而减少内存使用和提高效率。在Python中,可以使用生成器函数来实现流式读取和发送文本数据。此外,使用异步编程(如asyncio库)也可以有效地处理高并发的文字传输需求,显著提高性能和响应速度。
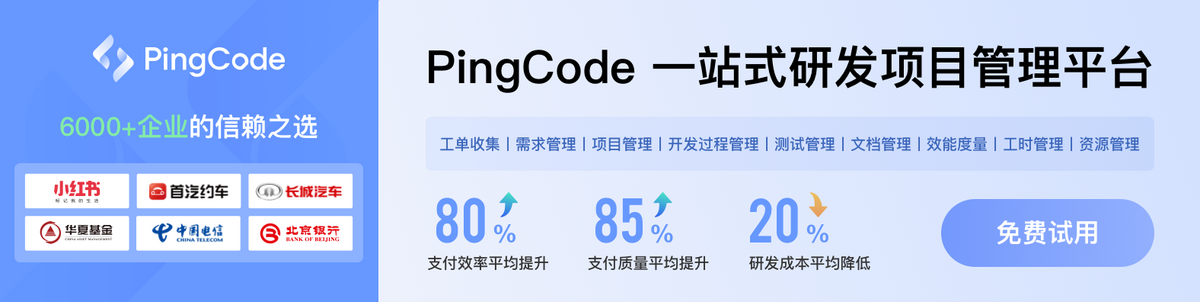