在Python中爬取PDF文档,有多种方法和工具可以使用,包括requests库、BeautifulSoup库、PyPDF2库、pdfminer库、Selenium库等。为了详细描述其中一种方法,我们将重点探讨如何使用requests库和BeautifulSoup库来下载PDF文件,并使用PyPDF2库来读取PDF内容。
一、安装必要的库
在开始之前,确保已经安装了所需的Python库。可以使用以下命令安装:
pip install requests beautifulsoup4 PyPDF2
二、使用Requests库和BeautifulSoup库爬取PDF文件
1、发送HTTP请求并解析HTML
首先,我们需要使用requests
库发送HTTP请求来获取网页内容,并使用BeautifulSoup
库解析HTML文档。这将帮助我们找到网页中所有的PDF链接。
import requests
from bs4 import BeautifulSoup
def get_pdf_links(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
pdf_links = []
for link in soup.find_all('a', href=True):
href = link['href']
if href.endswith('.pdf'):
pdf_links.append(href)
return pdf_links
url = 'http://example.com'
pdf_links = get_pdf_links(url)
print(f'Found {len(pdf_links)} PDF links')
2、下载PDF文件
接下来,我们将遍历所有找到的PDF链接,并使用requests
库下载这些PDF文件。
def download_pdfs(pdf_links, download_folder):
for link in pdf_links:
pdf_response = requests.get(link)
pdf_name = link.split('/')[-1]
with open(f'{download_folder}/{pdf_name}', 'wb') as pdf_file:
pdf_file.write(pdf_response.content)
print(f'Downloaded {pdf_name}')
download_folder = 'pdfs'
download_pdfs(pdf_links, download_folder)
三、使用PyPDF2库读取PDF内容
1、安装PyPDF2库
确保已经安装了PyPDF2
库,使用以下命令:
pip install PyPDF2
2、读取PDF文件内容
接下来,我们将使用PyPDF2
库读取下载的PDF文件内容。
import PyPDF2
def read_pdf(file_path):
with open(file_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
num_pages = reader.numPages
content = ''
for page_num in range(num_pages):
page = reader.getPage(page_num)
content += page.extract_text()
return content
pdf_file_path = 'pdfs/example.pdf'
pdf_content = read_pdf(pdf_file_path)
print(pdf_content)
四、整合代码
将上述步骤整合到一个完整的Python脚本中,以实现从网页爬取PDF文件并读取其内容的功能。
import requests
from bs4 import BeautifulSoup
import PyPDF2
import os
def get_pdf_links(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
pdf_links = []
for link in soup.find_all('a', href=True):
href = link['href']
if href.endswith('.pdf'):
pdf_links.append(href)
return pdf_links
def download_pdfs(pdf_links, download_folder):
if not os.path.exists(download_folder):
os.makedirs(download_folder)
for link in pdf_links:
pdf_response = requests.get(link)
pdf_name = link.split('/')[-1]
with open(f'{download_folder}/{pdf_name}', 'wb') as pdf_file:
pdf_file.write(pdf_response.content)
print(f'Downloaded {pdf_name}')
def read_pdf(file_path):
with open(file_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
num_pages = reader.numPages
content = ''
for page_num in range(num_pages):
page = reader.getPage(page_num)
content += page.extract_text()
return content
url = 'http://example.com'
download_folder = 'pdfs'
pdf_links = get_pdf_links(url)
print(f'Found {len(pdf_links)} PDF links')
download_pdfs(pdf_links, download_folder)
for pdf_name in os.listdir(download_folder):
pdf_path = os.path.join(download_folder, pdf_name)
content = read_pdf(pdf_path)
print(f'Content of {pdf_name}:')
print(content)
五、处理复杂情况
在实际应用中,我们可能会遇到一些复杂情况,例如PDF链接的URL不是绝对路径、PDF内容需要进一步处理等。以下是一些处理这些情况的建议:
1、处理相对路径的PDF链接
有些网页中的PDF链接可能是相对路径,需要将其转换为绝对路径。
from urllib.parse import urljoin
def get_pdf_links(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
pdf_links = []
for link in soup.find_all('a', href=True):
href = link['href']
if href.endswith('.pdf'):
pdf_links.append(urljoin(url, href))
return pdf_links
2、处理PDF内容
对于某些PDF文件,PyPDF2
库可能无法正确提取文本内容。可以尝试使用其他库(如pdfminer
)来处理这些文件。
pip install pdfminer.six
from pdfminer.high_level import extract_text
def read_pdf_with_pdfminer(file_path):
content = extract_text(file_path)
return content
pdf_content = read_pdf_with_pdfminer(pdf_file_path)
print(pdf_content)
通过本文的介绍,您应该能够使用Python爬取PDF文件并读取其内容。根据实际需求,选择合适的工具和方法来处理复杂情况。希望本文对您有所帮助!
相关问答FAQs:
如何在Python中获取PDF文档的内容?
在Python中,可以使用多个库来提取PDF文档的内容,如PyPDF2、pdfplumber和PyMuPDF等。通过这些库,你可以读取PDF文件的文本、提取图像和处理PDF表单等。具体步骤包括安装库、打开PDF文件并使用相应的方法提取所需数据。
使用Python爬取PDF文件时需要注意哪些问题?
在爬取PDF文件时,需确保遵循网站的爬虫政策和版权法。很多网站在robots.txt文件中会规定哪些内容可以被爬取,确保遵守这些规定。此外,处理PDF文件的格式和编码可能会有所不同,因此在提取数据时要考虑到文本的准确性和完整性。
爬虫过程中如何处理PDF文件的下载和保存?
使用Python的requests库可以轻松下载PDF文件。下载后,可以使用os或shutil库将文件保存到指定的目录。确保在保存文件时使用合适的文件名和扩展名,以便后续的处理和访问。通过设置适当的请求头,可以避免因反爬虫机制而导致的下载失败。
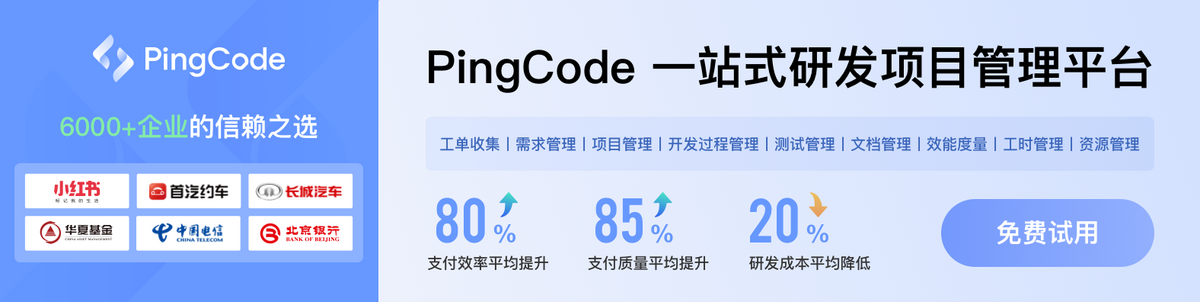