在Python中使用正则表达式进行匹配可以通过re
模块来实现,它提供了许多函数用于搜索、匹配和替换字符串中的模式。常用的正则表达式函数包括re.match()
、re.search()
、re.findall()
、re.finditer()
、re.sub()
。其中,re.match()
用于从字符串的开头进行匹配,re.search()
用于在整个字符串中搜索匹配,re.findall()
用于查找字符串中所有匹配的子串,re.finditer()
返回一个迭代器,re.sub()
用于替换匹配到的内容。例如,在使用re.search()
函数时,你可以通过指定正则表达式模式来在字符串中查找匹配的内容,并通过返回的匹配对象来获取匹配的详细信息。
正则表达式是一个非常强大的工具,可以用于处理字符串中的复杂模式匹配。Python的re
模块使得正则表达式的使用变得非常简单和高效。在本文中,我们将深入探讨Python中如何使用正则表达式进行匹配,包括常用函数的使用、正则表达式的基本语法和高级用法。
一、正则表达式的基础
正则表达式是一种用于描述和匹配字符串模式的工具。它可以用来验证输入、搜索、提取信息等。在Python中,正则表达式通过re
模块进行使用。
re
模块的引入
在使用正则表达式之前,需要先导入re
模块。可以通过以下方式导入:
import re
- 基本的正则表达式语法
- 字符:匹配自身,如
a
匹配字符a
。 - 点号
.
:匹配任意单个字符,除了换行符。 - 星号
*
:匹配前一个字符零次或多次。 - 加号
+
:匹配前一个字符一次或多次。 - 问号
?
:匹配前一个字符零次或一次。 - 方括号
[]
:匹配方括号内的任意字符。 - 反斜杠
:用于转义特殊字符。
- 管道符
|
:表示逻辑或。 - 小括号
()
:用于分组和提取。
二、正则表达式的常用函数
Python中的re
模块提供了多个函数用于正则表达式的匹配和处理。
re.match()
用于从字符串的起始位置开始匹配,如果起始位置不匹配,则返回None
。例如:
import re
pattern = r'^hello'
text = 'hello world'
match = re.match(pattern, text)
if match:
print('Match found:', match.group())
else:
print('No match')
re.search()
在字符串中搜索匹配,返回第一个匹配的对象,如果没有匹配则返回None
。例如:
import re
pattern = r'world'
text = 'hello world'
match = re.search(pattern, text)
if match:
print('Match found:', match.group())
else:
print('No match')
re.findall()
返回字符串中所有与模式匹配的子串列表。示例如下:
import re
pattern = r'\d+'
text = 'There are 3 apples and 5 oranges'
numbers = re.findall(pattern, text)
print('Numbers found:', numbers)
re.finditer()
返回一个迭代器,遍历每个匹配的对象。示例如下:
import re
pattern = r'\d+'
text = 'There are 3 apples and 5 oranges'
matches = re.finditer(pattern, text)
for match in matches:
print('Match found:', match.group())
re.sub()
用于替换匹配的子串。示例如下:
import re
pattern = r'apples'
replacement = 'bananas'
text = 'I like apples'
new_text = re.sub(pattern, replacement, text)
print('Replaced text:', new_text)
三、正则表达式的高级用法
正则表达式不仅可以用于简单的匹配,还可以用于更复杂的字符串处理。
- 分组和捕获
通过小括号()
来分组,可以在匹配时捕获相关的子串。例如:
import re
pattern = r'(hello) (world)'
text = 'hello world'
match = re.search(pattern, text)
if match:
print('Group 1:', match.group(1))
print('Group 2:', match.group(2))
- 非捕获分组
使用(?:...)
进行非捕获分组,这样做不会保存匹配的子串。例如:
import re
pattern = r'(?:hello) (world)'
text = 'hello world'
match = re.search(pattern, text)
if match:
print('Group 1:', match.group(1)) # Only one group is captured
- 前瞻和后顾
前瞻和后顾用于在匹配时进行条件限制。前瞻用(?=...)
表示,后顾用(?<=...)
表示。例如:
import re
前瞻
pattern = r'\d+(?= apples)'
text = 'There are 10 apples'
match = re.search(pattern, text)
if match:
print('Matched number:', match.group())
后顾
pattern = r'(?<=There are )\d+'
text = 'There are 10 apples'
match = re.search(pattern, text)
if match:
print('Matched number:', match.group())
四、正则表达式的特殊字符和转义
正则表达式中有许多特殊字符,它们具有特定的含义。如果要匹配这些字符本身,需要使用反斜杠进行转义。
- 特殊字符
包括. ^ $ * + ? { } [ ] \ | ( )
等。
- 转义字符
可以通过反斜杠来转义特殊字符。例如,要匹配字符*
,可以使用\*
。
import re
pattern = r'\*'
text = '3 * 5 = 15'
match = re.search(pattern, text)
if match:
print('Matched character:', match.group())
五、正则表达式的性能优化
在处理较长的字符串或复杂的匹配模式时,正则表达式的性能可能会受到影响。以下是一些优化建议:
- 使用
re.compile()
在需要多次使用同一正则表达式时,可以使用re.compile()
来预编译正则表达式,从而提高效率。
import re
pattern = re.compile(r'\d+')
text = 'There are 3 apples and 5 oranges'
numbers = pattern.findall(text)
print('Numbers found:', numbers)
- 避免过于复杂的模式
尽量简化正则表达式,避免使用过多的嵌套和复杂的条件。
- 使用非贪婪匹配
默认情况下,正则表达式是贪婪匹配的,即尽可能多地匹配字符。可以通过在量词后加问号?
