要用Python建立文件,可以使用open()函数、with语句、write()方法。其中,open()函数用于打开或创建文件,with语句确保文件使用完后自动关闭,write()方法用于写入内容。open()函数的第一个参数是文件名,第二个参数是模式(如'w'表示写入模式)。例如:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
这段代码创建了一个名为example.txt的文件,并在其中写入了“Hello, World!”这句话。使用with语句的好处是可以自动管理文件的打开和关闭,避免文件泄露和占用资源。
一、使用OPEN()函数创建文件
open()函数是Python内置的函数,用于打开文件并返回文件对象。在创建文件时,open()函数的第二个参数用于指定文件的打开模式。常用的模式包括:
- 'w':写入模式。如果文件不存在,将创建一个新文件;如果文件已存在,将覆盖文件的内容。
- 'a':追加模式。如果文件不存在,将创建一个新文件;如果文件已存在,数据将被追加到文件末尾。
- 'x':创建模式。如果文件不存在,将创建一个新文件;如果文件已存在,将引发FileExistsError。
使用open()函数创建文件的基本步骤如下:
- 调用open()函数,传入文件名和模式。
- 使用write()方法写入数据。
- 调用close()方法关闭文件。
file = open('data.txt', 'w')
file.write('This is a new file created using open() function.')
file.close()
这种方法需要手动关闭文件,否则可能会导致文件未保存或资源泄露。
二、WITH语句管理文件对象
为了解决文件关闭的问题,Python提供了with语句,它能自动管理文件的打开和关闭。使用with语句,可以简化代码并提高安全性。
with open('data.txt', 'w') as file:
file.write('This is a new file created using with statement.')
在with块中,文件对象是有效的,块结束后,文件会被自动关闭。这种方法简洁、直观,推荐用于文件操作。
三、WRITE()方法写入内容
write()方法是文件对象的方法,用于将字符串写入文件。使用write()方法时,需要注意以下几点:
- write()方法只能写入字符串。如果要写入其他数据类型,需要先转换为字符串。
- write()方法不会在数据后自动添加换行符。若要换行,需要手动添加'\n'。
- 在写入大量数据时,可以使用writelines()方法,该方法接受一个字符串列表,将列表中的每个字符串写入文件。
lines = ['Line 1\n', 'Line 2\n', 'Line 3\n']
with open('data.txt', 'w') as file:
file.writelines(lines)
四、在不同模式下创建文件
根据实际需求,可以选择不同的模式创建文件。以下是几种常见的使用场景:
- 写入模式('w'):适用于创建新文件或覆盖旧文件。使用这种模式时,如果文件已存在,文件内容将被清空。
with open('overwrite.txt', 'w') as file:
file.write('This will overwrite the existing content.')
- 追加模式('a'):适用于在文件末尾追加内容而不影响现有内容。
with open('append.txt', 'a') as file:
file.write('\nThis line will be appended to the file.')
- 创建模式('x'):适用于确保文件不存在时创建文件,如果文件已存在,将抛出异常。
try:
with open('create.txt', 'x') as file:
file.write('This file is created only if it does not exist.')
except FileExistsError:
print('File already exists.')
五、读取文件内容
在创建文件后,通常需要读取文件内容进行验证或处理。Python提供了多种读取文件内容的方法:
- read()方法:读取整个文件内容,返回一个字符串。
with open('data.txt', 'r') as file:
content = file.read()
print(content)
- readline()方法:一次读取一行,常用于逐行处理文件。
with open('data.txt', 'r') as file:
while True:
line = file.readline()
if not line:
break
print(line.strip())
- readlines()方法:读取文件所有行,返回一个列表,每行作为列表的一个元素。
with open('data.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
六、使用其他文件库
除了内置函数,Python还有许多第三方库可以用于文件操作,如pandas、numpy、openpyxl等。这些库提供了更高级的功能,适合处理复杂的数据文件。
- pandas库:常用于处理CSV、Excel文件。pandas提供了read_csv()和to_csv()方法,可以方便地读写CSV文件。
import pandas as pd
创建DataFrame并写入CSV文件
df = pd.DataFrame({'Name': ['Alice', 'Bob'], 'Age': [25, 30]})
df.to_csv('people.csv', index=False)
读取CSV文件
df = pd.read_csv('people.csv')
print(df)
- openpyxl库:用于处理Excel文件。openpyxl可以创建、修改和读取Excel文件。
from openpyxl import Workbook
创建Excel文件并写入数据
wb = Workbook()
ws = wb.active
ws['A1'] = 'Hello'
ws['B1'] = 'World'
wb.save('hello_world.xlsx')
- numpy库:擅长处理数值数据。numpy可以读写文本文件和二进制文件。
import numpy as np
创建数组并保存到文本文件
array = np.array([[1, 2, 3], [4, 5, 6]])
np.savetxt('array.txt', array)
从文本文件读取数组
loaded_array = np.loadtxt('array.txt')
print(loaded_array)
七、处理文件路径
在进行文件操作时,文件路径是一个重要的概念。Python的os模块提供了处理文件路径的功能。
- 获取文件路径:os.path模块可以获取文件的绝对路径、目录名和文件名。
import os
file_path = 'data.txt'
abs_path = os.path.abspath(file_path)
dir_name = os.path.dirname(abs_path)
base_name = os.path.basename(abs_path)
print(f'Absolute Path: {abs_path}')
print(f'Directory Name: {dir_name}')
print(f'Base Name: {base_name}')
- 检查文件或目录:os.path模块可以检查文件或目录是否存在。
if os.path.exists('data.txt'):
print('File exists.')
else:
print('File does not exist.')
