Python读取线程数据的方式主要包括使用线程间共享数据结构、使用线程同步机制和使用线程本地数据存储。其中,使用线程间共享数据结构是最常见的方法,比如使用queue.Queue
来在线程之间传递数据。线程同步机制可以确保线程安全地访问共享数据,比如使用threading.Lock
。线程本地数据存储提供了一种在线程中存储独立数据的方式,比如使用threading.local()
。下面将详细描述这些方法中的一种:使用queue.Queue
。
queue.Queue
是Python标准库中提供的一种线程安全的队列,它的主要特点是能够在多线程环境中安全地存储和传输数据。通过使用queue.Queue
,一个线程可以将数据放入队列中,而另一个线程则可以从队列中获取数据。queue.Queue
提供了put()
和get()
方法来实现数据的放入和取出,这些方法内部已经实现了线程同步,因此不需要额外的同步机制。使用queue.Queue
可以有效避免线程竞争和数据不一致的问题。此外,queue.Queue
还支持设置队列的最大长度,当队列已满时,put()
方法会阻塞,直到有空间可用;当队列为空时,get()
方法会阻塞,直到有数据可用。这种机制可以有效地协调生产者-消费者模式中的线程。
接下来的内容将详细介绍如何在Python中使用线程读取数据。
一、使用queue.Queue读取线程数据
在多线程编程中,线程之间的数据共享是一个常见的需求。queue.Queue
是Python中为了解决线程间数据传输而提供的一个模块。它是线程安全的,并且能够有效地避免线程竞争的问题。通过使用queue.Queue
,可以轻松实现生产者-消费者模式。
在生产者-消费者模式中,生产者线程负责生成数据并将其放入队列中,消费者线程则从队列中取出数据进行处理。使用queue.Queue
可以使得生产者和消费者线程独立地运行,而不必直接通信。生产者通过put()
方法将数据放入队列,消费者通过get()
方法从队列中取出数据。queue.Queue
内部已经处理了线程同步,因此不需要额外的锁机制。
import threading
import queue
import time
def producer(q):
for i in range(5):
print(f"Producing {i}")
q.put(i)
time.sleep(1)
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consuming {item}")
q.task_done()
q = queue.Queue()
thread1 = threading.Thread(target=producer, args=(q,))
thread2 = threading.Thread(target=consumer, args=(q,))
thread1.start()
thread2.start()
thread1.join()
q.put(None) # Signal the consumer to exit
thread2.join()
在这个例子中,我们创建了一个生产者线程和一个消费者线程。生产者线程将数据放入队列中,而消费者线程从队列中取出数据进行处理。当生产者完成任务后,向队列中放入一个None
,作为消费者退出的信号。
二、使用threading.Lock确保线程安全
在某些情况下,需要多个线程同时访问一个共享的资源,例如一个共享变量。为了避免线程之间的竞争条件,需要使用threading.Lock
来确保线程安全。threading.Lock
提供了简单的锁机制,允许一个线程独占访问共享资源。
使用threading.Lock
的基本方法是在线程访问共享资源之前调用acquire()
方法,访问完成后调用release()
方法。这样可以确保在同一时刻只有一个线程可以访问共享资源。
import threading
class Counter:
def __init__(self):
self.value = 0
self.lock = threading.Lock()
def increment(self):
with self.lock:
self.value += 1
counter = Counter()
def worker():
for _ in range(100000):
counter.increment()
threads = []
for _ in range(5):
thread = threading.Thread(target=worker)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(f'Final counter value: {counter.value}')
在这个例子中,Counter
类中包含一个共享变量value
,多个线程需要对其进行增量操作。为了确保线程安全,我们在增量操作中使用了with self.lock:
上下文管理器,这相当于在进入代码块之前调用acquire()
方法,退出代码块时自动调用release()
方法。这样可以确保每次只有一个线程可以执行增量操作,从而避免竞争条件。
三、使用threading.local()存储线程本地数据
在多线程编程中,除了共享数据外,有时还需要在每个线程中存储独立的数据。Python提供了threading.local()
来实现这一功能。threading.local()
创建的对象能够为每个线程存储独立的数据。
使用threading.local()
创建的对象类似于一个全局变量,但它的值是线程局部的。每个线程对该对象的访问仅限于该线程自身的上下文,因此不会与其他线程冲突。
import threading
local_data = threading.local()
def process_data():
print(f"Thread {threading.current_thread().name} has value {local_data.value}")
def worker(value):
local_data.value = value
process_data()
threads = []