来实现非贪婪匹配,从而提高效率。
import re
pattern = r'<.*?>'
text = '<tag>content</tag>'
matches = re.findall(pattern, text)
print('Matches:', matches)
六、正则表达式的实际应用
正则表达式广泛应用于文本处理、数据提取和格式验证等场景。
- 电子邮件验证
可以使用正则表达式验证电子邮件地址的格式。例如:
import re
pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'
email = 'test@example.com'
if re.match(pattern, email):
print('Valid email')
else:
print('Invalid email')
- URL提取
可以使用正则表达式从文本中提取URL。例如:
import re
pattern = r'https?://(?:[-\w.]|(?:%[\da-fA-F]{2}))+'
text = 'Visit https://www.example.com and http://www.test.com'
urls = re.findall(pattern, text)
print('URLs found:', urls)
- 数据清理
在数据处理中,正则表达式可以用于清理和格式化数据。例如,去除多余的空格和特殊字符。
import re
text = ' Hello, World! '
cleaned_text = re.sub(r'\s+', ' ', text).strip()
print('Cleaned text:', cleaned_text)
七、总结
Python中的正则表达式是一个强大而灵活的工具,能够处理各种复杂的字符串匹配任务。通过re
模块提供的函数,可以方便地进行模式匹配、搜索和替换操作。理解和掌握正则表达式的基础语法和高级用法,可以帮助我们在数据处理和文本分析中更加高效地解决问题。在实际应用中,我们应注意正则表达式的性能优化和适用场景,以便充分发挥其优势。
相关问答FAQs:
如何在Python中使用正则表达式进行字符串搜索?
在Python中,可以使用re
模块来执行正则表达式操作。通过调用re.search()
函数,可以在字符串中查找符合特定模式的内容。该函数返回一个匹配对象,如果没有找到匹配项,则返回None
。使用示例:
import re
pattern = r'\d+' # 匹配一个或多个数字
text = 'There are 123 apples'
match = re.search(pattern, text)
if match:
print('Found:', match.group())
在Python中如何替换字符串中的特定模式?
要替换字符串中的特定模式,可以使用re.sub()
函数。该函数接受三个主要参数:要替换的模式、替换的字符串和目标字符串。可以通过这个方法轻松地进行文本的批量替换。示例代码如下:
import re
pattern = r'apples'
replacement = 'oranges'
text = 'There are 123 apples'
new_text = re.sub(pattern, replacement, text)
print('Updated text:', new_text)
使用Python中的正则表达式时,如何处理特殊字符?
在正则表达式中,一些字符具有特殊含义,比如.
、*
、?
等。如果需要匹配这些特殊字符本身,可以使用反斜杠(\
)进行转义。例如,要匹配一个点号,可以使用r'\.'
。此外,可以使用re.escape()
函数自动对字符串中的所有特殊字符进行转义,确保在匹配时不会引起错误。示例代码如下:
import re
text = 'This is a dot: .'
escaped_pattern = re.escape('.')
match = re.search(escaped_pattern, text)
if match:
print('Dot found!')
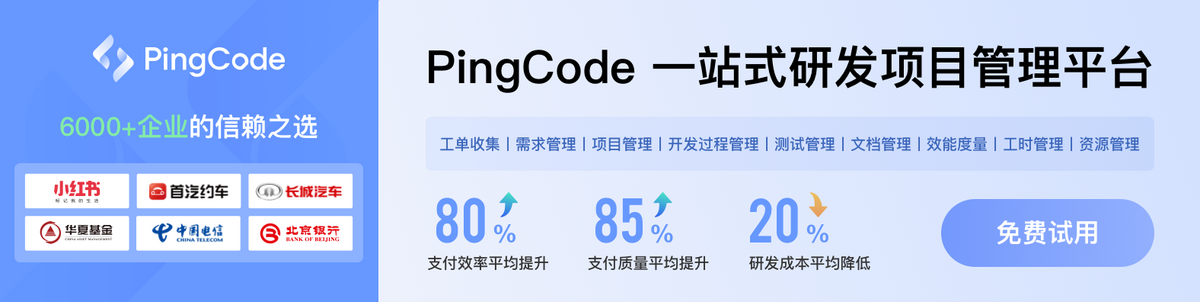