- 创建目录:os模块提供了创建目录的功能。
dir_name = 'new_folder'
if not os.path.exists(dir_name):
os.makedirs(dir_name)
print(f'Directory {dir_name} created.')
else:
print(f'Directory {dir_name} already exists.')
八、处理文件异常
在进行文件操作时,可能会遇到各种异常(例如,文件不存在、权限不足)。Python提供了异常处理机制,可以捕获和处理这些异常。
try:
with open('non_existent_file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('The file does not exist.')
except PermissionError:
print('You do not have permission to access this file.')
except Exception as e:
print(f'An error occurred: {e}')
通过使用try-except块,可以有效处理文件操作中的异常,确保程序的健壮性。
九、使用上下文管理器
除了使用with语句,Python还允许自定义上下文管理器来管理文件资源。上下文管理器实现了__enter__()和__exit__()方法,可以灵活地管理资源。
class FileManager:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
with FileManager('data.txt', 'w') as file:
file.write('Using a custom context manager to manage file resources.')
自定义上下文管理器可以让资源管理更加灵活和简洁。
十、文件操作性能优化
在处理大型文件时,性能可能成为一个问题。以下是一些优化文件操作性能的方法:
- 使用缓冲区:在打开文件时,可以指定缓冲区大小来提高文件读写性能。
with open('large_file.txt', 'r', buffering=2048) as file:
content = file.read()
- 批量处理:使用生成器或iter()方法可以减少内存使用,提高处理速度。
def read_in_chunks(file_object, chunk_size=1024):
while True:
data = file_object.read(chunk_size)
if not data:
break
yield data
with open('large_file.txt', 'r') as file:
for chunk in read_in_chunks(file):
print(chunk)
- 多线程或多进程:对于I/O密集型任务,可以使用多线程或多进程来提高效率。
import threading
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(content)
threads = []
for i in range(5):
thread = threading.Thread(target=read_file, args=('data.txt',))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
通过合理使用Python的文件操作功能,可以高效地创建和管理文件,满足各种应用场景的需求。
相关问答FAQs:
如何在Python中创建一个文本文件?
在Python中创建文本文件非常简单。您可以使用内置的open()
函数,指定文件名和模式。使用'w'
模式可以创建一个新的文件,如果文件已存在,则会覆盖它。以下是一个示例代码:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
这段代码会创建一个名为example.txt
的文件,并在其中写入“Hello, World!”。
在Python中如何添加内容到已存在的文件?
若要向已存在的文件添加内容,而不是覆盖它,可以使用'a'
模式(附加模式)。这样您可以在文件的末尾写入数据。示例代码如下:
with open('example.txt', 'a') as file:
file.write('\nAppending a new line.')
这将把“Appending a new line.”添加到example.txt
文件的末尾。
如何处理文件创建过程中可能出现的错误?
在创建文件时,可能会遇到各种错误,例如权限问题或磁盘空间不足。可以使用try
和except
块来捕获这些异常。以下是一个处理文件创建错误的示例:
try:
with open('example.txt', 'w') as file:
file.write('Creating a file safely.')
except IOError as e:
print(f'An error occurred: {e}')
通过这种方式,您可以确保在出现问题时不会导致程序崩溃,同时可以获取错误信息以便后续处理。
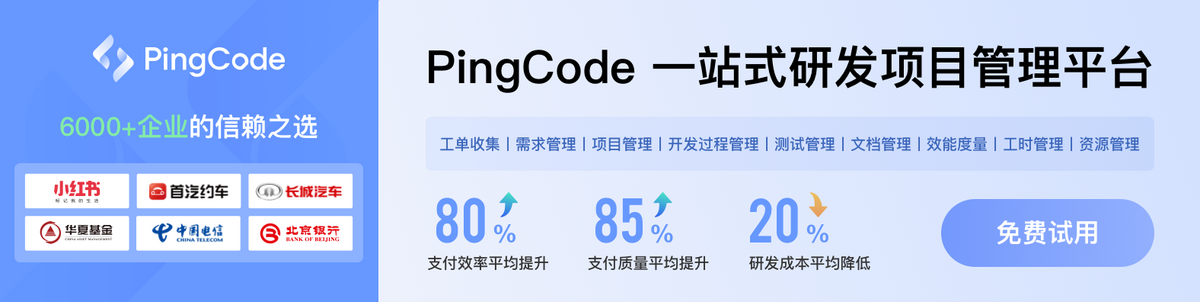