for i in range(5):
thread = threading.Thread(target=worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在这个例子中,我们使用threading.local()
创建了一个线程本地的数据对象local_data
。每个线程在调用worker()
函数时,都会为local_data.value
赋予一个独立的值。process_data()
函数打印出当前线程的名称和其对应的local_data.value
值。
通过使用threading.local()
,可以轻松地在多线程程序中管理线程局部数据,而不需要担心线程之间的数据冲突问题。
四、线程间的信号传递
在多线程编程中,线程之间的信号传递是一个重要的功能。信号传递可以用于线程之间的同步、通信和协调。Python提供了多种方式来实现线程间的信号传递。
- 使用Event对象
threading.Event
对象是一个简单的同步原语,用于在多个线程之间发送信号。Event
对象内部维护了一个布尔标志,初始值为False
。线程可以通过调用set()
方法将标志设为True
,通过调用clear()
方法将标志设为False
。其他线程可以通过wait()
方法等待标志变为True
。
import threading
import time
def wait_for_event(e):
print("Thread waiting for event")
e.wait()
print("Event received")
event = threading.Event()
thread = threading.Thread(target=wait_for_event, args=(event,))
thread.start()
time.sleep(2)
event.set()
thread.join()
在这个例子中,wait_for_event()
函数中的线程会等待event
对象的标志变为True
。主线程在2秒后调用event.set()
,将标志设为True
,从而唤醒等待的线程。
- 使用Condition对象
threading.Condition
对象提供了更为复杂的线程间通信机制。Condition
对象允许一个或多个线程等待某个条件的发生,同时也允许其他线程通知这些等待的线程。
import threading
condition = threading.Condition()
data = []
def consumer():
with condition:
condition.wait() # Wait for a notification
print(f"Consumed {data.pop()}")
def producer():
with condition:
data.append(42)
condition.notify() # Notify waiting threads
thread1 = threading.Thread(target=consumer)
thread2 = threading.Thread(target=producer)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在这个例子中,消费者线程会等待condition
对象的通知。在生产者线程中,当数据准备好后,调用condition.notify()
通知消费者线程。
五、线程池的使用
在线程编程中,线程池是一种常用的技术,用于高效地管理线程的创建和销毁。Python的concurrent.futures
模块提供了ThreadPoolExecutor
类,用于创建和管理线程池。
ThreadPoolExecutor
允许用户提交多个任务给线程池,由线程池中的多个线程并发执行这些任务。用户可以通过submit()
方法提交任务,并获得一个Future
对象。通过Future
对象,可以检查任务的执行状态和获取任务的返回结果。
import concurrent.futures
import time
def task(n):
print(f"Task {n} starting")
time.sleep(2)
print(f"Task {n} completed")
return n * 2
with concurrent.futures.ThreadPoolExecutor(max_workers=3) as executor:
futures = [executor.submit(task, i) for i in range(5)]
for future in concurrent.futures.as_completed(futures):
result = future.result()
print(f"Result: {result}")
在这个例子中,我们使用ThreadPoolExecutor
创建了一个包含3个线程的线程池,并提交了5个任务。as_completed()
方法返回一个迭代器,按任务完成的顺序返回Future
对象。通过调用future.result()
方法,可以获取任务的返回结果。
六、线程的终止和异常处理
在多线程编程中,线程的终止和异常处理是两个重要的问题。Python的threading.Thread
类不提供直接终止线程的方法,因此需要通过线程间的信号或标志来实现线程的安全终止。
- 使用标志终止线程
通过在线程间使用共享的标志变量,可以实现线程的终止。线程在运行时定期检查标志变量的值,根据标志变量的值决定是否终止。
import threading
import time
class StoppableThread(threading.Thread):
def __init__(self):
super().__init__()
self.stop_flag = False
def run(self):
while not self.stop_flag:
print("Thread running")
time.sleep(1)
thread = StoppableThread()
thread.start()
time.sleep(5)
thread.stop_flag = True
thread.join()
在这个例子中,StoppableThread
类定义了一个stop_flag
标志变量。线程在运行时检查stop_flag
的值,当标志为True
时,线程会终止。
- 线程中的异常处理
在多线程编程中,异常的处理也非常重要。当线程中发生异常时,需要捕获异常并进行适当的处理。为了确保异常能够被捕获和处理,可以在run()
方法中使用try-except
块。
import threading
class ExceptionThread(threading.Thread):
def run(self):
try:
raise ValueError("An error occurred")
except Exception as e:
print(f"Exception caught: {e}")
thread = ExceptionThread()
thread.start()
thread.join()
在这个例子中,ExceptionThread
类在run()
方法中引发了一个ValueError
异常。通过在run()
方法中使用try-except
块,可以捕获异常并进行处理。
七、线程的调度和优先级
在线程编程中,线程的调度和优先级是一个复杂的话题。Python的threading
模块不提供直接的线程优先级设置方法,线程的调度由操作系统决定。然而,可以通过某些技巧来影响线程的执行顺序。
- 使用
time.sleep()
控制线程执行顺序
在某些情况下,可以使用time.sleep()
函数来控制线程的执行顺序。通过在线程中调用time.sleep()
,可以使线程暂停一段时间,从而影响线程的执行顺序。
import threading
import time
def task(name, delay):
print(f"Task {name} starting")
time.sleep(delay)
print(f"Task {name} completed")
thread1 = threading.Thread(target=task, args=("A", 2))
thread2 = threading.Thread(target=task, args=("B", 1))
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在这个例子中,虽然thread1
先启动,但由于其内部有更长的time.sleep()
时间,thread2
会先完成。
- 使用
threading.Event
协调线程执行
通过使用threading.Event
,可以实现线程之间的协调和同步,从而影响线程的执行顺序。
import threading
event = threading.Event()
def task1():
print("Task 1 starting")
event.wait()
print("Task 1 completed")
def task2():
print("Task 2 starting")
event.set()
print("Task 2 completed")
thread1 = threading.Thread(target=task1)
thread2 = threading.Thread(target=task2)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在这个例子中,task1
中的线程会等待event
对象的信号,而task2
中的线程会设置信号。这种方式可以用于协调线程的执行顺序。
八、线程的性能优化
在线程编程中,性能优化是一个重要的课题。通过合理地使用线程,可以提高程序的并发性能。然而,不当的使用也可能导致性能下降。以下是一些线程性能优化的建议:
- 减少线程的创建和销毁
线程的创建和销毁是一个昂贵的操作,频繁地创建和销毁线程会导致性能下降。可以通过使用线程池来减少线程的创建和销毁。
- 避免过多的线程
过多的线程会导致线程之间的竞争加剧,从而影响性能。在使用线程时,应根据任务的性质和系统的资源合理地设置线程的数量。
- 使用线程池
通过使用线程池,可以高效地管理线程的创建和销毁,从而提高程序的并发性能。Python的concurrent.futures
模块提供了ThreadPoolExecutor
类,用于创建和管理线程池。
- 合理使用锁
锁是确保线程安全的重要工具,但过多地使用锁会导致性能下降。应尽量缩小锁的范围,减少锁的持有时间。
- 避免死锁
死锁是多线程编程中的一个常见问题,会导致线程无法继续执行。在使用锁时,应注意避免死锁的发生。
- 使用多进程替代多线程
在CPU密集型任务中,Python的全局解释器锁(GIL)会限制线程的并发性能。在这种情况下,可以考虑使用多进程来替代多线程。Python的multiprocessing
模块提供了多进程的支持。
通过合理地使用线程技术,可以提高程序的并发性能。然而,在使用线程时,应注意线程的安全性和可控性,以避免潜在的问题。
相关问答FAQs:
如何在Python中创建和使用线程?
在Python中,可以使用threading
模块来创建和管理线程。通过定义一个继承自threading.Thread
的类,或使用threading.Thread
的实例化,可以在新的线程中运行特定的任务。使用start()
方法来启动线程,使用join()
方法可以确保主线程等待子线程完成。
Python中线程数据共享的最佳实践是什么?
在多线程环境中,数据共享可能导致竞争条件。使用threading.Lock
可以避免多个线程同时访问共享资源,从而确保数据的完整性。此外,可以考虑使用queue.Queue
来安全地在线程之间传递数据,这样可以避免手动管理锁的问题。
如何在线程中处理异常?
在Python的线程中,异常不会自动传播到主线程。为了捕获线程中的异常,可以在目标函数中使用try...except
语句。这样可以确保即使在发生错误时,程序也能稳定运行,并可以记录错误信息以供后续排查。
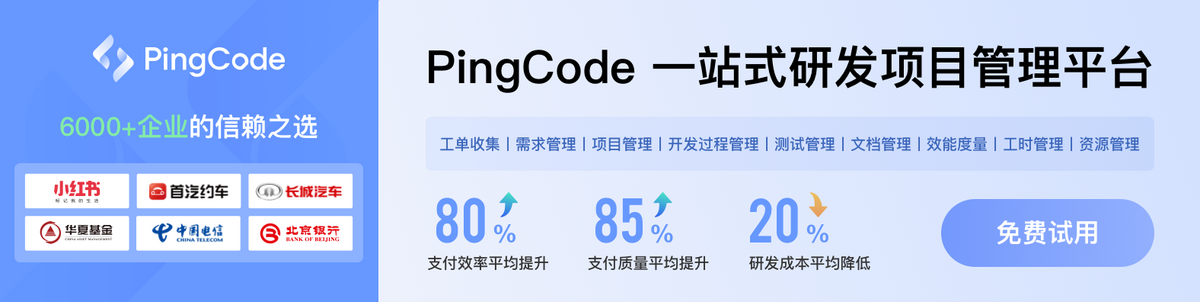